Welcome to this tutorial where you’ll learn how to create a simple form and add custom validation rules to its fields upon submission in a React app.
In this guide, we’ll walk through the process of implementing custom form validation and handling form data in a React application. Specifically, we’ll integrate Bootstrap 4 to enhance the styling of our forms.
Let’s dive into the step-by-step instructions to add custom validation with forms in a React.js app!
Step 1 – Create React App
In this step, open your terminal and execute the following command on your terminal to create a new react app:
npx create-react-app my-react-app
To run the React app, execute the following command on your terminal and check out your React app on this URL: localhost:3000
npm start
Step 2 – Install React Bootstrap
In this step, execute the following command to install react boostrap library into your react app and Add bootstrap.min.css file in src/App.js
file:
npm install bootstrap --save
npm install react-bootstrap bootstrap
import React, { Component } from 'react'
import '../node_modules/bootstrap/dist/css/bootstrap.min.css';
function App() {
return (
<div>
<h2>How to Add Custom Validatin with Forms in React</h2>
</div>
);
}
export default App;
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Step 3 – Creating a Custom Form Component with Validation
To proceed, follow these steps to create the CustomFormValidation.js
file:
- Navigate to the
src
directory of your React.js app. - Create a new file named
CustomFormValidation.js
. - Add the following code into the newly created file:
import React, { useState } from 'react';
const defaultState = {
name: null,
email: null,
password: null,
nameError: null,
emailError: null,
passwordError: null
};
const CustomFormValidation = () => {
const [formData, setFormData] = useState(defaultState);
const handleInputChange = (event) => {
const { name, value } = event.target;
setFormData({ ...formData, [name]: value });
};
const validate = () => {
let nameError = "";
let emailError = "";
let passwordError = "";
if (!formData.name) {
nameError = "Name field is required";
}
const reg = /^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/;
if (!formData.email || reg.test(formData.email) === false) {
emailError = "Email Field is Invalid ";
}
if (!formData.password) {
passwordError = "Password field is required";
}
if (emailError || nameError || passwordError) {
setFormData({ ...formData, nameError, emailError, passwordError });
return false;
}
return true;
};
const submit = () => {
if (validate()) {
console.warn(formData);
setFormData(defaultState);
}
};
return (
<div>
<div className="row">
<div className="col-md-6 offset-md-3">
<h3>React Custom Form Validation Example - Tutsmake.com</h3><br />
<div className="form-row">
<div className="form-group col-md-6">
<label>Name :</label>
<input type="text" className="form-control" name="name" value={formData.name || ''} onChange={handleInputChange} />
<span className="text-danger">{formData.nameError}</span>
</div>
</div>
<div className="form-row">
<div className="form-group col-md-6">
<label>Email :</label>
<input type="email" className="form-control" name="email" value={formData.email || ''} onChange={handleInputChange} />
<span className="text-danger">{formData.emailError}</span>
</div>
</div>
<div className="form-row">
<div className="form-group col-md-6">
<label>Password :</label>
<input type="password" className="form-control" name="password" value={formData.password || ''} onChange={handleInputChange} />
<span className="text-danger">{formData.passwordError}</span>
</div>
</div>
<div className="form-row">
<div className="col-md-12 text-center">
<button type="submit" className="btn btn-primary" onClick={submit}>Submit</button>
</div>
</div>
</div>
</div>
</div>
);
};
export default CustomFormValidation;
Explanation:
useState Hook:
useState
hook is used to initialize and manage the component’s state. TheformData
state contains form data and error messages.
Input Change Handler:
handleInputChange
function is used to update the state when input values change. It uses object destructuring to extractname
andvalue
from the input element, then updates the state using the spread operator.
Validation Function:
validate
function is responsible for validating the form fields. It checks if thename
,email
, andpassword
fields are filled correctly. If any field is invalid, it sets the corresponding error message in the state.
Submit Handler:
submit
function is called when the form is submitted. It first validates the form using thevalidate
function. If the form is valid, it logs the form data to the console and resets the state todefaultState
.
Rendering:
- JSX is used to render the form elements. Each input field (
name
,email
,password
) is associated with its corresponding state value andhandleInputChange
function. - Error messages are displayed if there are validation errors.
- The submit button triggers the
submit
function when clicked.
- JSX is used to render the form elements. Each input field (
Export:
- The
CustomFormValidation
component is exported as the default export of the module.
- The
This functional component achieves the same functionality as the class component but uses React hooks for state management.
Step 4 – Add Custom Form Component in App.js
In this step, you need to add CustomFormValidation.js file in src/App.js
file:
import React from 'react';
import '../node_modules/bootstrap/dist/css/bootstrap.min.css';
import CustomFormValidation from './CustomFormValidation'
function App() {
return (
<div className="App">
<CustomFormValidation />
</div>
);
}
export default App;
This code sets up the main entry point for a React application. Let’s break it down:
Imports:
- The code imports
React
from the ‘react’ package. - It also imports the Bootstrap CSS file from the installed Bootstrap package.
- The code imports
App Function:
- The
App
function is defined as a functional component. - Inside the function, it returns JSX that renders the
CustomFormValidation
component.
- The
Rendering:
- The
CustomFormValidation
component is rendered inside a<div>
element with the class name “App”. This is the root component of the application.
- The
Export:
- The
App
component is exported as the default export of the module, making it accessible to other parts of the application.
- The
Overall, this code sets up the main structure of the React application by rendering the CustomFormValidation
component inside the App
component. The Bootstrap CSS is also imported to provide styling to the application.
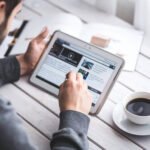
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.