In today’s fast-paced digital world, note-taking applications have become essential tools for individuals and businesses alike. They help in organizing thoughts, tasks, and important information seamlessly. This article introduces a structured approach to building a powerful backend for a note-taking application, inspired by Google’s Notes, using Express, MongoDB, and Passport. Our focus will be on a clean architecture that ensures scalability, maintainability, and ease of testing.
Why a Three-Layer Architecture?
A three-layer architecture divides an application into three distinct layers: Controllers, Services, and Data Access. This separation of concerns helps in managing complex applications by isolating business logic from routing and database interactions.
- Controllers: Handle HTTP requests and responses.
- Services: Contain business logic and processes.
- Data Access: Manage database interactions.
This structure helps prevent spaghetti code, makes the application easier to test, and enhances code readability.
Key Features of Our Notes App
To build a successful note-taking app, we need to implement several key features:
- User Authentication: Secure login and registration.
- Note Management: Create, update, copy, and delete notes dynamically.
- Simple Notes: Update and delete text in a straightforward mode.
- To-Dos: Include checkboxes to mark items complete or incomplete.
- Real-Time Updates: Push updates to clients when notes are created or deleted in different browser tabs.
- Search, Filtering, and Pagination: Efficiently retrieve and manage notes.
- Error Handling and Validation: Ensure robust input validation and error management.
Modular Approach
To achieve these features, we will divide our project into several modules, each focusing on a specific aspect of the application. Here’s a brief overview of each module:
Project Setup Module
- Initialize the project and set up dependencies.
- Configure environment variables.
- Set up a GitHub repository.
Authentication Module
- Implement user registration and login using Passport.
- Secure routes and manage user sessions.
Notes Management Module
- CRUD operations for notes.
- Real-time updates using WebSockets.
Todos Module
- Manage to-do lists within notes.
- Handle add, update, and delete operations for to-dos.
Search, Filtering, and Pagination Module
- Implement efficient search and filtering.
- Paginate results for better performance.
Error Handling and Validation Module
- Middleware for error handling.
- Validate user inputs to ensure data integrity.
Documentation and Testing Module
- Document the API using tools like Swagger.
- Write unit and integration tests.
Deployment Module
- Set up CI/CD pipelines.
- Deploy to a cloud provider like AWS or Heroku.
Conclusion
By following this modular approach, we can build a robust and scalable backend for our note-taking application. Each module is designed to be self-contained and focuses on a specific aspect of the application, ensuring that our codebase remains clean and maintainable.
In the upcoming articles, we will dive into each module, starting with the Project Setup Module. Stay tuned as we embark on this journey to build a powerful and efficient note-taking application with Express, MongoDB, and Passport.
Module 1: Project Setup
The objective of this module is to set up the foundational structure of the project. We will initialize the project, install necessary dependencies, configure environment variables, and set up a GitHub repository for version control.
Step-by-Step Guide
1. Initialize the Project
First, we need to create a new directory for our project and initialize it as a Node.js project.
mkdir notes-app
cd notes-app
npm init -y
This command creates a package.json
file with default settings.
2. Install Dependencies
Next, we need to install the necessary dependencies for our project. We will use Express for the server framework, Mongoose for interacting with MongoDB, and Passport for authentication.
npm install express mongoose passport passport-local express-session dotenv
For development, we also install Nodemon to automatically restart the server when file changes are detected.
npm install --save-dev nodemon
3. Project Structure
Create the following directory structure to organize the project:
notes-app/
├── config/
│ └── database.js
├── controllers/
│ └── authController.js
├── models/
│ └── User.js
├── services/
│ └── authService.js
├── routes/
│ └── authRoutes.js
├── middlewares/
│ └── errorHandler.js
├── .env
├── .gitignore
├── app.js
└── package.json
4. Configure Environment Variables
Create a .env
file in the root of the project to store environment variables:
PORT=3000
MONGO_URI=mongodb://localhost:27017/notesapp
SESSION_SECRET=yourSecretKey
5. Database Configuration
In the config
directory, create a database.js
file to configure the MongoDB connection:
const mongoose = require('mongoose');
const dotenv = require('dotenv');
dotenv.config();
const connectDB = async () => {
try {
await mongoose.connect(process.env.MONGO_URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
console.log('MongoDB connected');
} catch (err) {
console.error(err.message);
process.exit(1);
}
};
module.exports = connectDB;
6. App Entry Point
Create an app.js
file that serves as the entry point of the application:
const express = require('express');
const session = require('express-session');
const passport = require('passport');
const connectDB = require('./config/database');
const authRoutes = require('./routes/authRoutes');
const errorHandler = require('./middlewares/errorHandler');
const dotenv = require('dotenv');
dotenv.config();
// Connect to the database
connectDB();
const app = express();
// Middleware
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
// Express session
app.use(session({
secret: process.env.SESSION_SECRET,
resave: false,
saveUninitialized: true,
}));
// Passport middleware
app.use(passport.initialize());
app.use(passport.session());
// Routes
app.use('/api/auth', authRoutes);
// Error Handling Middleware
app.use(errorHandler);
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
7. GitHub Repository Setup
Initialize a Git repository and push the project to GitHub:
git init
git add .
git commit -m "Initial commit"
git remote add origin https://github.com/yourusername/notes-app.git
git push -u origin master
8. Nodemon Configuration
Add a start
script to package.json
for running the server with Nodemon:
"scripts": {
"start": "nodemon app.js"
}
9. Create a Basic Route
Create a simple route to verify that the server is working. In routes/authRoutes.js
:
const express = require('express');
const router = express.Router();
router.get('/test', (req, res) => {
res.send('Auth route working');
});
module.exports = router;
10. Error Handling Middleware
Create a basic error handling middleware in middlewares/errorHandler.js
:
const errorHandler = (err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Server Error');
};
module.exports = errorHandler;
Module 2: User management
The objective of this module is to implement user registration and login functionality using Passport for authentication. We will create routes, controllers, services, and data models to manage user authentication.
Step-by-Step Guide
1. User Model
First, let’s create a User model using Mongoose. This model will represent the users in our database.
In models/User.js
:
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const UserSchema = new Schema({
username: {
type: String,
required: true,
unique: true,
},
password: {
type: String,
required: true,
},
});
module.exports = mongoose.model('User', UserSchema);
Explanation:
- mongoose: We import Mongoose to interact with MongoDB.
- Schema: We define a schema for the User model.
- UserSchema: Contains
username
andpassword
fields, both required.username
is unique to ensure no duplicates. - module.exports: Exports the User model so it can be used elsewhere in the application.
2. Authentication Service
Next, we implement the business logic for user registration and login.
In services/authService.js
:
const User = require('../models/User');
const bcrypt = require('bcryptjs');
const passport = require('passport');
const LocalStrategy = require('passport-local').Strategy;
passport.use(
new LocalStrategy(async (username, password, done) => {
try {
const user = await User.findOne({ username });
if (!user) {
return done(null, false, { message: 'Incorrect username.' });
}
const isMatch = await bcrypt.compare(password, user.password);
if (!isMatch) {
return done(null, false, { message: 'Incorrect password.' });
}
return done(null, user);
} catch (err) {
return done(err);
}
})
);
passport.serializeUser((user, done) => {
done(null, user.id);
});
passport.deserializeUser(async (id, done) => {
try {
const user = await User.findById(id);
done(null, user);
} catch (err) {
done(err);
}
});
const registerUser = async (username, password) => {
const salt = await bcrypt.genSalt(10);
const hashedPassword = await bcrypt.hash(password, salt);
const newUser = new User({ username, password: hashedPassword });
await newUser.save();
return newUser;
};
module.exports = { registerUser };
Explanation:
- User: The User model imported from
models/User.js
. - bcrypt: Library for hashing passwords.
- passport: Authentication middleware for Node.js.
- LocalStrategy: Passport strategy for local authentication.
- passport.use: Defines a new LocalStrategy for Passport. It verifies if the username exists and if the password matches.
- passport.serializeUser: Serialize user to store in session.
- passport.deserializeUser: Deserialize user from session.
- registerUser: Function to register a new user. It hashes the password before saving the user to the database.
3. Authentication Controller
The controller handles HTTP requests and responses related to authentication.
In controllers/authController.js
:
const authService = require('../services/authService');
const register = async (req, res, next) => {
const { username, password } = req.body;
try {
const user = await authService.registerUser(username, password);
res.status(201).json(user);
} catch (err) {
next(err);
}
};
const login = (req, res) => {
res.status(200).json({ message: 'Logged in successfully' });
};
const logout = (req, res) => {
req.logout();
res.status(200).json({ message: 'Logged out successfully' });
};
module.exports = { register, login, logout };
Explanation:
- authService: Imported from
services/authService.js
. - register: Handles user registration. Extracts
username
andpassword
from the request body and usesauthService
to register the user. - login: Sends a success message upon successful login.
- logout: Logs out the user and sends a success message.
- module.exports: Exports the controller functions for use in routes.
4. Authentication Routes
Define routes for registration, login, and logout.
In routes/authRoutes.js
:
const express = require('express');
const passport = require('passport');
const authController = require('../controllers/authController');
const router = express.Router();
router.post('/register', authController.register);
router.post('/login', passport.authenticate('local'), authController.login);
router.get('/logout', authController.logout);
module.exports = router;
Explanation:
- express: Web framework for Node.js.
- passport: Imported to use Passport’s
authenticate
middleware. - authController: Imported from
controllers/authController.js
. - router: Express router to define routes.
- router.post(‘/register’): Route to handle user registration.
- router.post(‘/login’): Route to handle user login using Passport’s
authenticate
middleware. - router.get(‘/logout’): Route to handle user logout.
- module.exports: Exports the router for use in the main app.
5. Integrate Routes into App
Update app.js
to use the authentication routes.
In app.js
:
const express = require('express');
const session = require('express-session');
const passport = require('passport');
const connectDB = require('./config/database');
const authRoutes = require('./routes/authRoutes');
const errorHandler = require('./middlewares/errorHandler');
const dotenv = require('dotenv');
dotenv.config();
// Connect to the database
connectDB();
const app = express();
// Middleware
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
// Express session
app.use(session({
secret: process.env.SESSION_SECRET,
resave: false,
saveUninitialized: true,
}));
// Passport middleware
app.use(passport.initialize());
app.use(passport.session());
// Routes
app.use('/api/auth', authRoutes);
// Error Handling Middleware
app.use(errorHandler);
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Explanation:
- authRoutes: Imported from
routes/authRoutes.js
and used with the base path/api/auth
. - The rest of the code remains the same as in the project setup module.
Module 3: Notes Management
The objective of this module is to implement CRUD operations for notes, allowing users to create, read, update, and delete notes. We will use WebSockets for real-time updates when notes are created or deleted in different browser tabs.
Step-by-Step Guide
1. Notes Model
First, we create a Notes model using Mongoose. This model will represent the notes in our database.
In models/Note.js
:
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
// Define the Note schema
const NoteSchema = new Schema({
user: {
type: Schema.Types.ObjectId,
ref: 'User',
required: true
},
title: {
type: String,
required: true
},
content: {
type: String,
required: true
},
createdAt: {
type: Date,
default: Date.now
},
updatedAt: {
type: Date,
default: Date.now
}
});
module.exports = mongoose.model('Note', NoteSchema);
Explanation:
- mongoose: We import Mongoose to interact with MongoDB.
- Schema: We define a schema for the Note model.
- NoteSchema: Contains
user
(reference to the user who created the note),title
,content
,createdAt
, andupdatedAt
fields. - module.exports: Exports the Note model so it can be used elsewhere in the application.
2. Notes Service
Next, we implement the business logic for managing notes.
In services/noteService.js
:
const Note = require('../models/Note');
// Function to create a new note
const createNote = async (userId, title, content) => {
const newNote = new Note({
user: userId,
title,
content
});
await newNote.save();
return newNote;
};
// Function to get all notes for a specific user
const getNotes = async (userId) => {
const notes = await Note.find({ user: userId }).sort({ updatedAt: -1 });
return notes;
};
// Function to get a single note by ID
const getNoteById = async (noteId) => {
const note = await Note.findById(noteId);
return note;
};
// Function to update a note by ID
const updateNote = async (noteId, title, content) => {
const updatedNote = await Note.findByIdAndUpdate(noteId, { title, content, updatedAt: Date.now() }, { new: true });
return updatedNote;
};
// Function to delete a note by ID
const deleteNote = async (noteId) => {
await Note.findByIdAndDelete(noteId);
};
module.exports = {
createNote,
getNotes,
getNoteById,
updateNote,
deleteNote
};
Explanation:
- Note: The Note model imported from
models/Note.js
. - createNote: Creates a new note with the provided
userId
,title
, andcontent
. - getNotes: Retrieves all notes for a specific user, sorted by
updatedAt
in descending order. - getNoteById: Retrieves a single note by its ID.
- updateNote: Updates a note by its ID with new
title
andcontent
. - deleteNote: Deletes a note by its ID.
- module.exports: Exports the service functions for use in the controller.
3. Notes Controller
The controller handles HTTP requests and responses related to notes.
In controllers/noteController.js
:
const noteService = require('../services/noteService');
// Controller to handle creating a new note
const createNote = async (req, res, next) => {
const { title, content } = req.body;
const userId = req.user.id;
try {
const newNote = await noteService.createNote(userId, title, content);
res.status(201).json(newNote);
} catch (err) {
next(err);
}
};
// Controller to handle fetching all notes for the logged-in user
const getNotes = async (req, res, next) => {
const userId = req.user.id;
try {
const notes = await noteService.getNotes(userId);
res.status(200).json(notes);
} catch (err) {
next(err);
}
};
// Controller to handle fetching a single note by ID
const getNoteById = async (req, res, next) => {
const noteId = req.params.id;
try {
const note = await noteService.getNoteById(noteId);
if (!note) {
return res.status(404).json({ message: 'Note not found' });
}
res.status(200).json(note);
} catch (err) {
next(err);
}
};
// Controller to handle updating a note by ID
const updateNote = async (req, res, next) => {
const noteId = req.params.id;
const { title, content } = req.body;
try {
const updatedNote = await noteService.updateNote(noteId, title, content);
if (!updatedNote) {
return res.status(404).json({ message: 'Note not found' });
}
res.status(200).json(updatedNote);
} catch (err) {
next(err);
}
};
// Controller to handle deleting a note by ID
const deleteNote = async (req, res, next) => {
const noteId = req.params.id;
try {
await noteService.deleteNote(noteId);
res.status(200).json({ message: 'Note deleted' });
} catch (err) {
next(err);
}
};
module.exports = {
createNote,
getNotes,
getNoteById,
updateNote,
deleteNote
};
Explanation:
- noteService: Imported from
services/noteService.js
. - createNote: Extracts
title
andcontent
from the request body anduserId
from the authenticated user. UsesnoteService
to create a new note. - getNotes: Retrieves all notes for the authenticated user using
noteService
. - getNoteById: Retrieves a single note by its ID using
noteService
. Returns 404 if the note is not found. - updateNote: Updates a note by its ID with new
title
andcontent
usingnoteService
. Returns 404 if the note is not found. - deleteNote: Deletes a note by its ID using
noteService
. - module.exports: Exports the controller functions for use in routes.
4. Notes Routes
Define routes for notes management.
In routes/noteRoutes.js
:
const express = require('express');
const noteController = require('../controllers/noteController');
const { ensureAuthenticated } = require('../middlewares/authMiddleware');
const router = express.Router();
// Route to create a new note
router.post('/', ensureAuthenticated, noteController.createNote);
// Route to get all notes for the logged-in user
router.get('/', ensureAuthenticated, noteController.getNotes);
// Route to get a single note by ID
router.get('/:id', ensureAuthenticated, noteController.getNoteById);
// Route to update a note by ID
router.put('/:id', ensureAuthenticated, noteController.updateNote);
// Route to delete a note by ID
router.delete('/:id', ensureAuthenticated, noteController.deleteNote);
module.exports = router;
Explanation:
- express: Web framework for Node.js.
- noteController: Imported from
controllers/noteController.js
. - ensureAuthenticated: Middleware to ensure the user is authenticated before accessing the routes.
- router: Express router to define routes.
- router.post(‘/’): Route to handle creating a new note.
- router.get(‘/’): Route to handle fetching all notes for the logged-in user.
- router.get(‘/
- router.put(‘/
- router.delete(‘/
- module.exports: Exports the router for use in the main app.
5. Ensure Authentication Middleware
Middleware to ensure the user is authenticated before accessing certain routes.
In middlewares/authMiddleware.js
:
const ensureAuthenticated = (req, res, next) => {
if (req.isAuthenticated()) {
return next();
}
res.status(401).json({ message: 'Please log in to access this resource' });
};
module.exports = {
ensureAuthenticated
};
Explanation:
- ensureAuthenticated: Middleware function that checks if the user is authenticated using
req.isAuthenticated()
. If authenticated, it proceeds to the next middleware or route handler. If not, it responds with a 401 status and an error message. - module.exports: Exports the middleware function for use in routes.
6. Integrate Routes into App
Update app.js
to use the notes routes.
In app.js
:
const express = require('express');
const session = require('express-session');
const passport = require('passport');
const connectDB = require('./config/database');
const authRoutes = require('./routes/authRoutes');
const noteRoutes = require('./routes/noteRoutes');
const errorHandler = require('./middlewares/errorHandler');
const dotenv = require('dotenv');
dotenv.config();
// Connect to the database
connectDB();
const app = express();
// Middleware
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
// Express session
app.use(session({
secret: process.env.SESSION_SECRET,
resave: false,
saveUninitialized: true,
}));
// Passport middleware
app.use(passport.initialize());
app.use(passport.session());
// Routes
app.use('/api/auth', authRoutes);
app.use('/api/notes', noteRoutes);
// Error Handling Middleware
app.use(errorHandler);
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Explanation:
- noteRoutes: Imported from
routes/noteRoutes.js
and used with the base path/api/notes
. - The rest of the code remains the same as in the previous modules.
Module 4: Todos Management
The objective of this module is to implement the functionality for managing to-dos within notes. Users will be able to add, update, and delete to-do items within their notes.
Step-by-Step Guide
1. Todos Model
First, we create a Todos model using Mongoose. This model will represent the to-do items within a note in our database.
In models/Todo.js
:
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
// Define the Todo schema
const TodoSchema = new Schema({
note: {
type: Schema.Types.ObjectId,
ref: 'Note',
required: true
},
text: {
type: String,
required: true
},
completed: {
type: Boolean,
default: false
},
createdAt: {
type: Date,
default: Date.now
}
});
module.exports = mongoose.model('Todo', TodoSchema);
Explanation:
- mongoose: Import Mongoose to interact with MongoDB.
- Schema: Define a schema for the Todo model.
- TodoSchema: Contains
note
(reference to the note containing the to-do),text
(description of the to-do),completed
(status of the to-do), andcreatedAt
(timestamp). - module.exports: Export the Todo model for use elsewhere in the application.
2. Todos Service
Next, we implement the business logic for managing to-dos.
In services/todoService.js
:
const Todo = require('../models/Todo');
// Function to create a new to-do
const createTodo = async (noteId, text) => {
const newTodo = new Todo({
note: noteId,
text
});
await newTodo.save();
return newTodo;
};
// Function to get all to-dos for a specific note
const getTodos = async (noteId) => {
const todos = await Todo.find({ note: noteId }).sort({ createdAt: -1 });
return todos;
};
// Function to update a to-do by ID
const updateTodo = async (todoId, text, completed) => {
const updatedTodo = await Todo.findByIdAndUpdate(todoId, { text, completed }, { new: true });
return updatedTodo;
};
// Function to delete a to-do by ID
const deleteTodo = async (todoId) => {
await Todo.findByIdAndDelete(todoId);
};
module.exports = {
createTodo,
getTodos,
updateTodo,
deleteTodo
};
Explanation:
- Todo: The Todo model imported from
models/Todo.js
. - createTodo: Creates a new to-do with the provided
noteId
andtext
. - getTodos: Retrieves all to-dos for a specific note, sorted by
createdAt
in descending order. - updateTodo: Updates a to-do by its ID with new
text
andcompleted
status. - deleteTodo: Deletes a to-do by its ID.
- module.exports: Exports the service functions for use in the controller.
3. Todos Controller
The controller handles HTTP requests and responses related to to-dos.
In controllers/todoController.js
:
const todoService = require('../services/todoService');
// Controller to handle creating a new to-do
const createTodo = async (req, res, next) => {
const { noteId, text } = req.body;
try {
const newTodo = await todoService.createTodo(noteId, text);
res.status(201).json(newTodo);
} catch (err) {
next(err);
}
};
// Controller to handle fetching all to-dos for a specific note
const getTodos = async (req, res, next) => {
const noteId = req.params.noteId;
try {
const todos = await todoService.getTodos(noteId);
res.status(200).json(todos);
} catch (err) {
next(err);
}
};
// Controller to handle updating a to-do by ID
const updateTodo = async (req, res, next) => {
const todoId = req.params.id;
const { text, completed } = req.body;
try {
const updatedTodo = await todoService.updateTodo(todoId, text, completed);
if (!updatedTodo) {
return res.status(404).json({ message: 'Todo not found' });
}
res.status(200).json(updatedTodo);
} catch (err) {
next(err);
}
};
// Controller to handle deleting a to-do by ID
const deleteTodo = async (req, res, next) => {
const todoId = req.params.id;
try {
await todoService.deleteTodo(todoId);
res.status(200).json({ message: 'Todo deleted' });
} catch (err) {
next(err);
}
};
module.exports = {
createTodo,
getTodos,
updateTodo,
deleteTodo
};
Explanation:
- todoService: Imported from
services/todoService.js
. - createTodo: Extracts
noteId
andtext
from the request body and usestodoService
to create a new to-do. - getTodos: Retrieves all to-dos for a specific note using
todoService
. - updateTodo: Updates a to-do by its ID with new
text
andcompleted
status usingtodoService
. Returns 404 if the to-do is not found. - deleteTodo: Deletes a to-do by its ID using
todoService
. - module.exports: Exports the controller functions for use in routes.
4. Todos Routes
Define routes for to-dos management.
In routes/todoRoutes.js
:
const express = require('express');
const todoController = require('../controllers/todoController');
const { ensureAuthenticated } = require('../middlewares/authMiddleware');
const router = express.Router();
// Route to create a new to-do
router.post('/', ensureAuthenticated, todoController.createTodo);
// Route to get all to-dos for a specific note
router.get('/:noteId', ensureAuthenticated, todoController.getTodos);
// Route to update a to-do by ID
router.put('/:id', ensureAuthenticated, todoController.updateTodo);
// Route to delete a to-do by ID
router.delete('/:id', ensureAuthenticated, todoController.deleteTodo);
module.exports = router;
Explanation:
- express: Web framework for Node.js.
- todoController: Imported from
controllers/todoController.js
. - ensureAuthenticated: Middleware to ensure the user is authenticated before accessing the routes.
- router: Express router to define routes.
- router.post(‘/’): Route to handle creating a new to-do.
- router.get(‘/
- router.put(‘/
- router.delete(‘/
- module.exports: Exports the router for use in the main app.
5. Integrate Routes into App
Update app.js
to use the to-do routes.
In app.js
:
const express = require('express');
const session = require('express-session');
const passport = require('passport');
const connectDB = require('./config/database');
const authRoutes = require('./routes/authRoutes');
const noteRoutes = require('./routes/noteRoutes');
const todoRoutes = require('./routes/todoRoutes');
const errorHandler = require('./middlewares/errorHandler');
const dotenv = require('dotenv');
dotenv.config();
// Connect to the database
connectDB();
const app = express();
// Middleware
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
// Express session
app.use(session({
secret: process.env.SESSION_SECRET,
resave: false,
saveUninitialized: true,
}));
// Passport middleware
app.use(passport.initialize());
app.use(passport.session());
// Routes
app.use('/api/auth', authRoutes);
app.use('/api/notes', noteRoutes);
app.use('/api/todos', todoRoutes);
// Error Handling Middleware
app.use(errorHandler);
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Explanation:
- todoRoutes: Imported from
routes/todoRoutes.js
and used with the base path/api/todos
. - The rest of the code remains the same as in the previous modules.
Module 5: Search, Filtering, and Pagination
The objective of this module is to implement search, filtering, and pagination for notes. This will allow users to efficiently retrieve and manage their notes.
Step-by-Step Guide
1. Enhance Note Service for Search, Filtering, and Pagination
We need to update the note service to support search, filtering, and pagination functionalities.
In services/noteService.js
:
const Note = require('../models/Note');
// Function to create a new note
const createNote = async (userId, title, content) => {
const newNote = new Note({
user: userId,
title,
content
});
await newNote.save();
return newNote;
};
// Function to get all notes for a specific user with search, filtering, and pagination
const getNotes = async (userId, search, filter, page, limit) => {
const query = { user: userId };
// Add search condition if search term is provided
if (search) {
query.$or = [
{ title: { $regex: search, $options: 'i' } },
{ content: { $regex: search, $options: 'i' } }
];
}
// Add filter condition if filter is provided
if (filter) {
query.createdAt = { $gte: new Date(filter) };
}
// Set default values for pagination
page = page || 1;
limit = limit || 10;
const skip = (page - 1) * limit;
const notes = await Note.find(query).sort({ updatedAt: -1 }).skip(skip).limit(limit);
const total = await Note.countDocuments(query);
return { notes, total, page, pages: Math.ceil(total / limit) };
};
// Function to get a single note by ID
const getNoteById = async (noteId) => {
const note = await Note.findById(noteId);
return note;
};
// Function to update a note by ID
const updateNote = async (noteId, title, content) => {
const updatedNote = await Note.findByIdAndUpdate(noteId, { title, content, updatedAt: Date.now() }, { new: true });
return updatedNote;
};
// Function to delete a note by ID
const deleteNote = async (noteId) => {
await Note.findByIdAndDelete(noteId);
};
module.exports = {
createNote,
getNotes,
getNoteById,
updateNote,
deleteNote
};
Explanation:
- createNote: Unchanged from previous implementation.
- getNotes:
- query: Initialized to filter notes by user ID.
- search: Adds a condition to match
title
orcontent
if a search term is provided. - filter: Adds a condition to filter notes created after a specific date.
- page: Default value set to 1 if not provided.
- limit: Default value set to 10 if not provided.
- skip: Calculates the number of documents to skip based on the current page.
- notes: Retrieves notes matching the query, sorted by
updatedAt
, and applies pagination. - total: Counts the total number of documents matching the query.
- return: Returns an object containing the notes, total count, current page, and total pages.
- getNoteById: Unchanged from previous implementation.
- updateNote: Unchanged from previous implementation.
- deleteNote: Unchanged from previous implementation.
- module.exports: Exports the service functions for use in the controller.
2. Update Note Controller for Search, Filtering, and Pagination
The controller will handle HTTP requests and responses related to search, filtering, and pagination.
In controllers/noteController.js
:
const noteService = require('../services/noteService');
// Controller to handle creating a new note
const createNote = async (req, res, next) => {
const { title, content } = req.body;
const userId = req.user.id;
try {
const newNote = await noteService.createNote(userId, title, content);
res.status(201).json(newNote);
} catch (err) {
next(err);
}
};
// Controller to handle fetching all notes for the logged-in user with search, filtering, and pagination
const getNotes = async (req, res, next) => {
const userId = req.user.id;
const { search, filter, page, limit } = req.query;
try {
const result = await noteService.getNotes(userId, search, filter, parseInt(page), parseInt(limit));
res.status(200).json(result);
} catch (err) {
next(err);
}
};
// Controller to handle fetching a single note by ID
const getNoteById = async (req, res, next) => {
const noteId = req.params.id;
try {
const note = await noteService.getNoteById(noteId);
if (!note) {
return res.status(404).json({ message: 'Note not found' });
}
res.status(200).json(note);
} catch (err) {
next(err);
}
};
// Controller to handle updating a note by ID
const updateNote = async (req, res, next) => {
const noteId = req.params.id;
const { title, content } = req.body;
try {
const updatedNote = await noteService.updateNote(noteId, title, content);
if (!updatedNote) {
return res.status(404).json({ message: 'Note not found' });
}
res.status(200).json(updatedNote);
} catch (err) {
next(err);
}
};
// Controller to handle deleting a note by ID
const deleteNote = async (req, res, next) => {
const noteId = req.params.id;
try {
await noteService.deleteNote(noteId);
res.status(200).json({ message: 'Note deleted' });
} catch (err) {
next(err);
}
};
module.exports = {
createNote,
getNotes,
getNoteById,
updateNote,
deleteNote
};
Explanation:
- createNote: Unchanged from previous implementation.
- getNotes:
- userId: Extracts the authenticated user’s ID from the request.
- { search, filter, page, limit }: Extracts query parameters for search, filter, page, and limit.
- result: Calls
noteService.getNotes
with the extracted parameters and returns the result. - res.status(200).json(result): Responds with the result.
- getNoteById: Unchanged from previous implementation.
- updateNote: Unchanged from previous implementation.
- deleteNote: Unchanged from previous implementation.
- module.exports: Exports the controller functions for use in routes.
3. Update Note Routes for Search, Filtering, and Pagination
Update the routes to include search, filtering, and pagination functionalities.
In routes/noteRoutes.js
:
const express = require('express');
const noteController = require('../controllers/noteController');
const { ensureAuthenticated } = require('../middlewares/authMiddleware');
const router = express.Router();
// Route to create a new note
router.post('/', ensureAuthenticated, noteController.createNote);
// Route to get all notes for the logged-in user with search, filtering, and pagination
router.get('/', ensureAuthenticated, noteController.getNotes);
// Route to get a single note by ID
router.get('/:id', ensureAuthenticated, noteController.getNoteById);
// Route to update a note by ID
router.put('/:id', ensureAuthenticated, noteController.updateNote);
// Route to delete a note by ID
router.delete('/:id', ensureAuthenticated, noteController.deleteNote);
module.exports = router;
Explanation:
- express: Web framework for Node.js.
- noteController: Imported from
controllers/noteController.js
. - ensureAuthenticated: Middleware to ensure the user is authenticated before accessing the routes.
- router: Express router to define routes.
- router.post(‘/’): Route to handle creating a new note.
- router.get(‘/’): Route to handle fetching all notes for the logged-in user, with support for search, filtering, and pagination.
- router.get(‘/
- router.put(‘/
- router.delete(‘/
- module.exports: Exports the router for use in the main app.
4. Integrate Routes into App
Update app.js
to ensure the routes are correctly integrated.
In app.js
:
const express = require('express');
const session = require('express-session');
const passport = require('passport');
const connectDB = require('./config/database');
const authRoutes = require('./routes/authRoutes');
const noteRoutes = require('./routes/noteRoutes');
const todoRoutes = require('./routes/todoRoutes');
const errorHandler = require('./middlewares/errorHandler');
const dotenv = require('dotenv');
dotenv.config();
// Connect to the database
connectDB();
const app = express();
// Middleware
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
// Express session
app.use(session({
secret: process.env.SESSION_SECRET,
resave: false,
saveUninitialized: true,
}));
// Passport middleware
app.use(passport.initialize());
app.use(passport.session());
// Routes
app.use('/api/auth', authRoutes);
app.use('/api/notes', noteRoutes);
app.use('/api/todos', todoRoutes);
// Error Handling Middleware
app.use(errorHandler);
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Explanation:
- noteRoutes: Imported from
routes/noteRoutes.js
and used with the base path/api/notes
. - The rest of the code remains the same as in the previous modules.
Module 6: Error Handling and Validation
The objective of this module is to implement comprehensive error handling and input validation to ensure robustness and data integrity in our application.
Step-by-Step Guide
1. Error Handling Middleware
Create middleware to handle errors centrally.
In middlewares/errorHandler.js
:
// Error handling middleware
const errorHandler = (err, req, res, next) => {
console.error(err.stack); // Log the error stack trace to the console
const statusCode = err.statusCode || 500; // Default to status code 500 if not provided
res.status(statusCode).json({
message: err.message || 'Internal Server Error', // Default to a generic error message
stack: process.env.NODE_ENV === 'production' ? '🥞' : err.stack // Conditionally include stack trace based on environment
});
};
module.exports = errorHandler;
Explanation:
- errorHandler: Middleware function that captures errors in the application.
- err: The error object.
- req: The HTTP request object.
- res: The HTTP response object.
- next: The next middleware function in the stack.
- statusCode: Determines the status code for the response, defaulting to 500 if not specified.
- message: Provides an error message, defaulting to ‘Internal Server Error’ if not specified.
- stack: Conditionally includes the error stack trace based on the environment (only includes in non-production environments).
2. Input Validation Middleware
Implement input validation using express-validator
.
Install the library:
npm install express-validator
In middlewares/validators.js
:
const { body, validationResult } = require('express-validator');
// Validation rules for user registration
const validateUserRegistration = [
body('username').isLength({ min: 5 }).withMessage('Username must be at least 5 characters long'),
body('password').isLength({ min: 8 }).withMessage('Password must be at least 8 characters long')
];
// Validation rules for note creation
const validateNoteCreation = [
body('title').notEmpty().withMessage('Title is required'),
body('content').notEmpty().withMessage('Content is required')
];
// Validation rules for to-do creation
const validateTodoCreation = [
body('noteId').notEmpty().withMessage('Note ID is required'),
body('text').notEmpty().withMessage('Text is required')
];
// Middleware to check validation results and send errors if any
const validationHandler = (req, res, next) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
next();
};
module.exports = {
validateUserRegistration,
validateNoteCreation,
validateTodoCreation,
validationHandler
};
Explanation:
- body: Import from
express-validator
to validate request body fields. - validationResult: Function to collect validation errors.
- validateUserRegistration: Array of validation rules for user registration.
- username: Must be at least 5 characters long.
- password: Must be at least 8 characters long.
- validateNoteCreation: Array of validation rules for note creation.
- title: Must not be empty.
- content: Must not be empty.
- validateTodoCreation: Array of validation rules for to-do creation.
- noteId: Must not be empty.
- text: Must not be empty.
- validationHandler: Middleware to handle validation results and send error responses if validation fails.
3. Integrate Validation into Routes
Update the routes to include validation middleware.
In routes/authRoutes.js
:
const express = require('express');
const passport = require('passport');
const authController = require('../controllers/authController');
const { validateUserRegistration, validationHandler } = require('../middlewares/validators');
const router = express.Router();
// Route to register a new user
router.post('/register', validateUserRegistration, validationHandler, authController.register);
// Route to login a user
router.post('/login', passport.authenticate('local'), authController.login);
// Route to logout a user
router.get('/logout', authController.logout);
module.exports = router;
Explanation:
- validateUserRegistration: Added as middleware to validate user registration fields.
- validationHandler: Added as middleware to handle validation errors.
In routes/noteRoutes.js
:
const express = require('express');
const noteController = require('../controllers/noteController');
const { ensureAuthenticated } = require('../middlewares/authMiddleware');
const { validateNoteCreation, validationHandler } = require('../middlewares/validators');
const router = express.Router();
// Route to create a new note
router.post('/', ensureAuthenticated, validateNoteCreation, validationHandler, noteController.createNote);
// Route to get all notes for the logged-in user with search, filtering, and pagination
router.get('/', ensureAuthenticated, noteController.getNotes);
// Route to get a single note by ID
router.get('/:id', ensureAuthenticated, noteController.getNoteById);
// Route to update a note by ID
router.put('/:id', ensureAuthenticated, validateNoteCreation, validationHandler, noteController.updateNote);
// Route to delete a note by ID
router.delete('/:id', ensureAuthenticated, noteController.deleteNote);
module.exports = router;
Explanation:
- validateNoteCreation: Added as middleware to validate note creation fields.
- validationHandler: Added as middleware to handle validation errors.
In routes/todoRoutes.js
:
const express = require('express');
const todoController = require('../controllers/todoController');
const { ensureAuthenticated } = require('../middlewares/authMiddleware');
const { validateTodoCreation, validationHandler } = require('../middlewares/validators');
const router = express.Router();
// Route to create a new to-do
router.post('/', ensureAuthenticated, validateTodoCreation, validationHandler, todoController.createTodo);
// Route to get all to-dos for a specific note
router.get('/:noteId', ensureAuthenticated, todoController.getTodos);
// Route to update a to-do by ID
router.put('/:id', ensureAuthenticated, validateTodoCreation, validationHandler, todoController.updateTodo);
// Route to delete a to-do by ID
router.delete('/:id', ensureAuthenticated, todoController.deleteTodo);
module.exports = router;
Explanation:
- validateTodoCreation: Added as middleware to validate to-do creation fields.
- validationHandler: Added as middleware to handle validation errors.
4. Integrate Error Handling Middleware into App
Update app.js
to ensure the error handling middleware is used.
In app.js
:
const express = require('express');
const session = require('express-session');
const passport = require('passport');
const connectDB = require('./config/database');
const authRoutes = require('./routes/authRoutes');
const noteRoutes = require('./routes/noteRoutes');
const todoRoutes = require('./routes/todoRoutes');
const errorHandler = require('./middlewares/errorHandler');
const dotenv = require('dotenv');
dotenv.config();
// Connect to the database
connectDB();
const app = express();
// Middleware
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
// Express session
app.use(session({
secret: process.env.SESSION_SECRET,
resave: false,
saveUninitialized: true,
}));
// Passport middleware
app.use(passport.initialize());
app.use(passport.session());
// Routes
app.use('/api/auth', authRoutes);
app.use('/api/notes', noteRoutes);
app.use('/api/todos', todoRoutes);
// Error Handling Middleware
app.use(errorHandler);
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Explanation:
- errorHandler: Added at the end of the middleware stack to handle any errors that occur during request processing.
- The rest of the code remains the same as in the previous modules.
Module 7: Documentation and Testing
The objective of this module is to document the API using Swagger and write tests to ensure the functionality of the application. This will help in maintaining the quality and reliability of the application.
Step-by-Step Guide
1. API Documentation with Swagger
We will use Swagger to document our API endpoints. Swagger provides a user-friendly interface for developers to explore and interact with the API.
Install the necessary packages:
npm install swagger-jsdoc swagger-ui-express
Create a swagger.js
file in the config
directory to set up Swagger:
In config/swagger.js
:
const swaggerJsdoc = require('swagger-jsdoc');
const swaggerUi = require('swagger-ui-express');
// Swagger definition
const swaggerDefinition = {
openapi: '3.0.0',
info: {
title: 'Notes App API',
version: '1.0.0',
description: 'API documentation for the Notes App'
},
servers: [
{
url: 'http://localhost:3000',
description: 'Development server'
}
]
};
// Options for the swagger docs
const options = {
swaggerDefinition,
apis: ['./routes/*.js']
};
// Initialize swagger-jsdoc
const swaggerSpec = swaggerJsdoc(options);
module.exports = (app) => {
app.use('/api-docs', swaggerUi.serve, swaggerUi.setup(swaggerSpec));
};
Explanation:
- swaggerJsdoc: Library to generate Swagger specification from JSDoc comments.
- swaggerUi: Library to serve Swagger UI.
- swaggerDefinition: Basic information about the API (title, version, description, server URL).
- options: Configuration options for Swagger, including the path to the API route files.
- swaggerSpec: Generates the Swagger specification.
- module.exports: Function to set up Swagger UI in the application.
Add Swagger comments to the route files. For example, in routes/authRoutes.js
:
const express = require('express');
const passport = require('passport');
const authController = require('../controllers/authController');
const { validateUserRegistration, validationHandler } = require('../middlewares/validators');
const router = express.Router();
/**
* @swagger
* tags:
* name: Authentication
* description: User authentication
*/
/**
* @swagger
* /api/auth/register:
* post:
* summary: Register a new user
* tags: [Authentication]
* requestBody:
* required: true
* content:
* application/json:
* schema:
* type: object
* required:
* - username
* - password
* properties:
* username:
* type: string
* password:
* type: string
* responses:
* 201:
* description: User registered successfully
* 400:
* description: Bad request
*/
router.post('/register', validateUserRegistration, validationHandler, authController.register);
/**
* @swagger
* /api/auth/login:
* post:
* summary: Log in a user
* tags: [Authentication]
* requestBody:
* required: true
* content:
* application/json:
* schema:
* type: object
* required:
* - username
* - password
* properties:
* username:
* type: string
* password:
* type: string
* responses:
* 200:
* description: User logged in successfully
* 401:
* description: Unauthorized
*/
router.post('/login', passport.authenticate('local'), authController.login);
/**
* @swagger
* /api/auth/logout:
* get:
* summary: Log out a user
* tags: [Authentication]
* responses:
* 200:
* description: User logged out successfully
*/
router.get('/logout', authController.logout);
module.exports = router;
Explanation:
- @swagger: JSDoc comments to define API documentation.
- tags: Grouping of endpoints under a common tag (e.g., Authentication).
- /api/auth/register: Documentation for the register endpoint.
- /api/auth/login: Documentation for the login endpoint.
- /api/auth/logout: Documentation for the logout endpoint.
Repeat similar Swagger comments for other route files.
Integrate Swagger setup into app.js
:
In app.js
:
const express = require('express');
const session = require('express-session');
const passport = require('passport');
const connectDB = require('./config/database');
const authRoutes = require('./routes/authRoutes');
const noteRoutes = require('./routes/noteRoutes');
const todoRoutes = require('./routes/todoRoutes');
const errorHandler = require('./middlewares/errorHandler');
const dotenv = require('dotenv');
const setupSwagger = require('./config/swagger');
dotenv.config();
// Connect to the database
connectDB();
const app = express();
// Middleware
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
// Express session
app.use(session({
secret: process.env.SESSION_SECRET,
resave: false,
saveUninitialized: true,
}));
// Passport middleware
app.use(passport.initialize());
app.use(passport.session());
// Routes
app.use('/api/auth', authRoutes);
app.use('/api/notes', noteRoutes);
app.use('/api/todos', todoRoutes);
// Swagger setup
setupSwagger(app);
// Error Handling Middleware
app.use(errorHandler);
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Explanation:
- setupSwagger: Imported from
config/swagger
and used to set up Swagger UI in the application.
2. Testing with Jest and Supertest
Set up Jest and Supertest for testing.
Install the necessary packages:
npm install --save-dev jest supertest
Add a test script to package.json
:
"scripts": {
"test": "jest"
}
Create a test file for authentication in tests/auth.test.js
:
In tests/auth.test.js
:
const request = require('supertest');
const app = require('../app');
const mongoose = require('mongoose');
const User = require('../models/User');
beforeAll(async () => {
await mongoose.connect(process.env.MONGO_URI, {
useNewUrlParser: true,
useUnifiedTopology: true
});
});
afterAll(async () => {
await mongoose.connection.close();
});
describe('Auth API', () => {
let token;
it('should register a new user', async () => {
const res = await request(app)
.post('/api/auth/register')
.send({
username: 'testuser',
password: 'testpassword'
});
expect(res.statusCode).toEqual(201);
expect(res.body).toHaveProperty('username', 'testuser');
});
it('should login a user', async () => {
const res = await request(app)
.post('/api/auth/login')
.send({
username: 'testuser',
password: 'testpassword'
});
expect(res.statusCode).toEqual(200);
token = res.body.token;
});
it('should logout a user', async () => {
const res = await request(app)
.get('/api/auth/logout')
.set('Authorization', `Bearer ${token}`);
expect(res.statusCode).toEqual(200);
});
});
afterAll(async () => {
await User.deleteMany({});
});
Explanation:
- request: Imported from
supertest
to simulate HTTP requests. - app: The Express app imported from
app.js
. - mongoose: Imported to manage MongoDB connections.
- User: Imported to manage user data in tests.
- beforeAll: Hook to set up the database connection before running tests.
- afterAll: Hook to close the database connection and clean up after tests.
- describe: Grouping of related tests for the Auth API.
- it: Individual test cases for user registration, login, and logout.
- token: Variable to store the authentication token for subsequent requests.
Repeat similar tests for notes and todos functionalities.
Module 8: Deployment
The objective of this module is to deploy the application to a cloud provider, ensuring that it is accessible to users. We will set up deployment pipelines using CI/CD tools and configure deployment environments.
Step-by-Step Guide
1. Prepare for Deployment
Ensure that the application is ready for deployment by addressing the following:
- Environment Variables: Ensure that sensitive information is stored in environment variables.
- Production Dependencies: Verify that all necessary dependencies are listed in the
dependencies
section ofpackage.json
. - Build Scripts: Ensure that necessary build scripts are defined in
package.json
.
2. Choose a Cloud Provider
We will use Heroku for this deployment example. Heroku is a popular cloud platform that is easy to set up and use for Node.js applications.
3. Deploying to Heroku
Step 1: Create a Heroku Account
If you don’t already have a Heroku account, create one at heroku.com.
Step 2: Install the Heroku CLI
Install the Heroku Command Line Interface (CLI) to manage your applications from the terminal.
npm install -g heroku
Step 3: Log In to Heroku
Log in to your Heroku account using the CLI.
heroku login
Step 4: Create a Heroku Application
Create a new Heroku application from your project directory.
heroku create notes-app-example
Step 5: Configure Environment Variables
Set the necessary environment variables on Heroku.
heroku config:set SESSION_SECRET=yourSecretKey
heroku config:set MONGO_URI=yourMongoURI
Step 6: Deploy the Application
Deploy your application to Heroku using Git.
git add .
git commit -m "Prepare for deployment"
git push heroku master
Step 7: Open the Application
Open your deployed application in the browser.
heroku open
4. Set Up Continuous Integration/Continuous Deployment (CI/CD)
To automate the deployment process, we will set up CI/CD using GitHub Actions.
Step 1: Create a GitHub Actions Workflow
Create a directory for GitHub Actions workflows in your project:
mkdir -p .github/workflows
Create a workflow file in the .github/workflows
directory:
In .github/workflows/deploy.yml
:
name: Deploy to Heroku
on:
push:
branches:
- master
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Check out code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v1
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
- name: Deploy to Heroku
env:
HEROKU_API_KEY: ${{ secrets.HEROKU_API_KEY }}
HEROKU_APP_NAME: notes-app-example
run: |
git remote add heroku https://git.heroku.com/${{ env.HEROKU_APP_NAME }}.git
git push heroku master
Explanation:
- name: The name of the workflow.
- on: Specifies the event that triggers the workflow (
push
to themaster
branch). - jobs: Defines the jobs to be run in the workflow.
- build: The job to build and deploy the application.
- runs-on: Specifies the environment for the job (Ubuntu).
- steps: The steps to execute the job.
- Check out code: Uses the
actions/checkout
action to check out the code from the repository. - Set up Node.js: Uses the
actions/setup-node
action to set up Node.js. - Install dependencies: Installs the project dependencies using
npm install
. - Run tests: Runs the tests using
npm test
. - Deploy to Heroku: Deploys the application to Heroku using the
HEROKU_API_KEY
andHEROKU_APP_NAME
environment variables.
- Check out code: Uses the
- build: The job to build and deploy the application.
Step 2: Add Heroku API Key to GitHub Secrets
Add the Heroku API key to your GitHub repository secrets:
- Go to your GitHub repository.
- Click on
Settings
. - Click on
Secrets
in the left sidebar. - Click on
New repository secret
. - Add a secret with the name
HEROKU_API_KEY
and the value as your Heroku API key.
Step 3: Push Changes to GitHub
Push your changes to GitHub to trigger the CI/CD pipeline.
git add .github/workflows/deploy.yml
git commit -m "Set up CI/CD with GitHub Actions"
git push origin master
The objective of this final section is to summarize the development process, highlight the key aspects of the application, and provide guidance on future improvements and maintenance.
Summary of Development Process
Project Setup:
- Initialized the project with necessary dependencies and structured the project for scalability and maintainability.
- Configured environment variables and connected to a GitHub repository for version control.
Authentication Module:
- Implemented user registration and login using Passport.js.
- Created a User model and authentication service to manage user data and authentication logic.
- Secured routes with middleware to ensure authenticated access.
Notes Management Module:
- Implemented CRUD operations for notes.
- Created a Note model, service, and controller to handle business logic and data manipulation.
- Ensured real-time updates using WebSockets for an enhanced user experience.
Todos Management Module:
- Managed to-do items within notes with CRUD operations.
- Created a Todo model, service, and controller to encapsulate the to-do logic.
Search, Filtering, and Pagination Module:
- Enhanced the notes service to support search, filtering, and pagination.
- Updated the controller and routes to handle these additional functionalities.
Error Handling and Validation Module:
- Implemented comprehensive error handling middleware.
- Used
express-validator
to validate input data and ensure data integrity.
Documentation and Testing Module:
- Documented the API using Swagger for easy exploration and interaction.
- Wrote tests using Jest and Supertest to ensure the reliability of the application.
Deployment Module:
- Deployed the application to Heroku for accessibility.
- Set up CI/CD using GitHub Actions to automate the deployment process and maintain consistency.
Key Features of the Application
- Authentication: Secure user registration and login.
- Notes Management: Create, update, delete, and search notes.
- Todos Management: Manage to-do items within notes.
- Real-Time Updates: Sync notes across different sessions.
- Search and Filtering: Efficiently retrieve notes based on user criteria.
- Pagination: Handle large datasets with pagination.
- Error Handling: Robust error handling mechanisms.
- Validation: Ensure data integrity with comprehensive validation.
- API Documentation: User-friendly API documentation with Swagger.
- Testing: Automated tests to ensure functionality.
- Deployment: Seamless deployment and CI/CD setup.
Future Improvements
User Roles and Permissions:
- Implement different user roles (e.g., admin, editor, viewer) with specific permissions.
- Enhance security by restricting access based on roles.
Advanced Search:
- Add advanced search capabilities (e.g., full-text search, tag-based search).
- Optimize search queries for better performance.
File Attachments:
- Allow users to attach files to notes.
- Integrate with cloud storage services for file management.
Collaboration Features:
- Enable real-time collaboration on notes.
- Implement shared notes and collaborative editing.
Notifications:
- Add notifications for important events (e.g., due dates, reminders).
- Integrate with email or push notification services.
Performance Optimization:
- Optimize database queries and indexes for faster data retrieval.
- Implement caching strategies to reduce load on the server.
Improved UI/UX:
- Develop a frontend to enhance user interaction and experience.
- Use modern frontend frameworks (e.g., React, Angular, Vue) for a dynamic user interface.
Maintenance
- Regular Updates: Keep dependencies up to date to ensure security and performance.
- Monitoring: Use monitoring tools (e.g., New Relic, Datadog) to track application performance and errors.
- Backup: Implement regular database backups to prevent data loss.
- Security Audits: Conduct regular security audits to identify and fix vulnerabilities.
Enhancing the note-taking application with additional features and optimizations can greatly improve user experience, functionality, and scalability. Here are some detailed suggestions for future improvements:
1. User Roles and Permissions
- Roles Implementation: Define roles such as admin, editor, and viewer.
- Permissions Management: Restrict access to certain actions based on user roles.
- Admin Dashboard: Create an admin dashboard to manage users, roles, and permissions.
2. Advanced Search
- Full-Text Search: Integrate full-text search capabilities using services like Elasticsearch or MongoDB Atlas Search.
- Tag-Based Search: Allow users to add tags to notes and implement search functionality based on tags.
- Advanced Filtering: Add more complex filtering options, such as date ranges, priority levels, or custom fields.
3. File Attachments
- File Uploads: Enable users to attach files to notes.
- Cloud Storage Integration: Integrate with cloud storage services like AWS S3, Google Cloud Storage, or Azure Blob Storage for managing file attachments.
- Preview and Download: Allow users to preview and download attached files directly from the notes.
4. Collaboration Features
- Real-Time Collaboration: Enable multiple users to edit notes simultaneously with real-time updates.
- Shared Notes: Allow users to share notes with others, with options for view or edit permissions.
- Commenting: Implement a commenting system for users to leave comments on notes.
5. Notifications
- Email Notifications: Send email notifications for important events, such as due dates or updates to shared notes.
- Push Notifications: Integrate with push notification services to send alerts directly to users’ devices.
- In-App Notifications: Implement an in-app notification system to alert users of relevant events.
6. Performance Optimization
- Database Optimization: Optimize database queries and create appropriate indexes to improve performance.
- Caching: Implement caching strategies (e.g., Redis) to reduce database load and speed up response times.
- Load Balancing: Use load balancers to distribute traffic across multiple server instances.
7. Improved UI/UX
- Frontend Development: Develop a modern frontend using frameworks like React, Angular, or Vue.js.
- Responsive Design: Ensure the application is fully responsive and works well on different devices and screen sizes.
- User Experience Enhancements: Focus on improving navigation, accessibility, and overall user interaction.
8. Mobile Application
- Native Mobile Apps: Develop native mobile applications for iOS and Android to provide a seamless mobile experience.
- Cross-Platform Development: Use frameworks like React Native or Flutter to create cross-platform mobile applications.
9. Analytics and Insights
- User Activity Tracking: Implement tracking to monitor user activity and usage patterns.
- Analytics Dashboard: Create an analytics dashboard to provide insights into user behavior and application performance.
- Reporting Tools: Offer reporting tools for users to generate and export reports based on their notes and activities.
10. Security Enhancements
- Two-Factor Authentication: Implement two-factor authentication (2FA) for an additional layer of security.
- Rate Limiting: Protect against brute-force attacks by implementing rate limiting on sensitive endpoints.
- Regular Security Audits: Conduct regular security audits and penetration testing to identify and fix vulnerabilities.
11. Localization and Internationalization
- Multi-Language Support: Add support for multiple languages to cater to a global audience.
- Locale-Based Formatting: Adjust date, time, and number formats based on user locale
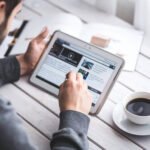
Welcome to DevTechTutor.com, your ultimate resource for mastering web development and technology! Whether you're a beginner eager to dive into coding or an experienced developer looking to sharpen your skills, DevTechTutor.com is here to guide you every step of the way. Our mission is to make learning web development accessible, engaging, and effective.