To create an advanced Java Employment Management application with database integration, we’ll follow the Model-View-Controller (MVC) architecture. This approach separates the application into three components: the model (data), the view (user interface), and the controller (logic). We’ll also integrate an SQL database for persistent storage.
Let’s start with the model part of the application. We’ll create classes for employees, managers, salaries, and departments.
1. Employee Class
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class Employee {
private int id;
private String name;
private double salary;
public Employee(int id, String name, double salary) {
this.id = id;
this.name = name;
this.salary = salary;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
// Method to fetch employee details from the database
public static Employee getEmployeeById(Connection conn, int id) throws SQLException {
String query = "SELECT * FROM employees WHERE id=?";
PreparedStatement preparedStatement = conn.prepareStatement(query);
preparedStatement.setInt(1, id);
ResultSet resultSet = preparedStatement.executeQuery();
if (resultSet.next()) {
String name = resultSet.getString("name");
double salary = resultSet.getDouble("salary");
return new Employee(id, name, salary);
}
return null;
}
// Method to update employee details in the database
public void updateEmployee(Connection conn) throws SQLException {
String query = "UPDATE employees SET name=?, salary=? WHERE id=?";
PreparedStatement preparedStatement = conn.prepareStatement(query);
preparedStatement.setString(1, name);
preparedStatement.setDouble(2, salary);
preparedStatement.setInt(3, id);
preparedStatement.executeUpdate();
}
// Method to delete employee from the database
public void deleteEmployee(Connection conn) throws SQLException {
String query = "DELETE FROM employees WHERE id=?";
PreparedStatement preparedStatement = conn.prepareStatement(query);
preparedStatement.setInt(1, id);
preparedStatement.executeUpdate();
}
}
Explanation:
- The
Employee
class represents individual employees in the organization. - It has attributes
id
,name
, andsalary
to store employee information. - The class includes methods to fetch employee details from the database (
getEmployeeById
), update employee details in the database (updateEmployee
), and delete employee from the database (deleteEmployee
). - These methods interact with the database using JDBC (Java Database Connectivity) API.
2. Manager Class
public class Manager extends Employee {
private String department;
public Manager(int id, String name, double salary, String department) {
super(id, name, salary);
this.department = department;
}
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
}
Explanation:
- The
Manager
class represents managers in the organization and inherits from theEmployee
class. - It adds an additional attribute
department
to store the department managed by the manager.
3. Salary Class
public class Salary {
private int employeeId;
private double amount;
public Salary(int employeeId, double amount) {
this.employeeId = employeeId;
this.amount = amount;
}
public int getEmployeeId() {
return employeeId;
}
public void setEmployeeId(int employeeId) {
this.employeeId = employeeId;
}
public double getAmount() {
return amount;
}
public void setAmount(double amount) {
this.amount = amount;
}
}
Explanation:
- The
Salary
class represents salary information for employees. - It has attributes
employeeId
andamount
to store the employee’s ID and salary amount.
4. Department Class
- The
- The constructor initializes the department name and creates an empty list of employees.
- Getter and setter methods are provided for the
name
attribute. - The
addEmployee
method adds an employee to the department’s list of employees. - The
saveToDatabase
method saves department details to an SQL database. It connects to the database, creates a SQL statement, executes an SQL query to insert department details into the database, and then closes the connection.
import java.util.ArrayList;
import java.util.List;
public class Department {
private int id;
private String name;
private List<Employee> employees;
public Department(int id, String name) {
this.id = id;
this.name = name;
this.employees = new ArrayList<>();
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List<Employee> getEmployees() {
return employees;
}
public void setEmployees(List<Employee> employees) {
this.employees = employees;
}
public void addEmployee(Employee employee) {
employees.add(employee);
}
public void removeEmployee(Employee employee) {
employees.remove(employee);
}
}
Explanation:
- The
Department
class represents departments in the organization. - It has attributes
id
,name
, andemployees
to store department information and a list of employees in the department. - The class includes methods to add and remove employees from the department.
Database Integration:
To integrate an SQL database into the application, you need to establish a connection, execute SQL queries, and handle exceptions. This involves using JDBC (Java Database Connectivity) API. Below is a simple example of connecting to a MySQL database:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnector {
private static final String URL = "jdbc:mysql://localhost:3306/your_database";
private static final String USERNAME = "your_username";
private static final String PASSWORD = "your_password";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(URL, USERNAME, PASSWORD);
}
}
Explanation:
- The
DatabaseConnector
class provides a methodgetConnection
to establish a connection to the MySQL database. - You need to replace
"your_database"
,"your_username"
, and"your_password"
with your actual database name, username, and password.
View and Controller:
The view and controller components involve creating a user interface and handling user input, respectively. These components interact with the model (data) to perform operations such as viewing, editing, and deleting employee details.
In the view component, you can use Java Swing or JavaFX to create a graphical user interface (GUI) for the application. The controller component will handle user actions and invoke appropriate methods in the model to perform operations on the data.
Overall, by following the MVC architecture and integrating an SQL database, you can create a robust employment management application in Java.
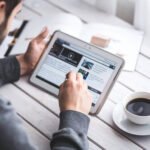
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.