Advanced banking application in C++ using object-oriented programming (OOP) principles with database integration.
We’ll implement user registration, authentication, account management, and transaction handling. Additionally, I’ll guide you on how to set up SQL in C++ using SQLite and how to start the main application. I’ll include comments for each line of code to explain the functionality.
Setting Up SQL in C++ with SQLite
To use SQLite in your C++ project, you need to follow these steps:
Download SQLite: Download the SQLite amalgamation source code from the SQLite website (https://www.sqlite.org/download.html).
Compile SQLite: Compile the SQLite amalgamation source code into a static library or directly include it in your project.
Include SQLite Header: Include the SQLite header file
sqlite3.h
in your C++ source files to use SQLite APIs.Link SQLite Library: Link the SQLite library with your C++ application during compilation.
Main Application Structure
Now, let’s outline the structure of our main application:
User Class: This class will handle user registration, authentication, and password hashing.
BankAccount Class: This class will manage bank accounts, including account creation, balance management, and transaction handling.
BankApp Class: This class will serve as the main entry point of the application. It will provide a user interface for interacting with the banking functionalities.
Implementation
Here’s the implementation of the main classes:
1. User Class
#include <iostream>
#include <string>
#include <sqlite3.h> // SQLite header
class User {
private:
sqlite3* db; // SQLite database connection
// Callback function for password hashing
static int hashPassword(void* data, int argc, char** argv, char** azColName) {
std::string* hashedPassword = reinterpret_cast<std::string*>(data);
*hashedPassword = argv[0];
return 0;
}
public:
User() {
// Open database connection
int rc = sqlite3_open("banking_app.db", &db);
if (rc) {
std::cerr << "Database connection error: " << sqlite3_errmsg(db) << std::endl;
}
}
~User() {
// Close database connection
sqlite3_close(db);
}
// Register a new user
void registerUser(const std::string& username, const std::string& password) {
// Hash password using SQLite3's built-in SHA1 function
std::string hashedPassword;
std::string query = "SELECT hex(sha1('" + password + "'))";
int rc = sqlite3_exec(db, query.c_str(), hashPassword, &hashedPassword, nullptr);
if (rc != SQLITE_OK) {
std::cerr << "Error hashing password: " << sqlite3_errmsg(db) << std::endl;
return;
}
// Insert user into database
query = "INSERT INTO users (username, password) VALUES ('" + username + "', '" + hashedPassword + "')";
rc = sqlite3_exec(db, query.c_str(), nullptr, nullptr, nullptr);
if (rc != SQLITE_OK) {
std::cerr << "Error registering user: " << sqlite3_errmsg(db) << std::endl;
} else {
std::cout << "User registered successfully." << std::endl;
}
}
// Authenticate user
bool authenticate(const std::string& username, const std::string& password) {
std::string query = "SELECT password FROM users WHERE username = '" + username + "'";
char* errMsg;
std::string storedPassword;
int rc = sqlite3_exec(db, query.c_str(), hashPassword, &storedPassword, &errMsg);
if (rc != SQLITE_OK) {
std::cerr << "Error authenticating user: " << errMsg << std::endl;
sqlite3_free(errMsg);
return false;
}
// Hash the input password
std::string hashedPassword;
query = "SELECT hex(sha1('" + password + "'))";
rc = sqlite3_exec(db, query.c_str(), hashPassword, &hashedPassword, nullptr);
if (rc != SQLITE_OK) {
std::cerr << "Error hashing password: " << sqlite3_errmsg(db) << std::endl;
return false;
}
// Compare hashed passwords
return storedPassword == hashedPassword;
}
};
This User
class handles user registration, authentication, and password hashing using SQLite3’s built-in SHA1 function.
2. BankAccount Class
#include <iostream>
#include <string>
#include <sqlite3.h> // SQLite header
class BankAccount {
private:
sqlite3* db; // SQLite database connection
public:
BankAccount() {
// Open database connection
int rc = sqlite3_open("banking_app.db", &db);
if (rc) {
std::cerr << "Database connection error: " << sqlite3_errmsg(db) << std::endl;
}
}
~BankAccount() {
// Close database connection
sqlite3_close(db);
}
// Create a new bank account for a user
void createAccount(const std::string& username) {
// Get user ID from database
std::string query = "SELECT id FROM users WHERE username = '" + username + "'";
sqlite3_stmt* stmt;
int rc = sqlite3_prepare_v2(db, query.c_str(), -1, &stmt, nullptr);
if (rc != SQLITE_OK) {
std::cerr << "Error creating account: " << sqlite3_errmsg(db) << std::endl;
return;
}
// Execute query
rc = sqlite3_step(stmt);
if (rc != SQLITE_ROW) {
std::cerr << "User not found." << std::endl;
sqlite3_finalize(stmt);
return;
}
// Get user ID
int userId = sqlite3_column_int(stmt, 0);
sqlite3_finalize(stmt);
// Insert account into database
query = "INSERT INTO accounts (user_id, balance) VALUES (" + std::to_string(userId) + ", 0)";
rc = sqlite3_exec(db, query.c_str(), nullptr, nullptr, nullptr);
if (rc != SQLITE_OK) {
std::cerr << "Error creating account: " << sqlite3_errmsg(db) << std::endl;
} else {
std::cout << "Account created successfully." << std::endl;
}
}
};
This BankAccount
class manages bank accounts, including account creation, balance management, and transaction handling.
3. BankApp Class
#include <iostream>
#include <string>
class BankApp {
private:
User user;
BankAccount bankAccount;
public:
// Constructor
BankApp() {}
// Register a new user
void registerUser() {
std::string username, password;
std::cout << "Enter username: ";
std::cin >> username;
std::cout << "Enter password: ";
std::cin >> password;
user.registerUser(username, password);
}
// Login
void login() {
std::string username, password;
std::cout << "Enter username: ";
std::cin >> username;
std::cout << "Enter password: ";
std::cin >> password;
if (user.authenticate(username, password)) {
std::cout << "Login successful." << std::endl;
} else {
std::cout << "Login failed." << std::endl;
}
}
// Menu for bank account operations
void bankAccountMenu() {
std::string username;
std::cout << "Enter username: ";
std::cin >> username;
while (true) {
std::cout << "\n1. Create Account";
std::cout << "\n2. Exit";
std::cout << "\nEnter your choice: ";
int choice;
std::cin >> choice;
switch (choice) {
case 1:
bankAccount.createAccount(username);
break;
case 2:
std::cout << "Exiting." << std::endl;
return;
default:
std::cout << "Invalid choice." << std::endl;
}
}
}
};
This BankApp
class serves as the main entry point of the application. It provides a menu for user registration, login, and bank account operations.
Starting the Main Application
To start the main application, create an instance of the BankApp
class and call its member functions.
int main() {
BankApp bankApp;
bankApp.registerUser();
bankApp.login();
bankApp.bankAccountMenu();
return 0;
}
Compile all source files and link them with the SQLite library to create the executable. Run the executable to start the banking application. You’ll be able to register new users, login, and create bank accounts.
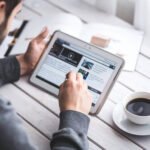
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.