In this article, we will outline the process of building a backend blog application using Node.js, MongoDB, and Express, following the MVC (Model-View-Controller) architectural pattern. This guide will cover everything from initial setup and installation to implementing CRUD operations, search, filtering, pagination, error handling, and finally documenting and deploying the application. We will not focus on the frontend, as our primary goal is to create a robust backend system.
Module 1: Setup and Installation
Step 1: Install Node.js and MongoDB First, ensure that Node.js and MongoDB are installed on your machine. Node.js can be downloaded from the official website, and MongoDB can be installed either locally or using a cloud service like MongoDB Atlas.
Step 2: Initialize the Project Create a new directory for your project and initialize a new Node.js project using the command npm init -y
. This will generate a package.json
file to manage your project’s dependencies.
Step 3: Install Dependencies Install essential dependencies such as Express for creating the server, Mongoose for interacting with MongoDB, and other utilities like body-parser and dotenv for environment variable management. Additionally, install nodemon as a development dependency to auto-restart the server on file changes.
Step 4: Setup Directory Structure Organize your project with a clear directory structure to separate concerns, such as models, controllers, routes, middlewares, and configuration files.
Module 2: Application Configuration
Environment Variables Create a .env
file to manage environment variables, including the MongoDB URI and the server port.
Database Configuration Set up a database configuration file to handle the connection to MongoDB using Mongoose. This file will be responsible for establishing and managing the database connection.
App Initialization In your main application file, initialize Express, connect to MongoDB, and set up middleware such as body-parser to parse incoming request bodies.
Module 3: MVC Structure
Model Define a single model for the blog posts in a separate file. This model will include the schema for the blog posts, which consists of a title and description.
Controller Create a controller file to handle the CRUD operations (Create, Read, Update, Delete) for the blog posts. Each function in the controller will correspond to a specific operation, such as creating a new blog post or retrieving all blog posts.
Routes Define the API endpoints in a routes file. These routes will map HTTP requests to the appropriate controller functions.
Module 4: Features Implementation
CRUD Operations Implement the basic CRUD operations in the controller and set up the corresponding routes. This includes creating, reading, updating, and deleting blog posts.
Search and Filtering Enhance the read functionality by adding search and filtering capabilities. This allows users to search for blog posts by title or filter posts based on certain criteria.
Pagination Implement pagination to manage large sets of blog posts. This involves returning a subset of posts for each request, along with metadata about the total number of posts and the current page.
Module 5: Error Handling and Validation
Error Handling Middleware Create a global error handling middleware to manage and respond to errors consistently across the application.
Request Validation Use a validation library to validate incoming requests, ensuring that the data meets the required criteria before processing it.
Module 6: Documentation and GitHub Integration
API Documentation Document your API endpoints, including the request parameters, response format, and any error messages. This can be done using tools like Swagger or simply by writing a detailed README file.
GitHub Integration Initialize a Git repository and push your project to GitHub. Use Visual Studio Code to manage your repository and track changes.
Module 7: Testing and Deployment
Testing Use tools like Postman to test your API endpoints and ensure they work as expected.
Deployment Deploy your application to a cloud platform such as Heroku, AWS, or DigitalOcean. Ensure that environment variables are properly configured in the deployment environment.
Module 8: Final Documentation
README.md Create a comprehensive README file to document the project setup, installation steps, usage instructions, and any other relevant information.
By following these modules, you can build a robust backend blog application using Node.js, MongoDB, and Express. This modular approach ensures that the development process is organized and manageable, leading to a well-structured and maintainable codebase.
Module 1: Setup and installation
In this module, we will focus on setting up the basic environment and installing the necessary tools and dependencies for our backend blog application.
Step 1: Install Node.js and MongoDB
Node.js Installation:
- Download and install Node.js from the official Node.js website.
- Verify the installation by running
node -v
andnpm -v
in your terminal to check the installed versions of Node.js and npm.
MongoDB Installation:
- Install MongoDB locally by downloading it from the official MongoDB website or use MongoDB Atlas, a cloud-based MongoDB service.
- If you are using MongoDB locally, ensure the MongoDB server is running. You can start it by running
mongod
in your terminal.
Step 2: Initialize the Project
Create a Project Directory:
- Open your terminal and create a new directory for your project. Navigate into this directory:
mkdir blog-backend
cd blog-backend
Initialize a Node.js Project:
- Initialize a new Node.js project with default settings
npm init -y
- This command will create a
package.json
file in your project directory.
Step 3: Install Dependencies
Install Essential Packages:
- Install Express for building the server, Mongoose for interacting with MongoDB, body-parser for parsing incoming request bodies, and dotenv for managing environment variables:bash
npm install express mongoose body-parser dotenv
Install Development Dependencies:
- Install nodemon as a development dependency to automatically restart the server when file changes are detected:
npm install --save-dev nodemon
Step 4: Setup Directory Structure
Organize Project Files:
- Create the following directory structure to separate concerns and maintain a clean codebase:
mkdir -p config controllers middlewares models routes
touch .env app.js
Directory Structure:
blog-backend/
├── config/
├── controllers/
├── middlewares/
├── models/
├── routes/
├── .env
├── app.js
└── package.json
Environment Variables:
- Create a
.env
file in the root of your project to store environment variables such as the MongoDB URI and server port. This file should look like this:
PORT=5000
MONGODB_URI=mongodb://localhost:27017/blogdb
Nodemon Configuration:
- Add a script in the
package.json
file to use nodemon for development
"scripts": {
"start": "node app.js",
"dev": "nodemon app.js"
}
Module 2 Application Configuration
In this module, we will configure our application by setting up environment variables, connecting to the MongoDB database, and initializing the Express app with the necessary middleware.
Environment Variables
Step 1: Create a .env
File
- The
.env
file should already exist from Module 1. Ensure it contains the following variables:
PORT=5000
MONGODB_URI=mongodb://localhost:27017/blogdb
Step 2: Load Environment Variables
- Install the
dotenv
package if you haven’t already
npm install dotenv
In your app.js
file, load the environment variables at the beginning
require('dotenv').config();
Database Configuration
Step 1: Create Database Configuration File
- Create a
config/database.js
file to handle the MongoDB connection using Mongoose:
// config/database.js
const mongoose = require('mongoose');
const connectDB = async () => {
try {
await mongoose.connect(process.env.MONGODB_URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
console.log('MongoDB connected');
} catch (err) {
console.error(err.message);
process.exit(1);
}
};
module.exports = connectDB;
Step 2: Connect to the Database
- Import and call the
connectDB
function in yourapp.js
file to establish a database connection
// app.js
const express = require('express');
const bodyParser = require('body-parser');
const connectDB = require('./config/database');
// Load environment variables
require('dotenv').config();
const app = express();
// Connect to database
connectDB();
// Middleware
app.use(bodyParser.json());
// Define routes (to be added later)
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
App Initialization
Step 1: Initialize Express
- Initialize Express in the
app.js
file, set up middleware, and start the server
// app.js
const express = require('express');
const bodyParser = require('body-parser');
const connectDB = require('./config/database');
// Load environment variables
require('dotenv').config();
const app = express();
// Connect to database
connectDB();
// Middleware
app.use(bodyParser.json());
// Define routes (to be added later)
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Step 2: Middleware Setup
- Use
body-parser
to parse JSON request bodies
app.use(bodyParser.json());
Module 3: MVC Structure
In this module, we will set up the Model-View-Controller (MVC) structure for our blog application. The MVC architecture helps in organizing the code in a structured manner, separating the data (model), the user interface (view), and the control logic (controller).
Model
Step 1: Define the Blog Model
- Create a
models/blog.js
file to define the schema for the blog posts. This schema will include thetitle
anddescription
fields, both of which are required.
// models/blog.js
const mongoose = require('mongoose');
const blogSchema = new mongoose.Schema({
title: {
type: String,
required: true,
},
description: {
type: String,
required: true,
},
}, { timestamps: true });
module.exports = mongoose.model('Blog', blogSchema);
Step 2: Create Blog Controller
Handle CRUD Operations
Create a controllers/blogController.js
file to handle the logic for creating, reading, updating, and deleting blog posts. Additionally, we’ll implement search, filtering, and pagination.
// controllers/blogController.js
const Blog = require('../models/blog');
// Create a new blog post
exports.createBlog = async (req, res) => {
try {
const { title, description } = req.body;
const newBlog = new Blog({ title, description });
await newBlog.save();
res.status(201).json(newBlog);
} catch (err) {
res.status(500).json({ message: err.message });
}
};
// Get all blog posts with pagination, search, and filtering
exports.getBlogs = async (req, res) => {
try {
const { page = 1, limit = 10, search = '' } = req.query;
const query = search ? { title: { $regex: search, $options: 'i' } } : {};
// Find blogs based on query, limit results, and skip pages
const blogs = await Blog.find(query)
.limit(limit * 1) // Convert limit to number and limit results
.skip((page - 1) * limit) // Skip results to get the correct page
.exec();
// Count total documents that match the query
const count = await Blog.countDocuments(query);
// Respond with blogs, total pages, and current page
res.json({
blogs,
totalPages: Math.ceil(count / limit), // Calculate total pages
currentPage: page, // Current page
});
} catch (err) {
res.status(500).json({ message: err.message });
}
};
// Get a single blog post by ID
exports.getBlogById = async (req, res) => {
try {
const blog = await Blog.findById(req.params.id);
if (!blog) return res.status(404).json({ message: 'Blog not found' });
res.json(blog);
} catch (err) {
res.status(500).json({ message: err.message });
}
};
// Update a blog post by ID
exports.updateBlog = async (req, res) => {
try {
const { title, description } = req.body;
const blog = await Blog.findByIdAndUpdate(
req.params.id,
{ title, description },
{ new: true } // Return the updated document
);
if (!blog) return res.status(404).json({ message: 'Blog not found' });
res.json(blog);
} catch (err) {
res.status(500).json({ message: err.message });
}
};
// Delete a blog post by ID
exports.deleteBlog = async (req, res) => {
try {
const blog = await Blog.findByIdAndDelete(req.params.id);
if (!blog) return res.status(404).json({ message: 'Blog not found' });
res.json({ message: 'Blog deleted' });
} catch (err) {
res.status(500).json({ message: err.message });
}
};
Detailed Explanation of controllers/blogController.js
Let’s go through the code in controllers/blogController.js
step by step, providing detailed explanations for each part.
Importing the Blog Model
const Blog = require('../models/blog');
This line imports the Blog
model, which we defined in the models/blog.js
file. The Blog
model represents the schema for our blog posts and allows us to interact with the MongoDB database using Mongoose.
Create a New Blog Post
exports.createBlog = async (req, res) => {
try {
const { title, description } = req.body; // Destructure title and description from the request body
const newBlog = new Blog({ title, description }); // Create a new Blog instance with the provided data
await newBlog.save(); // Save the new blog post to the database
res.status(201).json(newBlog); // Respond with the created blog post and a 201 status code
} catch (err) {
res.status(500).json({ message: err.message }); // Respond with a 500 status code and error message if something goes wrong
}
};
- Purpose: This function handles the creation of a new blog post.
- Process:
- Extract
title
anddescription
from the request body. - Create a new instance of the
Blog
model with the extracted data. - Save the new blog post to the database.
- Respond with the created blog post and a
201 Created
status code if successful. - If an error occurs, respond with a
500 Internal Server Error
status code and the error message.
- Extract
Get All Blog Posts with Pagination, Search, and Filtering
exports.getBlogs = async (req, res) => {
try {
const { page = 1, limit = 10, search = '' } = req.query; // Destructure page, limit, and search from the query parameters, providing default values
const query = search ? { title: { $regex: search, $options: 'i' } } : {}; // If search is provided, create a query object to search by title
// Find blogs based on query, limit results, and skip pages
const blogs = await Blog.find(query)
.limit(limit * 1) // Convert limit to a number and limit the number of results
.skip((page - 1) * limit) // Skip results to get the correct page
.exec();
// Count total documents that match the query
const count = await Blog.countDocuments(query);
// Respond with blogs, total pages, and current page
res.json({
blogs,
totalPages: Math.ceil(count / limit), // Calculate total pages based on the total count and limit
currentPage: page, // Current page
});
} catch (err) {
res.status(500).json({ message: err.message }); // Respond with a 500 status code and error message if something goes wrong
}
};
- Purpose: This function retrieves all blog posts, supporting pagination, search, and filtering.
- Process:
- Extract
page
,limit
, andsearch
from the query parameters, providing default values. - Create a query object based on the
search
parameter to filter blog posts by title using a regular expression. - Use the query object to find blog posts, applying the limit and skip for pagination.
- Count the total number of documents that match the query.
- Respond with the blog posts, total number of pages, and the current page.
- If an error occurs, respond with a
500 Internal Server Error
status code and the error message.
- Extract
Get a Single Blog Post by ID
exports.getBlogById = async (req, res) => {
try {
const blog = await Blog.findById(req.params.id); // Find the blog post by ID from the request parameters
if (!blog) return res.status(404).json({ message: 'Blog not found' }); // If no blog post is found, respond with a 404 status code
res.json(blog); // Respond with the found blog post
} catch (err) {
res.status(500).json({ message: err.message }); // Respond with a 500 status code and error message if something goes wrong
}
};
- Purpose: This function retrieves a single blog post by its ID.
- Process:
- Find the blog post by ID using the ID from the request parameters.
- If the blog post is not found, respond with a
404 Not Found
status code and an error message. - If the blog post is found, respond with the blog post.
- If an error occurs, respond with a
500 Internal Server Error
status code and the error message.
Update a Blog Post by ID
exports.updateBlog = async (req, res) => {
try {
const { title, description } = req.body; // Destructure title and description from the request body
const blog = await Blog.findByIdAndUpdate(
req.params.id,
{ title, description }, // Update the blog post with the new title and description
{ new: true } // Return the updated document
);
if (!blog) return res.status(404).json({ message: 'Blog not found' }); // If no blog post is found, respond with a 404 status code
res.json(blog); // Respond with the updated blog post
} catch (err) {
res.status(500).json({ message: err.message }); // Respond with a 500 status code and error message if something goes wrong
}
};
- Purpose: This function updates a blog post by its ID.
- Process:
- Extract
title
anddescription
from the request body. - Find the blog post by ID and update it with the new
title
anddescription
, returning the updated document. - If the blog post is not found, respond with a
404 Not Found
status code and an error message. - If the blog post is found and updated, respond with the updated blog post.
- If an error occurs, respond with a
500 Internal Server Error
status code and the error message.
- Extract
Delete a Blog Post by ID
exports.deleteBlog = async (req, res) => {
try {
const blog = await Blog.findByIdAndDelete(req.params.id); // Find the blog post by ID and delete it
if (!blog) return res.status(404).json({ message: 'Blog not found' }); // If no blog post is found, respond with a 404 status code
res.json({ message: 'Blog deleted' }); // Respond with a success message
} catch (err) {
res.status(500).json({ message: err.message }); // Respond with a 500 status code and error message if something goes wrong
}
};
- Purpose: This function deletes a blog post by its ID.
- Process:
- Find the blog post by ID and delete it.
- If the blog post is not found, respond with a
404 Not Found
status code and an error message. - If the blog post is found and deleted, respond with a success message.
- If an error occurs, respond with a
500 Internal Server Error
status code and the error message.
By understanding each of these functions and their steps, you can see how CRUD operations, search, filtering, and pagination are implemented in a Node.js and MongoDB application using Express and Mongoose.
Step 3: Define Routes
Set Up API Endpoints
Create a routes/blogRoutes.js
file to define the API endpoints for the blog posts. These routes will map HTTP requests to the appropriate controller functions.
// routes/blogRoutes.js
const express = require('express');
const router = express.Router();
const blogController = require('../controllers/blogController');
// Route to create a new blog post
router.post('/', blogController.createBlog);
// Route to get all blog posts with pagination, search, and filtering
router.get('/', blogController.getBlogs);
// Route to get a single blog post by ID
router.get('/:id', blogController.getBlogById);
// Route to update a blog post by ID
router.put('/:id', blogController.updateBlog);
// Route to delete a blog post by ID
router.delete('/:id', blogController.deleteBlog);
module.exports = router;
Step 4: Integrate Routes into the App
Main Application File
Import the routes in your app.js
file and use them in the Express app.
// app.js
const express = require('express');
const bodyParser = require('body-parser');
const connectDB = require('./config/database');
const blogRoutes = require('./routes/blogRoutes');
const dotenv = require('dotenv');
// Load environment variables
dotenv.config();
const app = express();
// Connect to database
connectDB();
// Middleware to parse JSON request bodies
app.use(bodyParser.json());
// Use blog routes for API endpoints
app.use('/api/blogs', blogRoutes);
// Error handling middleware
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).json({ message: err.message });
});
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Summary
- Model: Defines the structure of the blog posts.
- Controller: Contains the logic for handling CRUD operations, search, filtering, and pagination.
- Routes: Maps HTTP requests to the controller functions.
- App Initialization: Sets up the Express app, connects to MongoDB, and integrates the routes.
This detailed explanation and step-by-step setup should help you build a robust backend blog application using Node.js, MongoDB, and Express, with search, filtering, and pagination functionalities.
Module 4: Features Implementation
In this module, we will implement the core features of our blog application: CRUD operations (Create, Read, Update, Delete), search, filtering, and pagination. This is where the functionality of the application comes to life.
Step 1: CRUD Operations
We have already defined our CRUD operations in the controllers/blogController.js
file in the previous module. Let’s ensure we understand how each operation works and integrate them properly into our routes.
Create Operation
The createBlog
function allows users to create a new blog post. This function is already defined in our controller.
Read Operations
The getBlogs
function retrieves all blog posts with support for pagination, search, and filtering, while the getBlogById
function retrieves a single blog post by its ID.
Update Operation
The updateBlog
function updates an existing blog post by its ID.
Delete Operation
The deleteBlog
function deletes a blog post by its ID.
Step 2: Integrate Routes
Ensure that our routes are correctly set up to handle these operations.
Routes
// routes/blogRoutes.js
const express = require('express');
const router = express.Router();
const blogController = require('../controllers/blogController');
// Route to create a new blog post
router.post('/', blogController.createBlog);
// Route to get all blog posts with pagination, search, and filtering
router.get('/', blogController.getBlogs);
// Route to get a single blog post by ID
router.get('/:id', blogController.getBlogById);
// Route to update a blog post by ID
router.put('/:id', blogController.updateBlog);
// Route to delete a blog post by ID
router.delete('/:id', blogController.deleteBlog);
module.exports = router;
Integrate these routes into our main application file.
Main Application File
// app.js
const express = require('express');
const bodyParser = require('body-parser');
const connectDB = require('./config/database');
const blogRoutes = require('./routes/blogRoutes');
const dotenv = require('dotenv');
// Load environment variables
dotenv.config();
const app = express();
// Connect to database
connectDB();
// Middleware to parse JSON request bodies
app.use(bodyParser.json());
// Use blog routes for API endpoints
app.use('/api/blogs', blogRoutes);
// Error handling middleware
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).json({ message: err.message });
});
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Step 3: Implement Search, Filtering, and Pagination
We have already included these features in our getBlogs
controller function. Let’s ensure they are properly explained and tested.
Search Functionality
- The
search
query parameter allows users to search for blog posts by title. - We use a regular expression to perform a case-insensitive search.
Filtering Functionality
- Although we only have a
title
field, more complex filtering can be added by extending the query object.
Pagination
- The
page
andlimit
query parameters control the pagination. - The
skip
method is used to skip the appropriate number of documents to fetch the correct page.
Step 4: Testing the Implementation
Using Postman or Similar Tools
Create a New Blog Post
- POST request to
http://localhost:5000/api/blogs
- Body:
{ "title": "My First Blog", "description": "This is the description of my first blog post." }
- Response: The created blog post with a 201 status code.
- POST request to
Get All Blog Posts with Pagination, Search, and Filtering
- GET request to
http://localhost:5000/api/blogs?page=1&limit=10&search=first
- Response: A list of blog posts matching the search criteria, with pagination details.
- GET request to
Get a Single Blog Post by ID
- GET request to
http://localhost:5000/api/blogs/:id
- Response: The blog post with the specified ID.
- GET request to
Update a Blog Post by ID
- PUT request to
http://localhost:5000/api/blogs/:id
- Body:
{ "title": "Updated Title", "description": "Updated description." }
- Response: The updated blog post.
- PUT request to
Delete a Blog Post by ID
- DELETE request to
http://localhost:5000/api/blogs/:id
- Response: Success message indicating the blog post was deleted.
- DELETE request to
Module 5: Error Handling and Validation
In this module, we will focus on enhancing our application by implementing robust error handling and request validation. Proper error handling ensures that our application can gracefully handle unexpected issues, while request validation helps maintain data integrity by ensuring that the data received in requests meets the required criteria.
Step 1: Error Handling Middleware
We will create a centralized error handling middleware to manage errors consistently across the application.
Error Handling Middleware
Create a middlewares/errorHandler.js
file:
// middlewares/errorHandler.js
const errorHandler = (err, req, res, next) => {
console.error(err.stack); // Log the error stack trace for debugging purposes
res.status(500).json({ message: err.message }); // Respond with a 500 status code and error message
};
module.exports = errorHandler;
Integrate this middleware into our main application file:
Main Application File
// app.js
const express = require('express');
const bodyParser = require('body-parser');
const connectDB = require('./config/database');
const blogRoutes = require('./routes/blogRoutes');
const errorHandler = require('./middlewares/errorHandler');
const dotenv = require('dotenv');
// Load environment variables
dotenv.config();
const app = express();
// Connect to database
connectDB();
// Middleware to parse JSON request bodies
app.use(bodyParser.json());
// Use blog routes for API endpoints
app.use('/api/blogs', blogRoutes);
// Error handling middleware
app.use(errorHandler);
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Step 2: Request Validation
We will use express-validator
to validate incoming requests and ensure the data is correct before it reaches our controllers.
Install express-validator
npm install express-validator
Create Validation Middleware
Create a middlewares/validators.js
file to define our validation rules:
// middlewares/validators.js
const { body, validationResult } = require('express-validator');
// Validation rules for creating and updating a blog post
const blogValidationRules = [
body('title').notEmpty().withMessage('Title is required'),
body('description').notEmpty().withMessage('Description is required'),
];
// Middleware to check for validation errors
const validate = (req, res, next) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
next();
};
module.exports = {
blogValidationRules,
validate,
};
Update Routes to Include Validation
Update the routes/blogRoutes.js
file to include the validation middleware:
// routes/blogRoutes.js
const express = require('express');
const router = express.Router();
const blogController = require('../controllers/blogController');
const { blogValidationRules, validate } = require('../middlewares/validators');
// Route to create a new blog post with validation
router.post('/', blogValidationRules, validate, blogController.createBlog);
// Route to get all blog posts with pagination, search, and filtering
router.get('/', blogController.getBlogs);
// Route to get a single blog post by ID
router.get('/:id', blogController.getBlogById);
// Route to update a blog post by ID with validation
router.put('/:id', blogValidationRules, validate, blogController.updateBlog);
// Route to delete a blog post by ID
router.delete('/:id', blogController.deleteBlog);
module.exports = router;
Update Controller to Handle Validation Errors
No changes are needed in the controller functions, as the validation middleware will handle the validation errors before they reach the controllers.
Step 3: Testing Error Handling and Validation
Using Postman or Similar Tools
Test Creating a New Blog Post with Invalid Data
- POST request to
http://localhost:5000/api/blogs
- Body:
{ "title": "", "description": "" }
- Response: A 400 status code with validation error messages indicating that title and description are required.
- POST request to
Test Updating a Blog Post with Invalid Data
- PUT request to
http://localhost:5000/api/blogs/:id
- Body:
{ "title": "", "description": "" }
- Response: A 400 status code with validation error messages indicating that title and description are required.
- PUT request to
Test Creating, Reading, Updating, and Deleting Blog Posts with Valid Data
- Ensure that valid requests are processed correctly and any errors are handled gracefully.
Module 6: Documentation and GitHub Integration
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
In this module, we will focus on documenting our API and integrating our project with GitHub. Proper documentation is essential for anyone who wants to use or contribute to the project. Additionally, version control with GitHub will help manage our codebase and track changes effectively.
Step 1: API Documentation
We will document our API endpoints, including the request parameters, response format, and possible error messages. This can be done using a README file or a more interactive tool like Swagger.
Option 1: README File
Create a README.md
file in the root of your project directory and document your API endpoints.
Example README.md
# Blog API
This is a simple blog API built with Node.js, Express, and MongoDB.
## Endpoints
### Create a New Blog Post
- **URL:** `/api/blogs`
- **Method:** `POST`
- **Body:**
```json
{
"title": "Your Blog Title",
"description": "Your Blog Description"
}
- Responses:
201 Created
: The created blog post.400 Bad Request
: Validation errors.
Get All Blog Posts
- URL:
/api/blogs
- Method:
GET
- Query Parameters:
page
(optional): Page number for pagination (default: 1)limit
(optional): Number of posts per page (default: 10)search
(optional): Search term to filter posts by title
- Responses:
200 OK
: An array of blog posts, along with pagination details.
Get a Single Blog Post by ID
- URL:
/api/blogs/:id
- Method:
GET
- Responses:
200 OK
: The requested blog post.404 Not Found
: Blog post not found.
Update a Blog Post by ID
- URL:
/api/blogs/:id
- Method:
PUT
- Body
{
"title": "Updated Title",
"description": "Updated Description"
}
- Responses:
200 OK
: The updated blog post.400 Bad Request
: Validation errors.404 Not Found
: Blog post not found.
Delete a Blog Post by ID
- URL:
/api/blogs/:id
- Method:
DELETE
- Responses:
200 OK
: Success message indicating the blog post was deleted.404 Not Found
: Blog post not found.
Error Handling
500 Internal Server Error
: An error occurred on the server.
Environment Variables
PORT
: The port on which the server runs (default: 5000).MONGODB_URI
: The MongoDB connection URI.
**Option 2: Swagger**
Swagger is a tool for documenting APIs and can generate an interactive API documentation page.
**Install Swagger UI Express**
```bash
npm install swagger-ui-express swagger-jsdoc
Set Up Swagger
Create a swagger.js
file to configure Swagger:
// swagger.js
const swaggerJsDoc = require('swagger-jsdoc');
const swaggerUi = require('swagger-ui-express');
const swaggerOptions = {
swaggerDefinition: {
info: {
title: 'Blog API',
version: '1.0.0',
description: 'API documentation for the Blog API',
},
servers: [
{
url: 'http://localhost:5000',
},
],
},
apis: ['./routes/*.js'],
};
const swaggerDocs = swaggerJsDoc(swaggerOptions);
module.exports = (app) => {
app.use('/api-docs', swaggerUi.serve, swaggerUi.setup(swaggerDocs));
};
Integrate Swagger in app.js
:
// app.js
const express = require('express');
const bodyParser = require('body-parser');
const connectDB = require('./config/database');
const blogRoutes = require('./routes/blogRoutes');
const errorHandler = require('./middlewares/errorHandler');
const swaggerSetup = require('./swagger');
const dotenv = require('dotenv');
// Load environment variables
dotenv.config();
const app = express();
// Connect to database
connectDB();
// Middleware to parse JSON request bodies
app.use(bodyParser.json());
// Use blog routes for API endpoints
app.use('/api/blogs', blogRoutes);
// Error handling middleware
app.use(errorHandler);
// Setup Swagger
swaggerSetup(app);
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Add Swagger comments in routes/blogRoutes.js
:
// routes/blogRoutes.js
const express = require('express');
const router = express.Router();
const blogController = require('../controllers/blogController');
const { blogValidationRules, validate } = require('../middlewares/validators');
/**
* @swagger
* /api/blogs:
* post:
* description: Create a new blog post
* responses:
* 201:
* description: Created
* 400:
* description: Validation errors
*/
router.post('/', blogValidationRules, validate, blogController.createBlog);
/**
* @swagger
* /api/blogs:
* get:
* description: Get all blog posts with pagination, search, and filtering
* responses:
* 200:
* description: OK
*/
router.get('/', blogController.getBlogs);
/**
* @swagger
* /api/blogs/{id}:
* get:
* description: Get a single blog post by ID
* responses:
* 200:
* description: OK
* 404:
* description: Blog not found
*/
router.get('/:id', blogController.getBlogById);
/**
* @swagger
* /api/blogs/{id}:
* put:
* description: Update a blog post by ID
* responses:
* 200:
* description: OK
* 400:
* description: Validation errors
* 404:
* description: Blog not found
*/
router.put('/:id', blogValidationRules, validate, blogController.updateBlog);
/**
* @swagger
* /api/blogs/{id}:
* delete:
* description: Delete a blog post by ID
* responses:
* 200:
* description: OK
* 404:
* description: Blog not found
*/
router.delete('/:id', blogController.deleteBlog);
module.exports = router;
Step 2: GitHub Integration
Initialize Git Repository
git init
Create .gitignore
File
Create a .gitignore
file to exclude certain files and directories from being tracked by Git:
node_modules/
.env
node_modules/
.env
Commit and Push to GitHub
- Add Files to Git
git add .
2. Commit Changes
3. Push to GitHub
- Create a new repository on GitHub.
- Follow the instructions to push your local repository to GitHub:
git remote add origin https://github.com/your-username/your-repository.git
git branch -M main
git push -u origin main
GitHub Integration with Visual Studio Code
Open Project in VS Code
- Open your project folder in Visual Studio Code.
Use Git Integration
- Use the built-in Git integration in VS Code to manage your repository, track changes, commit, and push to GitHub.
git commit -m "Initial commit"
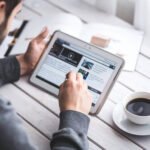
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.