This example will include basic functionalities such as creating an account, depositing funds, withdrawing funds, and checking the balance. Keep in mind that this is a basic tutorial and doesn’t cover all aspects of a real-world banking application (e.g., security, concurrency, database integration).
class BankAccount:
# Define a class representing a bank account
def __init__(self, account_number, holder_name):
# Constructor to initialize the account with account number and holder name
self.account_number = account_number # Stores the account number
self.holder_name = holder_name # Stores the name of the account holder
self.balance = 0.0 # Initialize balance to 0
def deposit(self, amount):
# Method to deposit funds into the account
self.balance += amount # Add the deposited amount to the balance
print(f"{amount} deposited successfully.") # Print a success message
def withdraw(self, amount):
# Method to withdraw funds from the account
if self.balance >= amount: # Check if the balance is sufficient for withdrawal
self.balance -= amount # Subtract the withdrawal amount from the balance
print(f"{amount} withdrawn successfully.") # Print a success message
else:
print("Insufficient funds.") # Print an error message if balance is insufficient
def get_balance(self):
# Method to get the current balance of the account
return self.balance # Return the current balance
def get_account_number(self):
# Method to get the account number
return self.account_number # Return the account number
def get_holder_name(self):
# Method to get the holder name
return self.holder_name # Return the holder name
def main():
# Main function for the banking application
print("Welcome to Our Bank!") # Display a welcome message
account_number = input("Enter your account number: ") # Prompt user to enter account number
holder_name = input("Enter your name: ") # Prompt user to enter their name
account = BankAccount(account_number, holder_name) # Create a BankAccount object
# Display a menu for banking operations
while True:
print("\n1. Deposit")
print("2. Withdraw")
print("3. Check Balance")
print("4. Exit")
choice = int(input("Enter your choice: ")) # Read user's choice
if choice == 1: # If user chooses deposit
deposit_amount = float(input("Enter deposit amount: ")) # Read deposit amount
account.deposit(deposit_amount) # Call deposit method
elif choice == 2: # If user chooses withdraw
withdraw_amount = float(input("Enter withdrawal amount: ")) # Read withdrawal amount
account.withdraw(withdraw_amount) # Call withdraw method
elif choice == 3: # If user chooses check balance
print("Account Holder:", account.get_holder_name())
print("Account Number:", account.get_account_number())
print("Balance:", account.get_balance())
elif choice == 4: # If user chooses exit
print("Thank you for using our bank!")
break # Exit the loop and end the program
else: # If user enters an invalid choice
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
This code sets up a basic banking application where users can deposit, withdraw, and check their balance. The BankAccount
class represents a bank account with attributes such as account number, holder name, and balance. The main
method in the BankingApplication
class serves as the entry point for the application. It prompts the user for their account number and name, creates a BankAccount
object, and then presents a menu for performing banking operations.
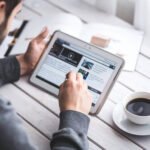