The focus will be on creating a clean, readable article with sections, headers, images, and supporting content. Here’s how we can approach it:
Step 1: HTML Structure
We’ll create an article that includes a header with navigation, a title, an introductory paragraph, several sections with headings and content, an image, and a conclusion. Finally, there will be a footer with navigation and social links.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Webpage Example</title>
<!-- Link to Font Awesome for icons -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0-beta3/css/all.min.css">
<link rel="stylesheet" href="styles.css">
</head>
<body>
<!-- Navbar Section -->
<header class="header">
<div class="container">
<div class="logo">
<h1>Shortly</h1>
</div>
<nav class="nav">
<ul>
<li><a href="#">Features</a></li>
<li><a href="#">Pricing</a></li>
<li><a href="#">Resources</a></li>
</ul>
<div class="auth-buttons">
<button class="login">Login</button>
<button class="signup">Sign Up</button>
</div>
</nav>
</div>
</header>
<!-- Hero Section -->
<section class="hero">
<div class="container">
<div class="hero-content">
<h2>More than just shorter links</h2>
<p>Build your brand's recognition and get detailed insights on how your links are performing.</p>
<button class="cta-button">Get Started</button>
</div>
<div class="hero-image">
<img src="https://source.unsplash.com/collection/190727/600x400" alt="Illustration">
</div>
</div>
</section>
<!-- Search/Link Shortening Section -->
<section class="shorten">
<div class="container">
<div class="shorten-box">
<input type="text" placeholder="Shorten a link here...">
<button class="shorten-button">Shorten It!</button>
</div>
</div>
</section>
<!-- Advanced Statistics Section -->
<section class="statistics">
<div class="container">
<h2>Advanced Statistics</h2>
<p>Track how your links are performing across the web with our advanced statistics dashboard.</p>
<div class="statistics-cards">
<div class="card">
<div class="card-icon"><i class="fas fa-chart-line"></i></div>
<h3>Brand Recognition</h3>
<p>Boost your brand recognition with each click. Generic links don't mean a thing. Branded links help instill confidence in your content.</p>
</div>
<div class="card">
<div class="card-icon"><i class="fas fa-clipboard-list"></i></div>
<h3>Detailed Records</h3>
<p>Gain insights into who is clicking your links. Knowing when and where people engage with your content helps inform better decisions.</p>
</div>
<div class="card">
<div class="card-icon"><i class="fas fa-cogs"></i></div>
<h3>Fully Customizable</h3>
<p>Improve brand awareness and content discoverability through customizable links, supercharging audience engagement.</p>
</div>
</div>
</div>
</section>
<!-- Footer Section -->
<footer class="footer">
<div class="container">
<div class="footer-logo">
<h2>Shortly</h2>
</div>
<div class="footer-links">
<div>
<h4>Features</h4>
<ul>
<li><a href="#">Link Shortening</a></li>
<li><a href="#">Branded Links</a></li>
<li><a href="#">Analytics</a></li>
</ul>
</div>
<div>
<h4>Resources</h4>
<ul>
<li><a href="#">Blog</a></li>
<li><a href="#">Developers</a></li>
<li><a href="#">Support</a></li>
</ul>
</div>
<div>
<h4>Company</h4>
<ul>
<li><a href="#">About</a></li>
<li><a href="#">Our Team</a></li>
<li><a href="#">Careers</a></li>
<li><a href="#">Contact</a></li>
</ul>
</div>
</div>
<div class="footer-social">
<a href="#"><i class="fab fa-facebook-f"></i></a>
<a href="#"><i class="fab fa-twitter"></i></a>
<a href="#"><i class="fab fa-pinterest"></i></a>
<a href="#"><i class="fab fa-instagram"></i></a>
</div>
</div>
</footer>
</body>
</html>
Step 2: CSS Styling
We’ll use modern CSS techniques to style the page, ensuring that it’s responsive and visually appealing.
/* Basic Reset */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body {
background-color: #f0f1f6;
color: #333;
}
.container {
width: 85%;
max-width: 1200px;
margin: 0 auto;
}
/* Header Styles */
.header {
background-color: #3b3054;
color: white;
padding: 20px 0;
display: flex;
justify-content: space-between;
align-items: center;
}
.header .logo h1 {
font-size: 1.8rem;
margin-left: 20px;
}
.header .nav {
display: flex;
justify-content: space-between;
align-items: center;
flex-grow: 1;
}
.header .nav ul {
list-style: none;
display: flex;
gap: 20px;
margin-right: auto;
margin-left: 40px;
}
.header .nav a {
color: white;
text-decoration: none;
font-weight: 500;
}
.auth-buttons {
display: flex;
gap: 10px;
margin-right: 20px;
}
.auth-buttons button {
background-color: transparent;
border: 1px solid white;
padding: 10px 20px;
border-radius: 25px;
color: white;
cursor: pointer;
font-weight: bold;
}
.auth-buttons .signup {
background-color: #2acfcf;
border: none;
}
/* Hero Section */
.hero {
display: flex;
align-items: center;
justify-content: space-between;
padding: 50px 0;
}
.hero-content h2 {
font-size: 2.5rem;
color: #34313d;
}
.hero-content p {
margin: 20px 0;
color: #bfbfbf;
font-size: 1.1rem;
}
.cta-button {
padding: 15px 30px;
background-color: #2acfcf;
border: none;
border-radius: 25px;
color: white;
font-weight: bold;
cursor: pointer;
}
.hero-image img {
max-width: 100%;
}
/* Shorten Section */
.shorten {
background-color: #3b3054;
padding: 30px 0;
position: relative;
}
.shorten-box {
display: flex;
justify-content: space-between;
background-color: white;
padding: 15px;
border-radius: 10px;
box-shadow: 0 5px 10px rgba(0,0,0,0.1);
}
.shorten-box input {
border: none;
outline: none;
padding: 15px;
flex: 1;
font-size: 1rem;
border-radius: 5px;
}
.shorten-button {
padding: 15px 30px;
background-color: #2acfcf;
border: none;
border-radius: 5px;
color: white;
font-weight: bold;
cursor: pointer;
}
/* Statistics Section */
.statistics {
padding: 50px 0;
text-align: center;
}
.statistics h2 {
font-size: 2rem;
color: #34313d;
}
.statistics p {
margin: 20px 0;
color: #bfbfbf;
font-size: 1.1rem;
}
.statistics-cards {
display: flex;
justify-content: space-between;
gap: 20px;
margin-top: 30px;
}
.card {
background-color: white;
padding: 30px;
border-radius: 10px;
box-shadow: 0 5px 15px rgba(0,0,0,0.1);
text-align: left;
flex: 1;
}
.card-icon {
font-size: 2rem;
color: #2acfcf;
margin-bottom: 20px;
}
.card h3 {
font-size: 1.5rem;
color: #34313d;
}
.card p {
color: #bfbfbf;
font-size: 1rem;
}
/* Footer Styles */
.footer {
background-color: #232127;
color: white;
padding: 50px 0;
display: flex;
justify-content: space-between;
flex-wrap: wrap;
align-items: center;
}
.footer h2 {
font-size: 1.8rem;
margin-bottom: 20px;
}
.footer .footer-logo {
flex-basis: 100%;
text-align: center;
}
.footer .footer-links {
display: flex;
justify-content: space-between;
gap: 40px;
flex-grow: 1;
flex-wrap: wrap;
margin: 20px 0;
}
.footer .footer-links h4 {
font-size: 1.2rem;
margin-bottom: 15px;
}
.footer .footer-links ul {
list-style: none;
}
.footer .footer-links a {
color: white;
text-decoration: none;
font-size: 1rem;
}
.footer .footer-social {
display: flex;
gap: 15px;
}
.footer .footer-social a {
color: white;
font-size: 1.5rem;
}
/* Responsive Design */
@media (max-width: 768px) {
.hero {
flex-direction: column;
text-align: center;
}
.statistics-cards {
flex-direction: column;
}
.footer {
text-align: center;
flex-direction: column;
}
.footer .footer-links {
flex-direction: column;
gap: 20px;
margin: 20px 0;
}
}
Explanation of the Layout:
Header with Navigation:
- A clean, centered header with a logo and navigation links.
Main Article Content:
- Structured with several sections including an introduction, core content sections, and a conclusion.
- The article includes headings, paragraphs, and an image to make it engaging and easy to read.
Footer:
- Contains quick links and social media icons, styled to match the overall theme.
Responsive Design:
- The layout adapts for smaller screens, ensuring that the content remains accessible and visually appealing on mobile devices.
Step 3: Viewing the Webpage
- Save the HTML code as
index.html
. - Save the CSS code as
styles.css
in the same directory. - Open the
index.html
file in your browser to see the article-style webpage.
This structure should give you a clean, well-organized article-style webpage that is easy to read and navigate, while also being responsive to different screen sizes.
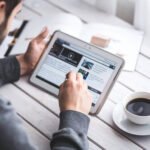
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.