Creating an inventory management application in C++ with a GUI and MySQL connectivity is feasible but involves a different set of libraries and tools. We will use the following:
- Qt: A powerful framework for creating cross-platform applications with a graphical user interface.
- MySQL Connector/C++: A MySQL client library for C++ that provides a C++ API for connecting to and interacting with MySQL databases.
Here’s a step-by-step guide to creating the application.
Prerequisites
- Install Qt (includes Qt Creator IDE): Qt Downloads
- Install MySQL Connector/C++: MySQL Connector/C++ Downloads
InventoryManagement
│ main.cpp
│ database.cpp
│ database.h
│ product.cpp
│ product.h
│ productdao.cpp
│ productdao.h
│ mainwindow.cpp
│ mainwindow.h
│ mainwindow.ui
└───include
qt_mysql_prj.pro
Step-by-Step Implementation
1. Setting up the Project
Create a new Qt Widgets Application in Qt Creator and set up the project structure as shown above.
2. database.h and database.cpp
These files handle the connection to the MySQL database.
database.h
#ifndef DATABASE_H
#define DATABASE_H
#include <mysql_driver.h>
#include <mysql_connection.h>
#include <cppconn/statement.h>
#include <cppconn/resultset.h>
#include <cppconn/prepared_statement.h>
class DatabaseConnection {
public:
static sql::Connection* getConnection();
};
#endif // DATABASE_H
Explanation:
DatabaseConnection
class provides a static method to establish and return a connection to the MySQL database.- It includes necessary MySQL Connector/C++ headers.
database.cpp
#include "database.h"
sql::Connection* DatabaseConnection::getConnection() {
sql::mysql::MySQL_Driver* driver = sql::mysql::get_mysql_driver_instance();
sql::Connection* conn = driver->connect("tcp://127.0.0.1:3306", "root", "password");
conn->setSchema("inventory");
return conn;
}
Explanation:
- The
getConnection
method establishes a connection to the MySQL database using the MySQL Connector/C++ API. - It connects to the MySQL server running on
127.0.0.1
(localhost) on port3306
, with the usernameroot
and passwordpassword
. - It selects the
inventory
schema/database. - It returns the connection object.
2. product.h
and product.cpp
These files define the Product
class representing a product entity.
product.h
#ifndef PRODUCT_H
#define PRODUCT_H
#include <string>
class Product {
public:
int id;
std::string name;
int quantity;
double price;
Product(int id, const std::string& name, int quantity, double price);
};
#endif // PRODUCT_H
Explanation:
Product
class models a product withid
,name
,quantity
, andprice
attributes.
product.cpp
#include "product.h"
Product::Product(int id, const std::string& name, int quantity, double price)
: id(id), name(name), quantity(quantity), price(price) {}
Explanation:
- The constructor initializes the attributes of the
Product
class.
3. productdao.h
and productdao.cpp
These files provide CRUD operations for the Product
class.
productdao.h
#ifndef PRODUCTDAO_H
#define PRODUCTDAO_H
#include "product.h"
#include <vector>
class ProductDAO {
public:
void addProduct(const Product& product);
std::vector<Product> getAllProducts();
void updateProduct(const Product& product);
void deleteProduct(int productId);
};
#endif // PRODUCTDAO_H
Explanation:
ProductDAO
class provides methods to add, retrieve, update, and delete products from the database.
productdao.cpp
#include "productdao.h"
#include "database.h"
void ProductDAO::addProduct(const Product& product) {
sql::Connection* conn = DatabaseConnection::getConnection();
sql::PreparedStatement* pstmt = conn->prepareStatement("INSERT INTO products (name, quantity, price) VALUES (?, ?, ?)");
pstmt->setString(1, product.name);
pstmt->setInt(2, product.quantity);
pstmt->setDouble(3, product.price);
pstmt->executeUpdate();
delete pstmt;
delete conn;
}
std::vector<Product> ProductDAO::getAllProducts() {
sql::Connection* conn = DatabaseConnection::getConnection();
sql::Statement* stmt = conn->createStatement();
sql::ResultSet* res = stmt->executeQuery("SELECT * FROM products");
std::vector<Product> products;
while (res->next()) {
products.emplace_back(res->getInt("id"), res->getString("name"), res->getInt("quantity"), res->getDouble("price"));
}
delete res;
delete stmt;
delete conn;
return products;
}
void ProductDAO::updateProduct(const Product& product) {
sql::Connection* conn = DatabaseConnection::getConnection();
sql::PreparedStatement* pstmt = conn->prepareStatement("UPDATE products SET name = ?, quantity = ?, price = ? WHERE id = ?");
pstmt->setString(1, product.name);
pstmt->setInt(2, product.quantity);
pstmt->setDouble(3, product.price);
pstmt->setInt(4, product.id);
pstmt->executeUpdate();
delete pstmt;
delete conn;
}
void ProductDAO::deleteProduct(int productId) {
sql::Connection* conn = DatabaseConnection::getConnection();
sql::PreparedStatement* pstmt = conn->prepareStatement("DELETE FROM products WHERE id = ?");
pstmt->setInt(1, productId);
pstmt->executeUpdate();
delete pstmt;
delete conn;
}
Explanation:
ProductDAO
class provides methods to add, retrieve, update, and delete products from the database.- Each method establishes a connection to the database, prepares an SQL statement, executes it, and handles the results.
4. mainwindow.ui
This file is created and edited using the Qt Designer. It defines the layout and components of the main window, including text fields, buttons, and a table view.
5. mainwindow.h
and mainwindow.cpp
These files define the main window’s logic and handle user interactions.
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QMessageBox>
#include "productdao.h"
#include <QStandardItemModel>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void on_addButton_clicked();
void on_updateButton_clicked();
void on_deleteButton_clicked();
void loadProducts();
private:
Ui::MainWindow *ui;
ProductDAO productDAO;
QStandardItemModel *model;
};
#endif // MAINWINDOW_H
Explanation:
MainWindow
class sets up the main window of the application and handles user interactions.- It includes slots for handling button clicks and a method for loading products into the table view.
mainwindow.cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include "product.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
, model(new QStandardItemModel(this)) {
ui->setupUi(this);
model->setHorizontalHeaderLabels({"ID", "Name", "Quantity", "Price"});
ui->tableView->setModel(model);
loadProducts();
}
MainWindow::~MainWindow() {
delete ui;
}
void MainWindow::loadProducts() {
model->removeRows(0, model->rowCount());
std::vector<Product> products = productDAO.getAllProducts();
for (const Product& product : products) {
QList<QStandardItem*> items;
items.append(new QStandardItem(QString::number(product.id)));
items.append(new QStandardItem(QString::fromStdString(product.name)));
items.append(new QStandardItem(QString::number(product.quantity)));
items.append(new QStandardItem(QString::number(product.price)));
model->appendRow(items);
}
}
void MainWindow::on_addButton_clicked() {
std::string name = ui->nameLineEdit->text().toStdString();
int quantity = ui->quantitySpinBox->value();
double price = ui->priceDoubleSpinBox->value();
Product product(0, name, quantity, price);
productDAO.addProduct(product);
loadProducts();
}
void MainWindow::on_updateButton_clicked() {
QModelIndexList selected = ui->tableView->selectionModel()->selectedRows();
if (!selected.isEmpty()) {
int id = selected[0].data().toInt();
std::string name = ui->nameLineEdit->text().toStdString();
int quantity = ui->quantitySpinBox->value();
double price = ui->priceDoubleSpinBox->value();
Product product(id, name, quantity, price);
productDAO.updateProduct(product);
loadProducts();
}
}
void MainWindow::on_deleteButton_clicked() {
QModelIndexList selected = ui->tableView->selectionModel()->selectedRows();
if (!selected.isEmpty()) {
int id = selected[0].data().toInt();
productDAO.deleteProduct(id);
loadProducts();
}
}
Explanation:
MainWindow
class sets up the main window of the application and handles user interactions.- The
loadProducts
method retrieves products from the database and populates the table view. - The
on_addButton_clicked
,on_updateButton_clicked
, andon_deleteButton_clicked
methods handle adding, updating, and deleting products respectively, and refresh the table view after each operation.
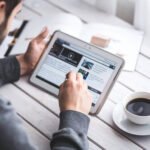
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.