In this project, we will create a simple landing page using React and focus on building a responsive layout with CSS Flexbox. I’ll guide you through each step in a classroom-like style, breaking down every part so it’s easy to understand. Let’s start from scratch and work our way through setting up, building, and styling the page.
Step 1: Setting Up Our Project with Vite
Explanation:
We will use Vite to create a new React project because it’s faster and simpler than traditional React setups. Vite gives us an easy environment to write and preview our code.
Instructions:
Open your terminal and type the following command to create a new React application using Vite:
npm create vite@latest simple-landing-page -- --template react
- This command creates a new folder named
simple-landing-page
with all the basic files we need to start our project. - The
--template react
part tells Vite that we want a React project.
- This command creates a new folder named
Change to the project directory:
cd simple-landing-page
- This command moves you into the project folder.
Install the required dependencies:
npm install
- This command installs all the tools and packages needed for the React project.
Step 2: Cleaning Up the Project
Explanation:
When Vite creates a new project, it comes with some example files. To keep things simple, we will remove unnecessary files and create our own.
Instructions:
- Inside the
src
folder, delete the following files:App.css
App.jsx
index.css
- Create a new CSS file named
styles.css
inside thesrc
folder. This is where we will write our CSS styles. - Create a new file named
App.jsx
inside thesrc
folder. This is where we will write our HTML structure using JSX.
Step 3: Creating the Basic Structure (HTML in React)
Explanation:
Now, we will build the structure of our landing page using JSX, which is a special syntax used in React to write HTML-like code. We’ll create a header, a hero section, a services section, and a footer. Don’t worry if it seems complex; we will break it down step by step.
Instructions:
- Open
App.jsx
and add the following code
import React from 'react';
import './styles.css'; // Importing our CSS styles
function App() {
return (
<div className="App">
{/* Header Section */}
<header className="header">
<nav className="navbar">
<h1 className="logo">My Landing Page</h1>
<ul className="nav-links">
<li><a href="#home">Home</a></li>
<li><a href="#services">Services</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
{/* Hero Section */}
<section className="hero-section" id="home">
<h2>Welcome to Our Landing Page</h2>
<p>We offer the best services to make your life easier.</p>
<button className="cta-button">Learn More</button>
</section>
{/* Services Section */}
<section className="services-section" id="services">
<div className="service">
<h3>Service 1</h3>
<p>Details about service 1.</p>
</div>
<div className="service">
<h3>Service 2</h3>
<p>Details about service 2.</p>
</div>
<div className="service">
<h3>Service 3</h3>
<p>Details about service 3.</p>
</div>
</section>
{/* Footer Section */}
<footer className="footer">
<p>© 2024 My Landing Page. All rights reserved.</p>
</footer>
</div>
);
}
export default App;
Explanation:
- We imported
React
and ourstyles.css
file. - We used JSX syntax to create different sections of our landing page:
- Header: Contains the website’s logo and navigation links.
- Hero Section: A welcoming section with a headline, some text, and a button.
- Services Section: Displays three service boxes using simple divs.
- Footer: A simple footer with copyright information.
Step 4: Adding CSS Styles
Explanation:
Now that we have our structure, we need to style it using CSS. We will use Flexbox to align items and media queries to make the page responsive.
Instructions:
- Open
styles.css
and add the following code
/* Reset default browser styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
line-height: 1.6;
}
.App {
display: flex;
flex-direction: column;
min-height: 100vh; /* Ensures the page covers the full viewport height */
}
/* Header and Navbar */
.header {
background-color: #333;
color: #fff;
padding: 10px 20px;
}
.navbar {
display: flex;
justify-content: space-between;
align-items: center;
}
.nav-links {
list-style: none;
display: flex;
}
.nav-links li {
margin-left: 20px;
}
.nav-links a {
color: #fff;
text-decoration: none;
}
/* Hero Section */
.hero-section {
background-color: #f4f4f4;
padding: 50px 20px;
text-align: center;
}
.cta-button {
background-color: #007bff;
color: #fff;
border: none;
padding: 10px 20px;
cursor: pointer;
}
/* Services Section */
.services-section {
display: flex;
justify-content: space-around;
padding: 40px 20px;
}
.service {
background-color: #e4e4e4;
padding: 20px;
width: 30%;
text-align: center;
}
/* Footer */
.footer {
background-color: #333;
color: #fff;
text-align: center;
padding: 20px 0;
margin-top: auto;
}
/* Responsive Styles */
@media (max-width: 768px) {
.navbar {
flex-direction: column;
}
.nav-links {
flex-direction: column;
margin-top: 10px;
}
.nav-links li {
margin: 10px 0;
}
.services-section {
flex-direction: column;
}
.service {
width: 100%;
margin-bottom: 20px;
}
}
Explanation:
- General Styles: We reset margins and padding and set
box-sizing: border-box;
to make the width calculations easier. - Header: Used Flexbox to align the navigation links in a row.
- Hero Section: Centered the content using
text-align: center
. - Services Section: Used Flexbox to create three columns.
- Responsive Design: Added a media query to change the layout on screens smaller than 768px (common for tablets and mobile devices).
Step 5: Running the Application
Instructions:
- In your terminal, start the application by running
npm run dev
- Open your web browser and navigate to the URL shown in the terminal (usually
http://localhost:5173
) to see your landing page.
Classroom Recap:
What We Did:
- Created a new React project using Vite.
- Built a basic structure for a landing page using JSX in React.
- Styled the page using CSS and Flexbox.
- Implemented a responsive design using media queries.
Key Points to Remember:
- Flexbox: Helps us align items in rows and columns easily.
- Media Queries: Allow us to change styles based on screen size, making our website responsive.
- Vite: A fast way to set up and run our React projects.
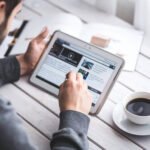
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.