Trading Analysis & Predictions
Creating a robust Event Management System (EMS) requires a well-structured backend that can efficiently handle various aspects like event creation, attendee management, venue management, budgeting, and more. In this first module, we’ll walk through the process of setting up the development environment. This includes installing necessary applications, setting up servers, managing dependencies, and connecting to a version control system (GitHub) using Visual Studio Code.
Module 1: Installation of Required Applications
Objective: This step focuses on setting up the essential tools and applications required to develop the backend of our Event Management System. By the end of this step, you will have installed Node.js, Visual Studio Code, Git, Postman, and optionally MongoDB, laying the groundwork for further development.
1.1 Install Node.js
Node.js is a JavaScript runtime that allows you to run JavaScript code on the server-side. It also includes npm (Node Package Manager), which is essential for managing project dependencies.
- Installation:
Visit the Node.js official website and download the latest stable version (LTS).
Follow the installation instructions specific to your operating system (Windows, macOS, or Linux).
Verify the installation by running the following commands in your terminal or command prompt:
node -v
npm -v
These commands should return the installed versions of Node.js and npm, confirming successful installation.
1.2 Install Visual Studio Code (VS Code)
Visual Studio Code is a powerful and versatile code editor with extensive support for extensions, making it ideal for JavaScript and Node.js development.
Installation:
- Download Visual Studio Code from the official website.
- Install it by following the instructions specific to your operating system.
- Once installed, open VS Code and familiarize yourself with the interface.
Extensions:
- To enhance your development experience, consider installing the following extensions:
- ESLint: For JavaScript and Node.js linting.
- Prettier: For code formatting.
- GitLens: For Git integration and visualization.
- DotENV: For managing environment variables.
- To enhance your development experience, consider installing the following extensions:
1.3 Install Git
Git is a version control system that allows you to track changes in your codebase and collaborate with others. It’s also essential for pushing your project to GitHub.
- Installation:
Download Git from the official website.
Follow the installation instructions for your operating system.
After installation, configure Git with your user information
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Verify the installation by running the following command
git --version
This command should return the installed version of Git, confirming successful installation.
1.4 Install Postman
Postman is a tool that allows you to test and interact with your API endpoints. It’s indispensable for verifying the functionality of your REST API during development.
- Installation:
- Download Postman from the official website.
- Install it by following the instructions for your operating system.
- Once installed, launch Postman and explore its interface, as it will be used extensively for API testing later on.
1.5 (Optional) Install MongoDB
MongoDB is the NoSQL database we’ll use to store our event management data. You can choose to install MongoDB locally or use a cloud-based service like MongoDB Atlas.
Local Installation:
Download MongoDB from the MongoDB official website.
Follow the installation instructions for your operating system.
After installation, verify that MongoDB is running by using the MongoDB shell:
mongod
Cloud Option – MongoDB Atlas:
- Sign up for a free MongoDB Atlas account at MongoDB Atlas.
- Create a new cluster, and get the connection string, which you’ll use later in your project’s
.env
file.
By completing Step 1, you have successfully installed and set up all the necessary tools and applications to start developing the Event Management System. These installations provide the foundation for building and managing the backend of the application in subsequent modules.
Module 1: Step 2 - Setting Up the Backend Server
Objective: In this step, we will set up the basic backend server using Node.js and Express. This involves initializing a Node.js project, installing essential dependencies, and creating a basic Express server that will act as the backbone for our Event Management System. We will also configure environment variables and set up a connection to MongoDB.
2.1 Initialize the Node.js Project
Create Project Directory:
- Open Visual Studio Code.
- Create a new directory for your project. You can do this directly in VS Code by navigating to
File > Open Folder
and selecting or creating a new folder.
Open Terminal:
- In VS Code, open a terminal by selecting
Terminal > New Terminal
.
- In VS Code, open a terminal by selecting
Initialize Node.js Project:
In the terminal, navigate to your project directory and initialize a new Node.js project with the following command:
npm init -y
This command creates a
package.json
file in your project directory. The-y
flag automatically accepts the default settings.
2.2 Install Essential Dependencies
- Install Express and Other Dependencies:
Run the following command in your terminal to install the necessary packages:
npm install express mongoose dotenv cors morgan
Express: A fast, unopinionated, minimalist web framework for Node.js.
Mongoose: An Object Data Modeling (ODM) library for MongoDB and Node.js, which makes it easier to work with MongoDB in a Node.js environment.
dotenv: A module that loads environment variables from a
.env
file intoprocess.env
, helping to manage sensitive information like database credentials.cors: Middleware to enable Cross-Origin Resource Sharing (CORS), which allows your API to handle requests from different domains.
morgan: A HTTP request logger middleware for Node.js that logs requests to the console, useful for debugging.
2.3 Set Up a Basic Express Server
Create
index.js
File:- In your project directory, create a new file named
index.js
. This will be the entry point of your Node.js application.
- In your project directory, create a new file named
Write Basic Server Code:
Open
index.js
in VS Code and add the following code:
const express = require('express');
const mongoose = require('mongoose');
const cors = require('cors');
const morgan = require('morgan');
const dotenv = require('dotenv');
dotenv.config();
const app = express();
// Middleware
app.use(cors());
app.use(morgan('dev'));
app.use(express.json());
// MongoDB Connection
mongoose.connect(process.env.MONGO_URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
})
.then(() => console.log('MongoDB connected'))
.catch(err => console.log(err));
// Basic route
app.get('/', (req, res) => {
res.send('Event Management API is running...');
});
// Server Listening
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Explanation:
- dotenv.config(): Loads environment variables from a
.env
file intoprocess.env
. - app.use(cors()): Enables CORS for all routes.
- app.use(morgan(‘dev’)): Logs HTTP requests to the console.
- app.use(express.json()): Parses incoming JSON requests and puts the parsed data in
req.body
. - mongoose.connect: Establishes a connection to MongoDB using the URI stored in the
.env
file. - app.get(‘/’): Defines a basic route that sends a simple response, indicating the server is running.
- dotenv.config(): Loads environment variables from a
Create
.env
File:In the root of your project directory, create a file named
.env
.Add the following lines to the
.env
file:
MONGO_URI=mongodb://localhost:27017/event_management
PORT=5000
Explanation:
- MONGO_URI: This is the connection string for your MongoDB database. If you’re using MongoDB Atlas, replace this with your Atlas connection string.
- PORT: The port number on which your server will listen. It’s set to
5000
by default.
Test the Server:
Start the server by running the following command in your terminal:
node index.js
If everything is set up correctly, you should see the following output in your terminal:
MongoDB connected
Server running on port 5000
Open your web browser and navigate to
http://localhost:5000/
. You should see the message “Event Management API is running…”.
2.4 Organize Project Structure (Optional at this Stage)
While not strictly necessary in the early stages, it’s good practice to start organizing your project structure for scalability:
Project Structure:
event-management-system/
├── node_modules/
├── .env
├── .gitignore
├── index.js
├── package.json
├── package-lock.json
└── README.md
- As the project grows, you can create additional folders like
controllers
,models
,routes
, andconfig
to organize your code better.
- As the project grows, you can create additional folders like
By completing Step 2, you’ve set up a basic Express server with a MongoDB connection, and your environment is configured to handle future development. This server will serve as the backbone for the entire Event Management System. With this foundation, you’re ready to proceed to more complex tasks like designing the database schema in the next module.
Module 2: Database Design
Objective: In this module, we’ll design the database for our Event Management System. This involves identifying entities, defining relationships, and creating a schema that reflects the system’s requirements. We’ll use MongoDB as our database, leveraging Mongoose for schema definitions.
2.1 Identify Entities and Relationships
Entities are the core components of the Event Management System. Each entity will correspond to a MongoDB collection, and relationships between entities will define how data is linked across collections.
Event:
- Attributes:
id
,name
,date
,time
,location
,description
,budgetId
,venueId
,attendeeIds
,speakerIds
- Relationships:
- One
Event
can have manyAttendees
. - One
Event
can have oneVenue
. - One
Event
can have manySpeakers
. - One
Event
can have oneBudget
.
- One
- Attributes:
Attendee:
- Attributes:
id
,name
,email
,phone
,eventId
- Relationships:
- Each
Attendee
is linked to oneEvent
.
- Each
- Attributes:
Venue:
- Attributes:
id
,name
,location
,capacity
,availability
- Relationships:
- Each
Venue
can host manyEvents
.
- Each
- Attributes:
Guest:
- Attributes:
id
,name
,email
,phone
,seatNumber
,eventId
- Relationships:
- Each
Guest
is linked to oneEvent
.
- Each
- Attributes:
Budget:
- Attributes:
id
,totalBudget
,expenses
,eventId
- Relationships:
- Each
Budget
is linked to oneEvent
.
- Each
- Attributes:
Speaker:
- Attributes:
id
,name
,topic
,timeSlot
,eventId
- Relationships:
- Each
Speaker
is linked to oneEvent
.
- Each
- Attributes:
Summary of Relationships:
- Event: The central entity.
- One-to-Many Relationships:
Event
→Attendees
Event
→Speakers
- One-to-One Relationships:
Event
→Budget
Event
→Venue
2.2 Create Mongoose Schemas
Event Schema:
const mongoose = require('mongoose');
const eventSchema = new mongoose.Schema({
name: { type: String, required: true },
date: { type: Date, required: true },
time: { type: String, required: true },
location: { type: String, required: true },
description: { type: String },
budgetId: { type: mongoose.Schema.Types.ObjectId, ref: 'Budget' },
venueId: { type: mongoose.Schema.Types.ObjectId, ref: 'Venue' },
attendeeIds: [{ type: mongoose.Schema.Types.ObjectId, ref: 'Attendee' }],
speakerIds: [{ type: mongoose.Schema.Types.ObjectId, ref: 'Speaker' }],
});
module.exports = mongoose.model('Event', eventSchema);
Attendee Schema:
const mongoose = require('mongoose');
const attendeeSchema = new mongoose.Schema({
name: { type: String, required: true },
email: { type: String, required: true },
phone: { type: String },
eventId: { type: mongoose.Schema.Types.ObjectId, ref: 'Event', required: true },
});
module.exports = mongoose.model('Attendee', attendeeSchema);
Venue Schema:
const mongoose = require('mongoose');
const venueSchema = new mongoose.Schema({
name: { type: String, required: true },
location: { type: String, required: true },
capacity: { type: Number, required: true },
availability: { type: Boolean, default: true },
});
module.exports = mongoose.model('Venue', venueSchema);
Guest Schema:
const mongoose = require('mongoose');
const guestSchema = new mongoose.Schema({
name: { type: String, required: true },
email: { type: String },
phone: { type: String },
seatNumber: { type: String },
eventId: { type: mongoose.Schema.Types.ObjectId, ref: 'Event', required: true },
});
module.exports = mongoose.model('Guest', guestSchema);
Budget Schema:
const mongoose = require('mongoose');
const budgetSchema = new mongoose.Schema({
totalBudget: { type: Number, required: true },
expenses: { type: Number, default: 0 },
eventId: { type: mongoose.Schema.Types.ObjectId, ref: 'Event', required: true },
});
module.exports = mongoose.model('Budget', budgetSchema);
Speaker Schema:
const mongoose = require('mongoose');
const speakerSchema = new mongoose.Schema({
name: { type: String, required: true },
topic: { type: String, required: true },
timeSlot: { type: String, required: true },
eventId: { type: mongoose.Schema.Types.ObjectId, ref: 'Event', required: true },
});
module.exports = mongoose.model('Speaker', speakerSchema);
2.3 Establish Database Relationships
Referencing Relationships:
- Use
ref
in Mongoose to reference other documents. For example, in theEvent
schema,attendeeIds
references theAttendee
model, which allows you to link multiple attendees to a single event. - This enables the use of Mongoose’s
populate()
method to retrieve linked documents.
- Use
Indexing for Performance:
Ensure frequently queried fields (like
eventId
in attendees, speakers, guests) are indexed for faster queries.Example:
attendeeSchema.index({ eventId: 1 });
2.4 Save and Test Schemas
Save Schema Files:
- Create a directory named
models
in your project and save each schema as a separate file (Event.js
,Attendee.js
, etc.).
- Create a directory named
Test Database Connection:
- In
index.js
, import and test one of your models to ensure everything is working correctly
- In
const Event = require('./models/Event');
app.get('/test', async (req, res) => {
const event = new Event({ name: 'Test Event', date: new Date(), time: '10:00 AM', location: 'Test Location' });
await event.save();
res.send(event);
});
- Start your server and visit
http://localhost:5000/test
to confirm that a new event is created in your MongoDB database.
- Start your server and visit
With Module 2 completed, your Event Management System now has a well-designed database schema that accurately reflects the relationships and entities involved in the application. This foundational work ensures that the subsequent modules—like user authentication, model creation, and route handling—are built on a solid and efficient data structure.
Module 3: User Authentication and Authorization
Objective: In this module, we will implement user registration, login, and authentication mechanisms for the Event Management System. We’ll use JSON Web Tokens (JWT) for secure authentication, bcrypt for password hashing, and middleware to protect routes. This module also covers error handling to ensure robust user management.
3.1 Install Additional Dependencies
Before implementing user authentication, we need to install some additional packages:
Install Required Packages:
npm install bcryptjs jsonwebtoken express-validator cookie-parser
- bcryptjs: For hashing and comparing passwords.
- jsonwebtoken: For generating and verifying JWTs.
- express-validator: For validating user input during registration and login.
- cookie-parser: For handling cookies, which can store JWTs.
3.2 Create User Model
We need a User
model to store user information, including hashed passwords.
Create
User.js
in themodels
Directory:
const mongoose = require('mongoose');
const bcrypt = require('bcryptjs');
const userSchema = new mongoose.Schema({
name: { type: String, required: true },
email: { type: String, required: true, unique: true },
password: { type: String, required: true },
role: { type: String, default: 'user' }, // 'user', 'admin', etc.
});
// Password hashing before saving the user
userSchema.pre('save', async function (next) {
if (!this.isModified('password')) {
return next();
}
const salt = await bcrypt.genSalt(10);
this.password = await bcrypt.hash(this.password, salt);
next();
});
// Password verification
userSchema.methods.matchPassword = async function (enteredPassword) {
return await bcrypt.compare(enteredPassword, this.password);
};
module.exports = mongoose.model('User', userSchema);
- Explanation:
userSchema.pre('save')
: Automatically hashes the password before saving the user document to the database.matchPassword
: A method to compare a given password with the hashed password stored in the database.
- Explanation:
3.3 Create Authentication Controllers
Create
authController.js
in a Newcontrollers
Directory:
const User = require('../models/User');
const jwt = require('jsonwebtoken');
const { validationResult } = require('express-validator');
// Generate JWT
const generateToken = (user) => {
return jwt.sign({ id: user._id, role: user.role }, process.env.JWT_SECRET, {
expiresIn: '1h',
});
};
// Register a new user
exports.register = async (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
const { name, email, password } = req.body;
try {
let user = await User.findOne({ email });
if (user) {
return res.status(400).json({ msg: 'User already exists' });
}
user = new User({ name, email, password });
await user.save();
const token = generateToken(user);
res.cookie('token', token, { httpOnly: true });
res.status(201).json({ token });
} catch (error) {
res.status(500).json({ msg: 'Server error' });
}
};
// Login user
exports.login = async (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
const { email, password } = req.body;
try {
const user = await User.findOne({ email });
if (!user) {
return res.status(400).json({ msg: 'Invalid credentials' });
}
const isMatch = await user.matchPassword(password);
if (!isMatch) {
return res.status(400).json({ msg: 'Invalid credentials' });
}
const token = generateToken(user);
res.cookie('token', token, { httpOnly: true });
res.json({ token });
} catch (error) {
res.status(500).json({ msg: 'Server error' });
}
};
// Logout user
exports.logout = (req, res) => {
res.cookie('token', '', { expires: new Date(0), httpOnly: true });
res.json({ msg: 'Logged out successfully' });
};
- Explanation:
- generateToken: Generates a JWT token with the user’s ID and role.
- register: Handles user registration, ensuring the email is unique and the password is hashed before saving the user.
- login: Validates user credentials and issues a JWT upon successful login.
- logout: Clears the JWT cookie to log the user out.
- Explanation:
3.4 Create Middleware for Authorization
Create
authMiddleware.js
in themiddleware
Directory
const jwt = require('jsonwebtoken');
const User = require('../models/User');
// Protect routes
exports.protect = async (req, res, next) => {
let token;
if (req.cookies.token) {
token = req.cookies.token;
} else if (
req.headers.authorization &&
req.headers.authorization.startsWith('Bearer')
) {
token = req.headers.authorization.split(' ')[1];
}
if (!token) {
return res.status(401).json({ msg: 'Not authorized, no token' });
}
try {
const decoded = jwt.verify(token, process.env.JWT_SECRET);
req.user = await User.findById(decoded.id).select('-password');
next();
} catch (error) {
return res.status(401).json({ msg: 'Not authorized, token failed' });
}
};
// Restrict routes by roles
exports.authorize = (...roles) => {
return (req, res, next) => {
if (!roles.includes(req.user.role)) {
return res.status(403).json({ msg: 'User role not authorized' });
}
next();
};
};
- Explanation:
- protect: Middleware that checks if the request has a valid JWT. If valid, the user’s details are attached to the
req
object. - authorize: Middleware that restricts access to specific roles (e.g., admin-only routes).
- protect: Middleware that checks if the request has a valid JWT. If valid, the user’s details are attached to the
- Explanation:
3.5 Create Routes
Create
authRoutes.js
in a Newroutes
Directory:
const express = require('express');
const { check } = require('express-validator');
const { register, login, logout } = require('../controllers/authController');
const router = express.Router();
// Register route
router.post(
'/register',
[
check('name', 'Name is required').not().isEmpty(),
check('email', 'Please include a valid email').isEmail(),
check('password', 'Password must be at least 6 characters').isLength({
min: 6,
}),
],
register
);
// Login route
router.post(
'/login',
[
check('email', 'Please include a valid email').isEmail(),
check('password', 'Password is required').exists(),
],
login
);
// Logout route
router.get('/logout', logout);
module.exports = router;
- Explanation:
- Routes are defined for registration, login, and logout. Validation is performed using
express-validator
before the controller logic is executed.
- Routes are defined for registration, login, and logout. Validation is performed using
- Explanation:
Integrate Routes in
index.js
:- Update
index.js
to use the authentication routes:
- Update
const authRoutes = require('./routes/authRoutes');
// Middleware for parsing cookies
const cookieParser = require('cookie-parser');
app.use(cookieParser());
// Use auth routes
app.use('/api/auth', authRoutes);
- Explanation:
- The routes are prefixed with
/api/auth
, meaning the full paths will be/api/auth/register
,/api/auth/login
, and/api/auth/logout
.
- The routes are prefixed with
- Explanation:
3.6 Error Handling
Create Global Error Handler:
- Add a global error handler to catch and respond to errors more gracefully:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).json({ msg: 'Server error' });
});
Refine Error Responses:
- Ensure that controllers and middleware send meaningful error messages, especially during validation and authentication failures.
3.7 Testing Authentication
- Test Using Postman:
Test the registration, login, and logout endpoints using Postman:
- Register: Send a POST request to
http://localhost:5000/api/auth/register
withname
,email
, andpassword
in the body. - Login: Send a POST request to
http://localhost:5000/api/auth/login
withemail
andpassword
. - Logout: Send a GET request to
http://localhost:5000/api/auth/logout
.
- Register: Send a POST request to
Check if JWTs are issued correctly, and test protected routes with and without valid tokens.
By completing Module 3, you have successfully implemented a robust user authentication and authorization system in the Event Management System. Users can now register, log in, and securely access protected resources. With JWT-based authentication in place, you can proceed with building out the core functionality of the system, knowing that your routes are securely protected.
Module 4: Models Creation
Objective: In this module, we’ll create the Mongoose models for our Event Management System. These models will define the structure of the data that will be stored in MongoDB. We’ve already started defining some models in the previous modules, such as the User
model. Now, we’ll create the remaining models and ensure they are all properly structured to support the application’s functionality.
4.1 Review and Finalize the Existing Models
Before creating new models, let’s review and finalize the models we already created in the previous modules:
User Model (
User.js
):- This model has already been created in Module 3 and includes fields for
name
,email
,password
, androle
. - Ensure that the password hashing and comparison methods are functioning as expected.
- This model has already been created in Module 3 and includes fields for
Event Model (
Event.js
):- We created the
Event
model in Module 2, defining attributes such asname
,date
,time
,location
,description
,budgetId
,venueId
,attendeeIds
, andspeakerIds
. - This model is the central entity that connects with other models like
Venue
,Attendee
,Speaker
, andBudget
.
- We created the
4.2 Create the Remaining Models
Now we’ll create the remaining models that were outlined in Module 2.
Attendee Model (
Attendee.js
):- This model stores information about the event attendees.
const mongoose = require('mongoose');
const attendeeSchema = new mongoose.Schema({
name: { type: String, required: true },
email: { type: String, required: true },
phone: { type: String },
eventId: { type: mongoose.Schema.Types.ObjectId, ref: 'Event', required: true },
});
module.exports = mongoose.model('Attendee', attendeeSchema);
- Explanation:
eventId
: This references theEvent
model, establishing a relationship between an attendee and an event.
- Explanation:
Venue Model (
Venue.js
):- This model stores information about the event venues.
const mongoose = require('mongoose');
const venueSchema = new mongoose.Schema({
name: { type: String, required: true },
location: { type: String, required: true },
capacity: { type: Number, required: true },
availability: { type: Boolean, default: true },
});
module.exports = mongoose.model('Venue', venueSchema);
- Explanation:
- The
Venue
model stores details likename
,location
,capacity
, andavailability
, which are crucial for event planning.
- The
- Explanation:
Guest Model (
Guest.js
):- This model manages information about the guests who attend the events
const mongoose = require('mongoose');
const guestSchema = new mongoose.Schema({
name: { type: String, required: true },
email: { type: String },
phone: { type: String },
seatNumber: { type: String },
eventId: { type: mongoose.Schema.Types.ObjectId, ref: 'Event', required: true },
});
module.exports = mongoose.model('Guest', guestSchema);
- Explanation:
- The
Guest
model allows us to track guest information, including seating arrangements.
- The
- Explanation:
Budget Model (
Budget.js
):- This model tracks the budgeting and expenses for each event
const mongoose = require('mongoose');
const budgetSchema = new mongoose.Schema({
totalBudget: { type: Number, required: true },
expenses: { type: Number, default: 0 },
eventId: { type: mongoose.Schema.Types.ObjectId, ref: 'Event', required: true },
});
module.exports = mongoose.model('Budget', budgetSchema);
- Explanation:
totalBudget
andexpenses
help manage the financial aspect of an event, ensuring that all costs are tracked.
- Explanation:
Speaker Model (
Speaker.js
):- This model manages information about the speakers at the events.
const mongoose = require('mongoose');
const speakerSchema = new mongoose.Schema({
name: { type: String, required: true },
topic: { type: String, required: true },
timeSlot: { type: String, required: true },
eventId: { type: mongoose.Schema.Types.ObjectId, ref: 'Event', required: true },
});
module.exports = mongoose.model('Speaker', speakerSchema);
- Explanation:
- The
Speaker
model includes information about the speakers, their topics, and the time slots they are assigned to during an event.
- The
- Explanation:
4.3 Integrate Models into the Application
Organize Models in the Project Structure:
- Ensure all models are placed in the
models
directory. - Your project structure should look something like this:
- Ensure all models are placed in the
event-management-system/
├── models/
│ ├── User.js
│ ├── Event.js
│ ├── Attendee.js
│ ├── Venue.js
│ ├── Guest.js
│ ├── Budget.js
│ └── Speaker.js
├── controllers/
├── routes/
├── middleware/
├── index.js
├── package.json
├── package-lock.json
├── .env
├── .gitignore
└── README.md
Import Models Where Needed:
- Import these models into your controllers or routes as needed. For example, in a controller where you need to access event attendees, you would import the
Attendee
model like so:
const Attendee = require('../models/Attendee');
Test the Models:
- You can write some test routes or scripts to ensure that the models are working as expected.
- For example, create a route to add a new event, assign a venue, and add attendees.
app.post('/api/events', async (req, res) => {
const { name, date, time, location, description } = req.body;
try {
const event = new Event({ name, date, time, location, description });
await event.save();
res.status(201).json(event);
} catch (error) {
res.status(500).json({ msg: 'Error creating event', error });
}
});
Handle Model Relationships:
- When creating new documents, ensure relationships are handled correctly. For example, when creating an
Attendee
, ensure you associate it with an existingEvent
by passing theeventId
.
- When creating new documents, ensure relationships are handled correctly. For example, when creating an
4.4 Testing and Validating the Models
Use Postman for API Testing:
- Test all CRUD operations (Create, Read, Update, Delete) for each model using Postman to ensure that the models interact correctly with MongoDB.
Validation and Error Handling:
- Ensure that all fields required by your schema have validation in place and that meaningful errors are returned when something goes wrong.
With Module 4 completed, you now have a fully fleshed-out set of models that accurately represent the key entities in your Event Management System. These models form the backbone of your application, enabling you to store, retrieve, and manipulate data related to events, attendees, venues, guests, budgets, and speakers. With the models in place, the next step will be to create controllers and routes that leverage these models to build out the functionality of your application.
Module 5: Controllers and Routes Creation
Objective: In this module, we will create the controllers and routes necessary to handle the core functionality of the Event Management System. The controllers will contain the logic for interacting with the models (created in the previous module), while the routes will define the API endpoints for accessing these controllers.
5.1 Create Controllers
Controllers are responsible for handling requests and returning responses. We’ll create controllers for the main entities: Event, Attendee, Venue, Guest, Budget, and Speaker.
Create Event Controller (
eventController.js
):
const Event = require('../models/Event');
// Create a new event
exports.createEvent = async (req, res) => {
try {
const event = new Event(req.body);
await event.save();
res.status(201).json(event);
} catch (error) {
res.status(500).json({ msg: 'Error creating event', error });
}
};
// Get all events
exports.getEvents = async (req, res) => {
try {
const events = await Event.find().populate('venueId speakerIds attendeeIds budgetId');
res.status(200).json(events);
} catch (error) {
res.status(500).json({ msg: 'Error fetching events', error });
}
};
// Get a single event by ID
exports.getEventById = async (req, res) => {
try {
const event = await Event.findById(req.params.id).populate('venueId speakerIds attendeeIds budgetId');
if (!event) {
return res.status(404).json({ msg: 'Event not found' });
}
res.status(200).json(event);
} catch (error) {
res.status(500).json({ msg: 'Error fetching event', error });
}
};
// Update an event
exports.updateEvent = async (req, res) => {
try {
const event = await Event.findByIdAndUpdate(req.params.id, req.body, { new: true });
if (!event) {
return res.status(404).json({ msg: 'Event not found' });
}
res.status(200).json(event);
} catch (error) {
res.status(500).json({ msg: 'Error updating event', error });
}
};
// Delete an event
exports.deleteEvent = async (req, res) => {
try {
const event = await Event.findByIdAndDelete(req.params.id);
if (!event) {
return res.status(404).json({ msg: 'Event not found' });
}
res.status(200).json({ msg: 'Event deleted successfully' });
} catch (error) {
res.status(500).json({ msg: 'Error deleting event', error });
}
};
- Explanation:
- createEvent: Handles the creation of a new event.
- getEvents: Fetches all events, including their related entities (venues, speakers, attendees, and budget).
- getEventById: Fetches a single event by its ID.
- updateEvent: Updates an existing event based on its ID.
- deleteEvent: Deletes an event by its ID.
- Explanation:
Create Attendee Controller (
attendeeController.js
):
const Attendee = require('../models/Attendee');
// Create a new attendee
exports.createAttendee = async (req, res) => {
try {
const attendee = new Attendee(req.body);
await attendee.save();
res.status(201).json(attendee);
} catch (error) {
res.status(500).json({ msg: 'Error creating attendee', error });
}
};
// Get all attendees
exports.getAttendees = async (req, res) => {
try {
const attendees = await Attendee.find().populate('eventId');
res.status(200).json(attendees);
} catch (error) {
res.status(500).json({ msg: 'Error fetching attendees', error });
}
};
// Get a single attendee by ID
exports.getAttendeeById = async (req, res) => {
try {
const attendee = await Attendee.findById(req.params.id).populate('eventId');
if (!attendee) {
return res.status(404).json({ msg: 'Attendee not found' });
}
res.status(200).json(attendee);
} catch (error) {
res.status(500).json({ msg: 'Error fetching attendee', error });
}
};
// Update an attendee
exports.updateAttendee = async (req, res) => {
try {
const attendee = await Attendee.findByIdAndUpdate(req.params.id, req.body, { new: true });
if (!attendee) {
return res.status(404).json({ msg: 'Attendee not found' });
}
res.status(200).json(attendee);
} catch (error) {
res.status(500).json({ msg: 'Error updating attendee', error });
}
};
// Delete an attendee
exports.deleteAttendee = async (req, res) => {
try {
const attendee = await Attendee.findByIdAndDelete(req.params.id);
if (!attendee) {
return res.status(404).json({ msg: 'Attendee not found' });
}
res.status(200).json({ msg: 'Attendee deleted successfully' });
} catch (error) {
res.status(500).json({ msg: 'Error deleting attendee', error });
}
};
- Explanation:
- createAttendee: Handles the creation of a new attendee.
- getAttendees: Fetches all attendees, including their associated event.
- getAttendeeById: Fetches a single attendee by ID.
- updateAttendee: Updates an attendee based on their ID.
- deleteAttendee: Deletes an attendee by ID.
- Explanation:
Create Similar Controllers for Venue, Guest, Budget, and Speaker:
- Follow the same pattern as above to create controllers for
Venue
,Guest
,Budget
, andSpeaker
. - Each controller should include methods for creating, reading (all and single by ID), updating, and deleting the respective entities.
- Follow the same pattern as above to create controllers for
5.2 Create Routes
With the controllers in place, we can now create routes that will map HTTP requests to these controller functions.
Create Event Routes (
eventRoutes.js
):
const express = require('express');
const { protect, authorize } = require('../middleware/authMiddleware');
const {
createEvent,
getEvents,
getEventById,
updateEvent,
deleteEvent,
} = require('../controllers/eventController');
const router = express.Router();
// Public routes
router.route('/').get(getEvents);
// Protected routes
router.use(protect);
router.route('/').post(authorize('admin'), createEvent);
router
.route('/:id')
.get(getEventById)
.put(authorize('admin'), updateEvent)
.delete(authorize('admin'), deleteEvent);
module.exports = router;
- Explanation:
- getEvents is a public route accessible to anyone.
- createEvent, updateEvent, and deleteEvent are protected and require the user to be authenticated and authorized (e.g.,
admin
role).
- Explanation:
Create Attendee Routes (
attendeeRoutes.js
):
const express = require('express');
const { protect } = require('../middleware/authMiddleware');
const {
createAttendee,
getAttendees,
getAttendeeById,
updateAttendee,
deleteAttendee,
} = require('../controllers/attendeeController');
const router = express.Router();
// Protected routes
router.use(protect);
router.route('/').get(getAttendees).post(createAttendee);
router
.route('/:id')
.get(getAttendeeById)
.put(updateAttendee)
.delete(deleteAttendee);
module.exports = router;
- Explanation:
- All routes in this file are protected, meaning only authenticated users can access them.
- Explanation:
Create Similar Routes for Venue, Guest, Budget, and Speaker:
- Create route files for
Venue
,Guest
,Budget
, andSpeaker
following the patterns above.
- Create route files for
5.3 Integrate Routes into the Application
Update
index.js
to Include Routes:
const eventRoutes = require('./routes/eventRoutes');
const attendeeRoutes = require('./routes/attendeeRoutes');
const venueRoutes = require('./routes/venueRoutes');
const guestRoutes = require('./routes/guestRoutes');
const budgetRoutes = require('./routes/budgetRoutes');
const speakerRoutes = require('./routes/speakerRoutes');
app.use('/api/events', eventRoutes);
app.use('/api/attendees', attendeeRoutes);
app.use('/api/venues', venueRoutes);
app.use('/api/guests', guestRoutes);
app.use('/api/budgets', budgetRoutes);
app.use('/api/speakers', speakerRoutes);
- Explanation:
- This integrates all the routes into the main application, making them accessible via
/api/events
,/api/attendees
, etc.
- This integrates all the routes into the main application, making them accessible via
- Explanation:
5.4 Testing the Controllers and Routes
Use Postman for Testing:
- Test each endpoint using Postman. Ensure that all CRUD operations are functioning correctly and that route protections (authentication and authorization) are enforced where applicable.
Check Error Handling:
- Make sure to test how the application responds to invalid data, missing fields, and unauthorized access. Proper error messages should be returned for each case.
By completing Module 5, you have successfully created the controllers and routes that handle the core functionality of the Event Management System. These components allow the system to manage events, attendees, venues, guests, budgets, and speakers, while ensuring that access is controlled through authentication and authorization mechanisms. With this module completed, the core of your application is now functional, and you can proceed to adding advanced features like pagination, filtering, and searching in the next module.
Module 6: Advanced Pagination, Filtering, and Search
Objective: In this module, we will implement advanced features such as pagination, filtering, and search functionality for the Event Management System. These features will enhance the usability of the API by allowing clients to retrieve data in a more efficient and targeted manner.
6.1 Implement Pagination
Pagination helps in managing large datasets by splitting them into smaller, manageable chunks. This also improves the performance of API responses.
Create Pagination Middleware (
paginate.js
):- Create a middleware that can be applied to any route that returns a list of items.
const paginate = (model) => {
return async (req, res, next) => {
const page = parseInt(req.query.page) || 1;
const limit = parseInt(req.query.limit) || 10;
const skip = (page - 1) * limit;
try {
const total = await model.countDocuments();
const pages = Math.ceil(total / limit);
const results = await model.find().skip(skip).limit(limit);
res.paginatedResults = {
page,
limit,
total,
pages,
results,
};
next();
} catch (error) {
res.status(500).json({ msg: 'Error during pagination', error });
}
};
};
module.exports = paginate;
- Explanation:
- The middleware calculates the
skip
andlimit
values based on thepage
andlimit
query parameters. - It attaches the paginated results to
res.paginatedResults
, which can be accessed in the route handler.
- The middleware calculates the
- Explanation:
Apply Pagination to Routes:
- Modify a route (e.g., events) to include pagination.
const express = require('express');
const { getEvents } = require('../controllers/eventController');
const paginate = require('../middleware/paginate');
const Event = require('../models/Event');
const router = express.Router();
router.route('/').get(paginate(Event), (req, res) => {
res.status(200).json(res.paginatedResults);
});
module.exports = router;
- Explanation:
- The
paginate(Event)
middleware is applied to the GET/api/events
route, and the paginated results are returned in the response.
- The
- Explanation:
6.2 Implement Filtering
Filtering allows users to retrieve data based on specific criteria, making it easier to find relevant information.
Add Filtering Logic to Controllers:
- Modify the
getEvents
controller to include filtering based on certain fields (e.g.,name
,date
,location
).
- Modify the
exports.getEvents = async (req, res) => {
try {
const query = {};
if (req.query.name) {
query.name = { $regex: req.query.name, $options: 'i' };
}
if (req.query.location) {
query.location = { $regex: req.query.location, $options: 'i' };
}
if (req.query.date) {
query.date = req.query.date;
}
const events = await Event.find(query).populate('venueId speakerIds attendeeIds budgetId');
res.status(200).json(events);
} catch (error) {
res.status(500).json({ msg: 'Error fetching events', error });
}
};
- Explanation:
- This logic checks for query parameters (
name
,location
,date
) and filters the results accordingly using MongoDB’s$regex
for partial matches and case-insensitive searches.
- This logic checks for query parameters (
- Explanation:
Apply Filtering to Other Controllers:
- Add similar filtering logic to the controllers for
Attendees
,Venues
,Guests
,Budgets
, andSpeakers
as needed. - Example for
Attendees
:
- Add similar filtering logic to the controllers for
exports.getAttendees = async (req, res) => {
try {
const query = {};
if (req.query.name) {
query.name = { $regex: req.query.name, $options: 'i' };
}
if (req.query.email) {
query.email = { $regex: req.query.email, $options: 'i' };
}
const attendees = await Attendee.find(query).populate('eventId');
res.status(200).json(attendees);
} catch (error) {
res.status(500).json({ msg: 'Error fetching attendees', error });
}
};
- Explanation:
- This example allows filtering attendees by
name
andemail
.
- This example allows filtering attendees by
- Explanation:
6.3 Implement Search Functionality
Search functionality allows users to find resources based on more general criteria, often applied across multiple fields.
Add a Global Search Method:
- Modify the
getEvents
controller to include a search option that checks multiple fields.
- Modify the
exports.getEvents = async (req, res) => {
try {
const query = {};
if (req.query.search) {
query.$or = [
{ name: { $regex: req.query.search, $options: 'i' } },
{ location: { $regex: req.query.search, $options: 'i' } },
{ description: { $regex: req.query.search, $options: 'i' } },
];
}
const events = await Event.find(query).populate('venueId speakerIds attendeeIds budgetId');
res.status(200).json(events);
} catch (error) {
res.status(500).json({ msg: 'Error fetching events', error });
}
};
- Explanation:
- The
search
query parameter allows searching across multiple fields (name
,location
,description
) using MongoDB’s$or
operator.
- The
- Explanation:
Apply Search to Other Controllers:
- Implement similar search functionality in other controllers (
Attendees
,Venues
,Guests
, etc.) as required.
- Implement similar search functionality in other controllers (
6.4 Combine Pagination, Filtering, and Search
Integrate All Features:
- Combine pagination, filtering, and search in a single controller. Here’s how you can integrate all these features in the
getEvents
controller:
- Combine pagination, filtering, and search in a single controller. Here’s how you can integrate all these features in the
const paginate = require('../middleware/paginate');
const Event = require('../models/Event');
exports.getEvents = async (req, res) => {
const query = {};
if (req.query.name) {
query.name = { $regex: req.query.name, $options: 'i' };
}
if (req.query.location) {
query.location = { $regex: req.query.location, $options: 'i' };
}
if (req.query.date) {
query.date = req.query.date;
}
if (req.query.search) {
query.$or = [
{ name: { $regex: req.query.search, $options: 'i' } },
{ location: { $regex: req.query.search, $options: 'i' } },
{ description: { $regex: req.query.search, $options: 'i' } },
];
}
try {
const paginatedResults = await Event.find(query)
.populate('venueId speakerIds attendeeIds budgetId')
.skip(res.paginatedResults.skip)
.limit(res.paginatedResults.limit);
res.status(200).json({
page: res.paginatedResults.page,
limit: res.paginatedResults.limit,
total: res.paginatedResults.total,
pages: res.paginatedResults.pages,
results: paginatedResults,
});
} catch (error) {
res.status(500).json({ msg: 'Error fetching events', error });
}
};
- Explanation:
- This example shows how pagination, filtering, and search can all be integrated into a single API endpoint.
- Explanation:
Test the Combined Functionality:
- Use Postman to test the combined functionality:
- Test different combinations of
page
,limit
, filtering fields, and search queries. - Ensure that the responses are paginated, filtered, and searchable as expected.
- Test different combinations of
- Use Postman to test the combined functionality:
By completing Module 6, you have successfully implemented advanced pagination, filtering, and search features for the Event Management System. These enhancements make the API more flexible and user-friendly, allowing clients to retrieve data more efficiently based on specific criteria. With these features in place, the system is well-equipped to handle large datasets and provide users with the tools they need to find the information they’re looking for. In the next module, we will focus on testing and deploying the application.
Module 7: Testing and Deployment
Objective: In this final module, we will focus on thoroughly testing the Event Management System to ensure it functions correctly and reliably. After testing, we’ll deploy the application to a production environment, making it accessible to users.
7.1 Testing the Application
Testing is critical to ensure the system functions as expected. We’ll perform both unit and integration testing.
Install Testing Libraries
- Install testing libraries like
Jest
andSupertest
for writing and running tests
- Install testing libraries like
npm install --save-dev jest supertest
- Jest: A JavaScript testing framework used for unit testing.
- Supertest: An HTTP assertions library used for testing API endpoints.
Setup Jest
- Add a script in
package.json
to run tests using Jest.
- Add a script in
"scripts": {
"test": "jest"
}
Write Unit Tests
Create a
tests
directory in the root of your project.Example Test for Event Controller (
tests/eventController.test.js
):
const request = require('supertest');
const app = require('../index'); // Assuming index.js is where your Express app is defined
const mongoose = require('mongoose');
const Event = require('../models/Event');
describe('Event API', () => {
beforeAll(async () => {
await Event.deleteMany({});
});
afterAll(async () => {
await mongoose.connection.close();
});
it('should create a new event', async () => {
const res = await request(app)
.post('/api/events')
.send({
name: 'Test Event',
date: '2024-08-30',
time: '10:00 AM',
location: 'Test Location',
});
expect(res.statusCode).toEqual(201);
expect(res.body).toHaveProperty('_id');
expect(res.body.name).toBe('Test Event');
});
it('should fetch all events', async () => {
const res = await request(app).get('/api/events');
expect(res.statusCode).toEqual(200);
expect(res.body.results.length).toBeGreaterThan(0);
});
});
- Explanation:
- The tests cover creating a new event and fetching all events.
beforeAll
andafterAll
hooks manage the test environment, ensuring the database is in a known state.
- Explanation:
Run Tests
- Run the tests using Jest.
npm test
- Review the output to ensure all tests pass.
Write Additional Tests
- Write similar tests for other controllers (
Attendee
,Venue
,Guest
,Budget
,Speaker
). - Include edge cases and error conditions (e.g., invalid data, unauthorized access).
- Write similar tests for other controllers (
7.2 Deployment
Once testing is complete and all tests pass, the application is ready to be deployed.
Choose a Hosting Provider
- Popular hosting options include:
- Heroku: Easy-to-use platform with a free tier for small projects.
- AWS (Amazon Web Services): Provides more control and scalability.
- DigitalOcean: Simple and cost-effective cloud hosting.
- Vercel or Netlify: Primarily for frontend, but can be used for backend deployments as well.
For this guide, we’ll focus on deploying to Heroku.
- Popular hosting options include:
Prepare the Application for Deployment
- Ensure the
PORT
environment variable is correctly handled inindex.js
.
- Ensure the
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Ensure all environment variables (e.g.,
MONGO_URI
,JWT_SECRET
) are properly set up.Create a
Procfile
in the root directory, which tells Heroku how to run your application:
web: node index.js
Deploy to Heroku
Login to Heroku:
heroku login
Create a New Heroku App:
heroku create event-management-system
Set Environment Variables:
heroku config:set MONGO_URI=<your_mongo_uri>
heroku config:set JWT_SECRET=<your_jwt_secret>
Deploy the Application:
git push heroku master
Monitor the Deployment:
- Use
heroku logs --tail
to monitor the logs and ensure the application starts correctly.
- Use
Test the Deployed Application
After deployment, use tools like Postman to test the API endpoints in the production environment.
Ensure that all functionality works as expected in the live environment.
Set Up Monitoring and Alerts
Use Heroku’s built-in monitoring tools or integrate with third-party services like New Relic or Datadog to monitor the health and performance of your application.
Set up alerts for critical issues (e.g., downtime, high response times).
Backup and Scalability
- Database Backup: Regularly back up your MongoDB database using tools like MongoDB Atlas‘s built-in backup service or Mongodump.
- Scalability: Plan for scaling your application based on user growth. Use Heroku’s scaling features or consider moving to more scalable platforms like AWS or Google Cloud as needed.
By completing Module 7, you have thoroughly tested and deployed your Event Management System. This final step ensures that your application is reliable, secure, and accessible to users. With the application live, you can continue to monitor its performance, gather feedback, and iterate on the system to add new features or improve existing functionality. Congratulations on completing the development of your Event Management System!
EXTRA OPTIONAL FUNCTIONS
Step 8.1.1: Monitoring and Logging
Objective: Set up monitoring and logging to ensure the application runs smoothly in production, and to quickly identify and fix any issues that arise.
8.1.1.1 Setting Up Monitoring
Choose a Monitoring Service:
- Popular monitoring services include New Relic, Datadog, and Heroku Metrics. Since we’ve deployed to Heroku, we’ll start with Heroku Metrics and then discuss how to integrate with New Relic.
Heroku Metrics:
- Enable Heroku Metrics:
- Heroku automatically provides some basic metrics (such as memory usage, response time, etc.) on their dashboard. Navigate to your Heroku app dashboard, and under the “Metrics” tab, you’ll see real-time monitoring.
- You can also add Papertrail for more advanced logging, which we’ll cover later.
- Set Up Alerts:
- In the Heroku dashboard, you can set up alerts for when certain thresholds are met, such as high memory usage or slow response times.
- Enable Heroku Metrics:
New Relic Integration:
- Add New Relic to Heroku:
- Go to your Heroku app dashboard, navigate to the “Resources” tab, and search for “New Relic APM” in the “Add-ons” search box.
- Click “Provision” to add New Relic to your app.
- Set Up New Relic:
- After provisioning, you’ll receive a New Relic license key. You’ll need to add this key to your Heroku environment variables:
- Add New Relic to Heroku:
heroku config:set NEW_RELIC_LICENSE_KEY=your_license_key
Install the New Relic agent in your Node.js application:
npm install newrelic
Create a newrelic.js
file in the root directory of your project:
'use strict';
/**
* New Relic agent configuration.
*
* See lib/config/default.js in the agent distribution for a more complete
* description of configuration variables and their potential values.
*/
exports.config = {
app_name: ['Event Management System'],
license_key: process.env.NEW_RELIC_LICENSE_KEY,
logging: {
level: 'info',
},
};
At the very top of your index.js
file (before any other code), require New Relic
require('newrelic');
Deploy your changes to Heroku
git add .
git commit -m "Add New Relic monitoring"
git push heroku master
- Visit the New Relic dashboard to view detailed performance metrics, error tracking, and more.
8.1.1.2 Setting Up Logging
Use Papertrail for Log Management:
- Add Papertrail to Heroku:
- On your Heroku dashboard, go to the “Resources” tab and search for “Papertrail” in the “Add-ons” search box.
- Click “Provision” to add Papertrail to your app.
- Set Up Logging:
- Papertrail automatically captures logs from your Heroku app. You can access these logs via the Papertrail dashboard linked from your Heroku app’s resources page.
- Log Format Customization:
- To enhance the logs, you can customize how logs are recorded in your Node.js app. You can do this using a logging library like
winston
:
- To enhance the logs, you can customize how logs are recorded in your Node.js app. You can do this using a logging library like
- Add Papertrail to Heroku:
npm install winston
Create a logger.js
file in your project:
const { createLogger, format, transports } = require('winston');
const { combine, timestamp, printf } = format;
const logFormat = printf(({ level, message, timestamp }) => {
return `${timestamp} [${level}]: ${message}`;
});
const logger = createLogger({
format: combine(timestamp(), logFormat),
transports: [new transports.Console()],
});
module.exports = logger;
Replace console.log
statements in your code with the logger
const logger = require('./logger');
logger.info('Server is running on port 5000');
logger.error('Error connecting to MongoDB');
Set Up Alerts in Papertrail:
- In the Papertrail dashboard, set up alerts for specific log patterns, such as error messages or warnings, so you can be notified immediately via email or Slack.
Test Your Logging Setup:
- Deploy your application and generate some logs by interacting with your API.
- Ensure that the logs appear in the Papertrail dashboard and that alerts are working as expected.
By following these steps, you’ve successfully set up monitoring with Heroku Metrics and New Relic, and you’ve configured log management using Papertrail. These tools will help you keep track of your application’s health, diagnose issues quickly, and ensure a smooth user experience.
Would you like to proceed with the next part of Step 8.1, or is there anything specific you’d like to focus on next?
Step 8.1.2: Regular Backups and Security Updates
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Objective: In this part of the post-deployment maintenance, we’ll focus on setting up regular backups for your database and ensuring your application is secure by regularly updating your dependencies and managing access controls.
8.1.2.1 Setting Up Regular Database Backups
Automated Backups with MongoDB Atlas (if using MongoDB Atlas):
- Enable Backups:
- MongoDB Atlas provides built-in automated backups. If you are using Atlas, navigate to your cluster in the MongoDB Atlas dashboard.
- Go to the “Backups” tab and ensure that backups are enabled. Atlas automatically takes snapshots based on the backup policy you select.
- Configure Backup Frequency:
- You can configure the frequency of backups (e.g., daily, weekly) and retention policies (e.g., how long to keep backups).
- Set up alerts for backup success or failure notifications to ensure you are always informed about the backup status.
- Enable Backups:
Manual Backups Using Mongodump (if using a local MongoDB instance):
- Install Mongodump:
mongodump
is a utility that comes with the MongoDB database tools package. It’s used to create a backup of your MongoDB database.- If it’s not already installed, download and install it from the MongoDB Download Center.
- Create a Backup Script:
Write a simple shell script to back up your database:
- Install Mongodump:
#!/bin/bash
TIMESTAMP=$(date +"%F")
BACKUP_DIR="/path_to_backup_directory/$TIMESTAMP"
MONGO_DATABASE="your_database_name"
MONGO_URI="mongodb://username:password@localhost:27017/$MONGO_DATABASE"
mkdir -p "$BACKUP_DIR"
mongodump --uri="$MONGO_URI" --out="$BACKUP_DIR"
Save this script as
backup.sh
.
- Automate Backups Using Cron (Linux/Unix-based systems):
- Schedule the backup script to run automatically using cron
crontab -e
Add a cron job to run the backup script daily at midnight
0 0 * * * /path_to_your_script/backup.sh >> /var/log/mongobackup.log 2>&1
Store Backups Securely:
- Consider copying your backups to a secure offsite location, such as an AWS S3 bucket, using tools like
awscli
:
aws s3 cp /path_to_backup_directory/ s3://your_s3_bucket/ --recursive
8.1.2.2 Managing Security Updates
Regularly Update Dependencies:
Run npm Audit:
npm audit
helps you identify vulnerabilities in your project’s dependencies.- Run the following command regularly to check for vulnerabilities
npm audit
Review the audit report and update any packages that have vulnerabilities
npm audit fix
Check for Outdated Packages:
- Use the
npm outdated
command to see which packages are outdated
npm outdated
Update outdated packages with
npm update
For major version updates, review the changelog for breaking changes:
npm install <package>@latest
Use Snyk for Continuous Security Monitoring:
- Integrate Snyk:
- Snyk is a tool that continuously monitors your application for vulnerabilities and provides alerts.
- You can sign up for a free account at Snyk.
- Integrate Snyk with your GitHub repository or directly in your CI/CD pipeline:
npm install -g snyk
snyk auth
snyk test
- Set up Snyk to monitor your project continuously and automatically open pull requests with fixes when vulnerabilities are found.
Manage Access Controls:
- Review and Update Access Permissions:
- Regularly review who has access to your production environment, including your Heroku dashboard, MongoDB database, and any other critical systems.
- Use role-based access control (RBAC) where possible, ensuring that users only have access to the resources they need.
- Use Two-Factor Authentication (2FA):
- Enable 2FA on all critical accounts, including GitHub, Heroku, MongoDB Atlas, and any other services you use.
- Review and Update Access Permissions:
Test Security:
- Perform Penetration Testing:
- If your application handles sensitive data, consider performing penetration testing to identify and fix potential security weaknesses.
- You can either hire a professional service or use open-source tools like OWASP ZAP.
- Review Logs Regularly:
- Regularly review your Papertrail logs for suspicious activity, such as unauthorized access attempts or unexpected errors
- Perform Penetration Testing:
By completing these steps, you’ve ensured that your database is regularly backed up, your dependencies are up-to-date and secure, and your application is protected by strong access controls. These measures will help you maintain the integrity and security of your Event Management System over time.
Would you like to continue with the next step, such as gathering user feedback and iterating on the application, or is there another specific area you’d like to focus on?
Step 8.3: Expanding the Application
Objective: In this step, we’ll focus on expanding the functionality of your Event Management System by adding new features. These features will enhance the application’s capabilities and provide additional value to users.
8.3.1 Adding New Features
Let’s explore a few potential features you can add to your application:
Real-Time Notifications
Objective: Implement real-time notifications to keep users informed about important updates related to their events, such as new attendee registrations, event updates, or schedule changes.
Implementation Steps:
Set Up Socket.io:
- Install Socket.io:
npm install socket.io
Integrate Socket.io with Express:
- In your
index.js
, set up Socket.io
const http = require('http');
const socketio = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = socketio(server);
io.on('connection', (socket) => {
console.log('New WebSocket connection');
socket.on('disconnect', () => {
console.log('User has disconnected');
});
});
server.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
- Replace
app.listen
withserver.listen
to ensure both HTTP and WebSocket traffic are handled.
- Replace
Emit Notifications from the Server:
- Modify your controllers to emit events via Socket.io whenever a significant action occurs (e.g., an attendee registers)
const io = require('./index').io; // Assuming io is exported from index.js
exports.createAttendee = async (req, res) => {
try {
const attendee = new Attendee(req.body);
await attendee.save();
// Emit an event when a new attendee registers
io.emit('newAttendee', { event: req.body.eventId, attendee });
res.status(201).json(attendee);
} catch (error) {
res.status(500).json({ msg: 'Error creating attendee', error });
}
};
Handle Notifications on the Client Side (if applicable):
- If you have a frontend or plan to add one, you can handle these events on the client side
const socket = io();
socket.on('newAttendee', (data) => {
console.log(`New attendee registered for event ${data.event}`);
// Update the UI or show a notification
});
Event Analytics Dashboard
Objective: Create an analytics dashboard that allows event organizers to view key metrics about their events, such as attendance rates, budget adherence, and engagement levels.
Implementation Steps:
Create a Dashboard Route and Controller:
- Set up a new route for the dashboard
const express = require('express');
const { getDashboardData } = require('../controllers/dashboardController');
const router = express.Router();
router.get('/:eventId', getDashboardData);
module.exports = router;
In the dashboardController.js
const Event = require('../models/Event');
const Attendee = require('../models/Attendee');
const Budget = require('../models/Budget');
exports.getDashboardData = async (req, res) => {
try {
const eventId = req.params.eventId;
const event = await Event.findById(eventId);
const attendees = await Attendee.find({ eventId });
const budget = await Budget.findOne({ eventId });
const attendanceRate = (attendees.length / event.capacity) * 100;
const budgetUsed = (budget.expenses / budget.totalBudget) * 100;
res.status(200).json({
eventName: event.name,
attendanceRate: attendanceRate.toFixed(2),
budgetUsed: budgetUsed.toFixed(2),
totalAttendees: attendees.length,
});
} catch (error) {
res.status(500).json({ msg: 'Error fetching dashboard data', error });
}
};
Frontend Integration (Optional):
- If you have a frontend, you can create a visual dashboard using a charting library like Chart.js or D3.js to display the analytics data.
Payment Integration
Objective: Integrate a payment gateway (e.g., Stripe) to handle event ticket sales, deposits, or donations directly within the application.
Implementation Steps:
Set Up Stripe:
- Install Stripe SDK
npm install stripe
Set Up Stripe API Key:
- Add your Stripe API key to the
.env
file
STRIPE_SECRET_KEY=your_stripe_secret_key
Create a Payment Route and Controller:
const express = require('express');
const stripe = require('stripe')(process.env.STRIPE_SECRET_KEY);
const router = express.Router();
router.post('/checkout', async (req, res) => {
const { amount, currency, description } = req.body;
try {
const paymentIntent = await stripe.paymentIntents.create({
amount,
currency,
description,
payment_method_types: ['card'],
});
res.status(201).json({ clientSecret: paymentIntent.client_secret });
} catch (error) {
res.status(500).json({ msg: 'Error creating payment intent', error });
}
});
module.exports = router;
Handle Payment on the Client Side (if applicable):
- On the frontend, use Stripe’s client-side library to handle the payment:
const stripe = Stripe('your_publishable_key');
const result = await stripe.confirmCardPayment(clientSecret, {
payment_method: {
card: cardElement, // Assuming you use Stripe's CardElement
billing_details: {
name: 'Cardholder Name',
},
},
});
if (result.error) {
console.log('Payment failed:', result.error.message);
} else {
console.log('Payment succeeded:', result.paymentIntent);
}
Testing Payments:
- Use Stripe’s test mode to simulate payments with test card numbers. Ensure the payment workflow is smooth and handles errors appropriately.
By adding features like real-time notifications, an event analytics dashboard, and payment integration, your Event Management System becomes significantly more powerful and user-friendly. These enhancements can provide substantial value to users, making the application more versatile and capable of handling complex event management needs.
Would you like to proceed with implementing one of these features, or is there another specific area you’d like to focus on next?
Step 8.4: Scaling the Application
Objective: In this step, we’ll focus on scaling your Event Management System to handle increased user load and ensure the application remains performant and available as it grows.
8.4.1 Database Scaling
Sharding with MongoDB
Objective: Implement database sharding to distribute your data across multiple servers (shards), improving performance and scalability.
Implementation Steps:
Understand Sharding Concepts:
- Shards: These are individual MongoDB instances that store a subset of your data.
- Config Servers: These store metadata and configuration settings for the sharded cluster.
- Query Routers (Mongos): These interface with client applications and route queries to the appropriate shard(s).
Set Up a Sharded Cluster (MongoDB Atlas or Self-Managed):
MongoDB Atlas:
- If using MongoDB Atlas, you can easily enable sharding through the Atlas UI by upgrading your cluster to a sharded cluster.
- Atlas handles the configuration and management of shards, making it simpler to scale your database.
Self-Managed MongoDB:
- If you’re managing MongoDB on your servers, you need to manually set up sharding:
- Deploy and configure config servers.
- Deploy multiple shards (replica sets recommended).
- Deploy mongos instances to route queries.
- Add Shards to the cluster:
- If you’re managing MongoDB on your servers, you need to manually set up sharding:
sh.addShard("shard1/hostname1:port,hostname2:port,hostname3:port")
sh.addShard("shard2/hostname4:port,hostname5:port,hostname6:port")
Enable Sharding on your database and collections
sh.enableSharding("your_database")
sh.shardCollection("your_database.your_collection", { shardKey: 1 })
- Shard Key Selection: Choose an appropriate shard key. This key determines how the data is distributed across shards. A good shard key ensures even distribution and minimizes cross-shard queries.
Monitor and Adjust Sharding Configuration:
- Use MongoDB’s built-in tools to monitor the performance of your sharded cluster and adjust the shard key or add/remove shards as needed.
- MongoDB Compass or Atlas Metrics can help visualize how data is distributed and how queries are being executed across shards.
Implement Caching
Objective: Reduce the load on your database by implementing caching, which stores frequently accessed data in memory.
Implementation Steps:
Use Redis for Caching:
- Install Redis:
- If you don’t already have Redis installed, you can install it locally or use a managed Redis service like Redis Labs or AWS ElastiCache.
- Integrate Redis with Node.js:
- Install the Redis client for Node.js
- Install Redis:
npm install redis
Connect to Redis in your application
const redis = require('redis');
const client = redis.createClient();
client.on('connect', () => {
console.log('Connected to Redis');
});
client.on('error', (err) => {
console.log('Redis error: ', err);
});
Cache API Responses:
- Cache the results of expensive database queries. For example, when fetching events:
exports.getEventById = async (req, res) => {
const eventId = req.params.id;
client.get(eventId, async (err, event) => {
if (event) {
return res.status(200).json(JSON.parse(event));
} else {
try {
const event = await Event.findById(eventId).populate('venueId speakerIds attendeeIds budgetId');
client.setex(eventId, 3600, JSON.stringify(event)); // Cache for 1 hour
return res.status(200).json(event);
} catch (error) {
res.status(500).json({ msg: 'Error fetching event', error });
}
}
});
};
Invalidate Cache:
- When an event is updated or deleted, invalidate the cache
exports.updateEvent = async (req, res) => {
const eventId = req.params.id;
try {
const event = await Event.findByIdAndUpdate(eventId, req.body, { new: true });
client.del(eventId); // Invalidate cache
res.status(200).json(event);
} catch (error) {
res.status(500).json({ msg: 'Error updating event', error });
}
};
Implement HTTP Caching:
- Set Cache-Control Headers:
- Use
Cache-Control
headers in your API responses to instruct browsers or proxies to cache certain responses:
- Use
res.set('Cache-Control', 'public, max-age=3600'); // Cache for 1 hour
Use ETag for Conditional Requests:
- Implement ETags to allow clients to cache responses and revalidate them when necessary:
res.set('ETag', someHashOfResponseBody);
if (req.headers['if-none-match'] === someHashOfResponseBody) {
return res.status(304).end(); // Not Modified
}
8.4.2 Load Balancing and Auto-Scaling
Load Balancing
Objective: Distribute incoming traffic across multiple instances of your application to improve availability and performance.
Implementation Steps:
Use Heroku’s Built-in Load Balancer:
- Add More Dynos:
- Heroku automatically load balances across multiple dynos (instances). Increase the number of dynos to handle more traffic:
- Add More Dynos:
heroku ps:scale web=2
- You can scale horizontally by increasing the number of dynos based on traffic.
Use AWS Elastic Load Balancing (For AWS Deployments):
- Create an Elastic Load Balancer in the AWS Management Console and configure it to distribute traffic across multiple EC2 instances running your Node.js application.
- Attach Target Groups:
- Define a target group (a group of instances that receive traffic) and attach it to the load balancer.
- Set Up Health Checks:
- Configure health checks to monitor the health of your instances and ensure that only healthy instances receive traffic.
Auto-Scaling
Objective: Automatically adjust the number of running instances based on traffic to ensure your application can handle varying loads without manual intervention.
Implementation Steps:
Enable Heroku Auto-Scaling:
- Heroku has an auto-scaling feature available for certain dyno types. You can enable auto-scaling from the Heroku dashboard under the “Resources” tab.
- Configure the scaling rules based on response time or throughput, and Heroku will automatically adjust the number of dynos.
Auto-Scaling with AWS (For AWS Deployments):
- Set Up Auto-Scaling Groups:
- Create an Auto Scaling group in the AWS Management Console that defines the minimum and maximum number of EC2 instances.
- Configure Scaling Policies:
- Set up scaling policies that trigger when certain conditions are met, such as CPU usage exceeding a certain threshold.
- AWS will automatically launch or terminate instances based on these policies.
- Set Up Auto-Scaling Groups:
Monitor Scaling Activities:
- Regularly monitor your application’s performance and the effectiveness of your load balancing and auto-scaling configurations.
- Use CloudWatch (AWS) or Heroku Metrics to track scaling activities and adjust your configurations as necessary.
By implementing these scaling strategies, your Event Management System will be better equipped to handle increased traffic and ensure consistent performance, even as your user base grows. Sharding your database, implementing caching, and setting up load balancing and auto-scaling will provide the foundation for a robust, scalable application.
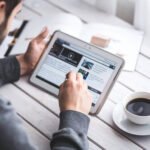
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.