In this project, we will build a simple portfolio website using React. This website will include an “About Me” section, a “Skills” section using CSS Grid, a “Projects” section, and a “Contact” section. We’ll focus on using Flexbox and CSS Grid for layout and making the design responsive.
Step 1: Setting Up Our Project with Vite
Explanation:
We will use Vite to set up our React project, as it’s fast and simple.
Instructions:
Open your terminal and type the following command to create a new React application using Vite:
npm create vite@latest portfolio-website -- --template react
- This command creates a new folder named
portfolio-website
and sets up a React project in it.
- This command creates a new folder named
Change to the project directory:
cd portfolio-website
Install the required dependencies:
npm install
Step 2: Cleaning Up the Project
Explanation:
The default files generated by Vite contain sample code that we don’t need. We’ll clean up these files and create our own structure.
Instructions:
- Inside the
src
folder, deleteApp.css
,App.jsx
, andindex.css
. - Create a new CSS file named
styles.css
inside thesrc
folder. This will contain all our CSS styles. - Create a new file named
App.jsx
in thesrc
folder, which will contain the structure of our portfolio website.
Step 3: Building the Basic Structure (HTML in React)
Explanation:
Now, we will set up the basic structure of our portfolio website. This will include:
- A header with navigation links.
- An “About Me” section.
- A “Skills” section using CSS Grid.
- A “Projects” section.
- A “Contact” section.
Instructions:
- Open
App.jsx
and add the following code
- Inside the
import React from 'react';
import './styles.css'; // Importing the CSS file
function App() {
return (
<div className="App">
{/* Header Section */}
<header className="header">
<h1 className="logo">My Portfolio</h1>
<nav className="navbar">
<ul className="nav-links">
<li><a href="#about">About</a></li>
<li><a href="#skills">Skills</a></li>
<li><a href="#projects">Projects</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
{/* About Section */}
<section id="about" className="about-section">
<h2>About Me</h2>
<p>Hello! I am a web developer with a passion for building beautiful and responsive websites.</p>
</section>
{/* Skills Section */}
<section id="skills" className="skills-section">
<h2>Skills</h2>
<div className="skills-grid">
<div className="skill">HTML</div>
<div className="skill">CSS</div>
<div className="skill">JavaScript</div>
<div className="skill">React</div>
<div className="skill">Node.js</div>
<div className="skill">Git</div>
</div>
</section>
{/* Projects Section */}
<section id="projects" className="projects-section">
<h2>Projects</h2>
<div className="projects-grid">
<div className="project-card">Project 1</div>
<div className="project-card">Project 2</div>
<div className="project-card">Project 3</div>
<div className="project-card">Project 4</div>
</div>
</section>
{/* Contact Section */}
<section id="contact" className="contact-section">
<h2>Contact</h2>
<p>Email: contact@example.com</p>
<p>Phone: +123 456 7890</p>
</section>
{/* Footer */}
<footer className="footer">
<p>© 2024 My Portfolio. All rights reserved.</p>
</footer>
</div>
);
}
export default App;
Explanation:
- We created different sections: About, Skills, Projects, and Contact.
- We used JSX syntax to define our components in the
App
function. - The
skills-grid
andprojects-grid
will use CSS Grid for layout.
Step 4: Styling the Page (CSS)
Explanation:
Now, let’s style the page using CSS. We’ll use Flexbox for the navigation and CSS Grid for the skills and projects sections. We’ll also make the layout responsive using media queries.
Instructions:
- Open
styles.css
and add the following styles
/* Reset default browser styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
line-height: 1.6;
}
.App {
display: flex;
flex-direction: column;
min-height: 100vh;
}
/* Header */
.header {
background-color: #333;
color: #fff;
padding: 10px 20px;
display: flex;
justify-content: space-between;
align-items: center;
}
.navbar .nav-links {
list-style: none;
display: flex;
}
.navbar .nav-links li {
margin-left: 20px;
}
.navbar .nav-links a {
color: #fff;
text-decoration: none;
}
/* About Section */
.about-section {
background-color: #f4f4f4;
padding: 50px 20px;
text-align: center;
}
/* Skills Section */
.skills-section {
background-color: #e4e4e4;
padding: 40px 20px;
text-align: center;
}
.skills-grid {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(100px, 1fr));
gap: 20px;
margin-top: 20px;
}
.skill {
background-color: #007bff;
color: #fff;
padding: 20px;
border-radius: 5px;
}
/* Projects Section */
.projects-section {
background-color: #f4f4f4;
padding: 40px 20px;
text-align: center;
}
.projects-grid {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(150px, 1fr));
gap: 20px;
margin-top: 20px;
}
.project-card {
background-color: #007bff;
color: #fff;
padding: 20px;
border-radius: 5px;
}
/* Contact Section */
.contact-section {
background-color: #e4e4e4;
padding: 40px 20px;
text-align: center;
}
/* Footer */
.footer {
background-color: #333;
color: #fff;
text-align: center;
padding: 20px 0;
margin-top: auto;
}
/* Responsive Styles */
@media (max-width: 768px) {
.navbar .nav-links {
flex-direction: column;
}
.navbar .nav-links li {
margin: 10px 0;
}
}
Explanation:
- Flexbox: Used to create a horizontal layout for the navigation in the header.
- CSS Grid: Used in the
.skills-grid
and.projects-grid
classes to create flexible grid layouts for the skills and projects sections. - Responsive Design: Added media queries to adjust the layout for smaller screens (e.g., stacking the navigation links vertically).
Step 5: Running the Application
Instructions:
- In the terminal, start the application by running
npm run dev
- Open your web browser and navigate to the URL shown in the terminal (usually
http://localhost:5173
) to see your portfolio website
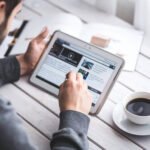
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.