In this tutorial, we’ll guide you through the process of creating a Customer Management System in C++ using SQLite. We’ll cover setting up the SQLite database, creating a C++ application to interact with the database, implementing CRUD (Create, Read, Update, Delete) operations on customer data, and demonstrating the functionality with example code.
1. Setting Up SQLite Database
SQLite is a self-contained, serverless, zero-configuration, and transactional SQL database engine. It is embedded within the application and doesn’t require a separate server process.
First, ensure you have SQLite installed on your system. You can download SQLite from its official website (https://www.sqlite.org/download.html) or use a package manager like Homebrew on macOS or apt on Ubuntu.
Once SQLite is installed, create a new SQLite database file. You can do this using the SQLite command-line interface or any SQLite database management tool. Let’s name our database file customer_management.db
.
2. Creating the C++ Application
Now, let’s create a C++ application that interacts with our SQLite database to manage customer data. We’ll perform the following steps:
- Connect to the SQLite database.
- Implement methods to perform CRUD operations on customer data.
- Demonstrate the usage of these methods with example code.
3. Connecting to SQLite Database
To connect to our SQLite database from C++, we’ll use the SQLite C/C++ Interface (https://www.sqlite.org/capi3ref.html). This interface provides functions for executing SQL statements and accessing the results.
First, ensure you have the SQLite library installed on your system. You can download the SQLite amalgamation from its official website and include the SQLite header file (sqlite3.h
) in your project.
Next, let’s establish a connection to our SQLite database:
#include <iostream>
#include <sqlite3.h>
// Function to handle database connection
sqlite3* createConnection(const char* dbFile) {
sqlite3* db;
int rc = sqlite3_open(dbFile, &db);
if (rc) {
std::cerr << "Can't open database: " << sqlite3_errmsg(db) << std::endl;
sqlite3_close(db);
return nullptr;
} else {
std::cout << "Connected to database: " << dbFile << std::endl;
return db;
}
}
int main() {
const char* database = "customer_management.db";
sqlite3* db = createConnection(database);
if (!db) {
return 1; // Exit with error
}
// Database connection established successfully
sqlite3_close(db);
return 0;
}
In the createConnection
function, we use sqlite3_open
to establish a connection to the SQLite database specified by the dbFile
parameter. If the connection is successful, a confirmation message is printed; otherwise, an error message is displayed.
4. Implementing CRUD Operations
Next, let’s implement methods to perform CRUD operations on customer data:
- Create a new customer.
- Retrieve all customers.
- Update customer details.
- Delete a customer.
Here’s the implementation:
// Function to create a new customer
int createCustomer(sqlite3* db, const char* name, const char* email, const char* phoneNumber) {
char* errMsg;
std::string sql = "INSERT INTO customers(name, email, phone_number) VALUES('" + std::string(name) + "', '" + std::string(email) + "', '" + std::string(phoneNumber) + "')";
int rc = sqlite3_exec(db, sql.c_str(), nullptr, 0, &errMsg);
if (rc != SQLITE_OK) {
std::cerr << "SQL error: " << errMsg << std::endl;
sqlite3_free(errMsg);
return -1;
} else {
return sqlite3_last_insert_rowid(db);
}
}
// Function to retrieve all customers
void getAllCustomers(sqlite3* db) {
sqlite3_stmt* stmt;
const char* sql = "SELECT * FROM customers";
int rc = sqlite3_prepare_v2(db, sql, -1, &stmt, nullptr);
if (rc != SQLITE_OK) {
std::cerr << "Failed to prepare statement: " << sqlite3_errmsg(db) << std::endl;
return;
}
std::cout << "All Customers:" << std::endl;
while ((rc = sqlite3_step(stmt)) == SQLITE_ROW) {
std::cout << sqlite3_column_int(stmt, 0) << "\t" <<
sqlite3_column_text(stmt, 1) << "\t" <<
sqlite3_column_text(stmt, 2) << "\t" <<
sqlite3_column_text(stmt, 3) << std::endl;
}
sqlite3_finalize(stmt);
}
// Function to update customer details
void updateCustomer(sqlite3* db, int id, const char* name, const char* email, const char* phoneNumber) {
char* errMsg;
std::string sql = "UPDATE customers SET name = '" + std::string(name) + "', email = '" + std::string(email) + "', phone_number = '" + std::string(phoneNumber) + "' WHERE id = " + std::to_string(id);
int rc = sqlite3_exec(db, sql.c_str(), nullptr, 0, &errMsg);
if (rc != SQLITE_OK) {
std::cerr << "SQL error: " << errMsg << std::endl;
sqlite3_free(errMsg);
}
}
// Function to delete a customer
void deleteCustomer(sqlite3* db, int id) {
char* errMsg;
std::string sql = "DELETE FROM customers WHERE id = " + std::to_string(id);
int rc = sqlite3_exec(db, sql.c_str(), nullptr, 0, &errMsg);
if (rc != SQLITE_OK) {
std::cerr << "SQL error: " << errMsg << std::endl;
sqlite3_free(errMsg);
}
}
In these methods, we execute SQL queries using sqlite3_exec
to perform CRUD operations on the database. The createCustomer
method inserts a new customer into the customers
table, getAllCustomers
retrieves all customers, updateCustomer
updates the details of an existing customer, and deleteCustomer
deletes a customer based on their ID.
5. Demonstrating Usage
Finally, let’s demonstrate the usage of these methods in the main
function:
int main() {
const char* database = "customer_management.db";
sqlite3* db = createConnection(database);
if (!db) {
return 1; // Exit with error
}
// Create a new customer
int customerId = createCustomer(db, "John Doe", "john@example.com", "1234567890");
if (customerId != -1) {
std::cout << "New customer ID: " << customerId << std::endl;
}
// Retrieve all customers
getAllCustomers(db);
// Update customer details
updateCustomer(db, customerId, "Jane Smith", "jane@example.com", "0987654321");
// Retrieve all customers after update
getAllCustomers(db);
// Delete a customer
deleteCustomer(db, customerId);
std::cout << "Deleted customer with ID: " << customerId << std::endl;
sqlite3_close(db);
return 0;
}
In the main
function, we establish a connection to the SQLite database, create a new customer, retrieve all customers, update a customer’s details, retrieve all customers again to see the update, and finally delete the customer we created.
Conclusion
In this tutorial, we’ve learned how to build a Customer Management System in C++ using SQLite. We covered setting up the SQLite database, connecting to it from C++, implementing CRUD operations on customer data, and demonstrating the usage of these operations with example code. This project serves as a foundation for building more complex database-driven applications in C++.
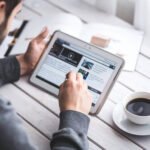
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.