In Java, you can display the current date and time using the System.currentTimeMillis()
method or the java.time
package, which was introduced in Java 8. Here are some examples:
Using System.currentTimeMillis()
You can get the current time in milliseconds since the Unix Epoch (January 1, 1970) using the System.currentTimeMillis()
method. You can then create a java.sql.Date
object using this value to display the current date.
Here’s an example:
long millis=System.currentTimeMillis();
java.sql.Date date=new java.sql.Date(millis);
System.out.println(date);
Using java.time
package
The java.time
package provides several classes for working with dates and times, such as LocalDateTime
, LocalDate
, LocalTime
, and ZonedDateTime
.
Here are some examples:
LocalDateTime
This method returns the current date and time:
System.out.println(java.time.LocalDateTime.now());
Local Date
This method simply returns the current date:
LocalDate date = LocalDate.now();
LocalTime
This method simply returns the current time and can be formatted:
LocalTime time = LocalTime.now();
ZonedDateTime
This method is used according to the time zone and can be implemented in the following way:
ZonedDateTime dateTime = ZonedDateTime.now();
Here’s an example program that displays the current date and time using the java.time
package:
import java.time.LocalDateTime;
import java.time.LocalDate;
import java.time.LocalTime;
import java.time.ZonedDateTime;
public class Main {
public static void main(String[] args) {
// Get current date and time
LocalDateTime now = LocalDateTime.now();
System.out.println("Current date and time: " + now);
// Get current date
LocalDate date = LocalDate.now();
System.out.println("Current date: " + date);
// Get current time
LocalTime time = LocalTime.now();
System.out.println("Current time: " + time);
// Get current date and time with time zone
ZonedDateTime zone = ZonedDateTime.now();
System.out.println("Current date and time with time zone: " + zone);
}
}
When you run this program, it will display the current date and time in your local time zone.
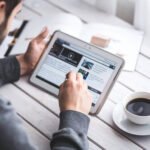
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.