Creating a CRUD (Create, Read, Update, Delete) application in Java with a GUI can be quite comprehensive. Below is a detailed guide that covers everything from setting up your project to building the application with comments and explanations.
Project Setup
- IDE: Use an Integrated Development Environment (IDE) like IntelliJ IDEA, Eclipse, or NetBeans.
- JDK: Ensure you have the Java Development Kit (JDK) installed.
Step-by-Step Guide
1. Create a new Java project
Create a new Java project in your chosen IDE.
2. Create the Main Class
This is the entry point of your application.
package com.crudapp;
// Main class containing the entry point of the application
public class Main {
public static void main(String[] args) {
// Create an instance of AppFrame
AppFrame appFrame = new AppFrame();
// Make the frame visible
appFrame.setVisible(true);
}
}
Explanation:
package com.crudapp;
: Declares the package name.public class Main { ... }
: Defines the main class.public static void main(String[] args) { ... }
: The main method where the program starts.AppFrame appFrame = new AppFrame();
: Creates an instance of theAppFrame
class.appFrame.setVisible(true);
: Makes theAppFrame
visible.
3. Create the AppFrame Class
This class will set up the main frame of the GUI.
p the main frame of the GUI.
package com.crudapp;
import javax.swing.*;
// Class for the main application frame
public class AppFrame extends JFrame {
private JPanel panel;
private JLabel label;
private JTextField textField;
private JButton createButton;
private JButton readButton;
private JButton updateButton;
private JButton deleteButton;
private JTextArea textArea;
// Constructor to set up the frame
public AppFrame() {
// Set the title of the frame
setTitle("CRUD Application");
// Initialize the components
panel = new JPanel();
label = new JLabel("Enter data:");
textField = new JTextField(20);
createButton = new JButton("Create");
readButton = new JButton("Read");
updateButton = new JButton("Update");
deleteButton = new JButton("Delete");
textArea = new JTextArea(10, 30);
// Add components to the panel
panel.add(label);
panel.add(textField);
panel.add(createButton);
panel.add(readButton);
panel.add(updateButton);
panel.add(deleteButton);
panel.add(new JScrollPane(textArea));
// Add the panel to the frame
add(panel);
// Set the size of the frame
setSize(400, 300);
// Define what happens when the frame is closed
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Add action listeners for buttons
createButton.addActionListener(e -> createData());
readButton.addActionListener(e -> readData());
updateButton.addActionListener(e -> updateData());
deleteButton.addActionListener(e -> deleteData());
}
// Method to create data
private void createData() {
String data = textField.getText();
if (!data.isEmpty()) {
DataStore.create(data);
textArea.append("Created: " + data + "\n");
textField.setText("");
}
}
// Method to read data
private void readData() {
textArea.append("Current Data:\n");
for (String data : DataStore.read()) {
textArea.append(data + "\n");
}
}
// Method to update data
private void updateData() {
String data = textField.getText();
if (!data.isEmpty()) {
DataStore.update(data);
textArea.append("Updated to: " + data + "\n");
textField.setText("");
}
}
// Method to delete data
private void deleteData() {
String data = textField.getText();
if (!data.isEmpty()) {
DataStore.delete(data);
textArea.append("Deleted: " + data + "\n");
textField.setText("");
}
}
}
Explanation:
import javax.swing.*;
: Imports the Swing components for the GUI.public class AppFrame extends JFrame { ... }
: Defines theAppFrame
class that extendsJFrame
to create a window.public AppFrame() { ... }
: Constructor for initializing the frame.setTitle("CRUD Application");
: Sets the title of the frame.- Component Initialization: Initializes components like
JPanel
,JLabel
,JTextField
,JButton
, andJTextArea
. - Adding Components: Adds components to the panel and the panel to the frame.
setSize(400, 300);
: Sets the size of the frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
: Ensures the application exits when the frame is closed.- Action Listeners: Adds action listeners for button clicks, calling respective methods (
createData
,readData
,updateData
,deleteData
).
4. Create the DataStore Class
This class handles the data operations.
package com.crudapp;
import java.util.ArrayList;
import java.util.List;
// Class to handle CRUD operations on data
public class DataStore {
// Static list to store data
private static List<String> dataStore = new ArrayList<>();
// Method to create data
public static void create(String data) {
dataStore.add(data);
}
// Method to read data
public static List<String> read() {
return new ArrayList<>(dataStore);
}
// Method to update data (simple replace mechanism)
public static void update(String data) {
if (!dataStore.isEmpty()) {
dataStore.set(dataStore.size() - 1, data); // update the last item
}
}
// Method to delete data
public static void delete(String data) {
dataStore.remove(data);
}
}
Explanation:
import java.util.ArrayList;
andimport java.util.List;
: Imports theArrayList
andList
classes from the Java Collections Framework.public class DataStore { ... }
: Defines theDataStore
class for data operations.private static List<String> dataStore = new ArrayList<>();
: Declares a static list to store data.public static void create(String data) { ... }
: Adds data to the list.public static List<String> read() { ... }
: Returns a copy of the data list.public static void update(String data) { ... }
: Updates the last item in the list with the new data.public static void delete(String data) { ... }
: Removes the specified data from the list.
Running the Application
- Run the
Main
class. - You should see a GUI window where you can enter data and perform CRUD operations.
Usage:
- Create: Enter text in the text field and click “Create” to add data.
- Read: Click “Read” to display all current data.
- Update: Enter new text in the text field and click “Update” to replace the last added item.
- Delete: Enter the exact text of an item and click “Delete” to remove it.
This guide provides a simple CRUD application with a basic GUI and detailed explanations, making it easier for beginners to understand each step and component.
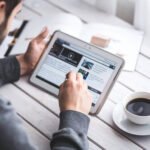
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.